Welcome to the World of Programming: Your Java Journey Begins
Java is a general-purpose, object-oriented, class-based programming language known for its robustness, reliability, and platform independence. This tutorial introduces the basics of Java, including syntax and a practical case - calculating pi using Monte Carlo simulation. First set the random seed, then simulate the throwing point, calculate the distance to the center of the circle and determine whether the point is within the circle. Finally, calculate pi and output the result.
Welcome to the world of programming: start your Java journey
Introduction
Today, we start the exciting journey of Java programming. Java is a general-purpose, object-oriented, class-based programming language known for its robustness, reliability, and platform independence. In this tutorial, we'll walk through the basics of Java step by step and explore a practical case to let you experience the power of programming first-hand.
Java Basics
// Java 基础语法 class MyClass { public static void main(String[] args) { System.out.println("欢迎来到 Java 世界!"); } }
Practical Case: Calculating Pi
Let us write a Java program to calculate pi . We will use Monte Carlo simulation to estimate the ratio of π areas to approximate π.
import java.util.Random; public class PiCalculator { public static void main(String[] args) { // 设置随机种子 Random random = new Random(); // 模拟次数 int numPoints = 100000; // 圆心坐标 double centerX = 0; double centerY = 0; // 半径 double radius = 1; // 点在圆内的计数 int countInCircle = 0; // 模拟投掷点 for (int i = 0; i < numPoints; i++) { // 生成随机点 double x = random.nextDouble() * 2 * radius - radius; double y = random.nextDouble() * 2 * radius - radius; // 计算到圆心的距离 double distance = Math.sqrt(x * x + y * y); // 判断点是否在圆内 if (distance <= radius) { countInCircle++; } } // 计算圆周率π double pi = 4.0 * countInCircle / numPoints; // 输出结果 System.out.println("近似值 π = " + pi); } }
Save this code as a PiCalculator.java
file and compile it using the command javac PiCalculator.java
. Then, run the program via java PiCalculator
. You will see an estimate of π printed on the console.
Congratulations on taking the first step in your Java programming journey! Keep learning and explore more features of Java.
The above is the detailed content of Welcome to the World of Programming: Your Java Journey Begins. For more information, please follow other related articles on the PHP Chinese website!
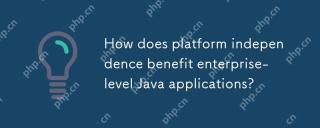
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
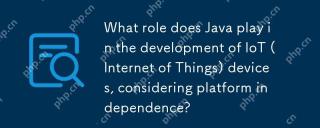
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
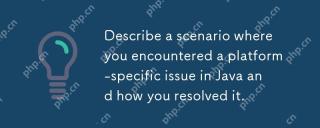
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
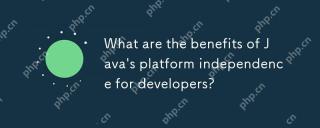
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
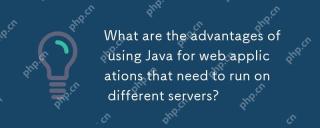
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
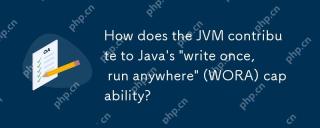
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
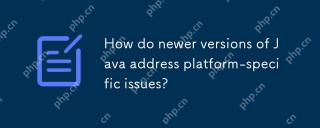
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
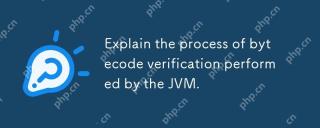
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
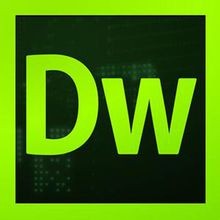
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
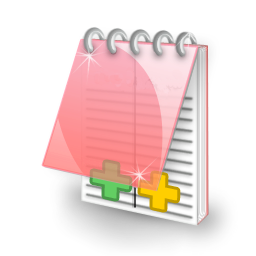
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
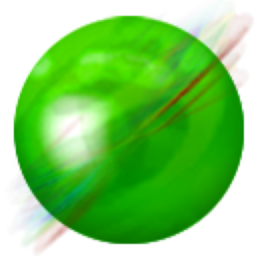
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
