Create Games with Java: A Fun Introduction for Beginners
A guide to creating a game using Java This guide provides a beginner-friendly introduction, demonstrating how to create a simple guessing number game using Java. It introduces basic concepts and techniques step by step, including: setting up the game interface and generating random numbers getting user input checking if the guess is correct providing hints and repeating the loop until the guess is correct
Creating Games with Java: A Fun Introduction for Beginners
Introduction
Java is a powerful programming language that can be used to create a variety of applications Programs and Games. For beginners, it is a good choice to learn programming and game development. This article will guide you through creating a simple game using Java, introducing basic concepts and techniques step by step.
Practical Case: Number Guessing Game
We will create a simple number guessing game. Players need to guess a random computer-generated number, with hints provided for each guess until the player guesses correctly.
Step 1: Set up the game interface
Create a class named GuessNumberGame
which will contain the game logic.
import java.util.Scanner; public class GuessNumberGame { private static final int MAX_NUMBER = 100; private static final Scanner scanner = new Scanner(System.in); // 游戏的主方法 public static void main(String[] args) { // 1. 生成一个随机数 int randomNumber = generateRandomNumber(); // 2. 循环获取用户的猜测 while (true) { // 提示用户输入猜测 System.out.print("请输入您的猜测(1-100):"); int guess = scanner.nextInt(); // 3. 检查猜测是否正确 if (guess == randomNumber) { System.out.println("恭喜!您猜对了!"); break; } else if (guess < randomNumber) { System.out.println("您的猜测太小了,请再次尝试。"); } else { System.out.println("您的猜测太大了,请再次尝试。"); } } } // 生成一个指定范围内的随机数 private static int generateRandomNumber() { return (int) (Math.random() * MAX_NUMBER) + 1; } }
Step 2: Get user input
Use the Scanner
class to get input from the user.
int guess = scanner.nextInt();
Step 3: Check Guesses
Compare the player’s guess to a random number. If the guess is correct, print a message and exit the loop. If the guess is wrong, a hint is provided based on whether the guess is greater or less than the random number.
if (guess == randomNumber) { System.out.println("恭喜!您猜对了!"); break; } else if (guess < randomNumber) { System.out.println("您的猜测太小了,请再次尝试。"); } else { System.out.println("您的猜测太大了,请再次尝试。"); }
Conclusion
Through this simple example, you have understood the following basic concepts of creating games in Java:
- Generate random numbers
- Get user input
- Check guesses using conditional statements
- Create and run a class in Java
The above is the detailed content of Create Games with Java: A Fun Introduction for Beginners. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
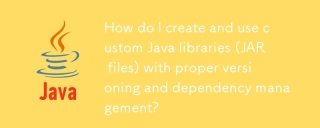
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
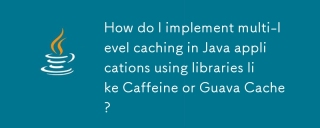
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
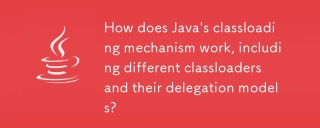
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
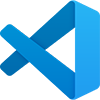
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
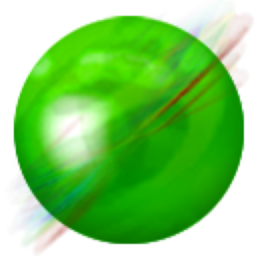
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
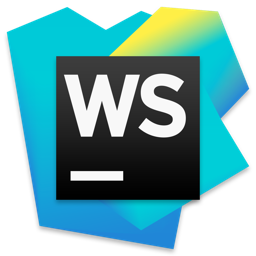
WebStorm Mac version
Useful JavaScript development tools