What is an Atomic in Java? Understanding Atomicity and Thread Safety in Java
1. Introduction to Atomic in Java
1.1 What is an Atomic in Java?
In Java, the java.util.concurrent.atomic package offers a set of classes that support lock-free thread-safe programming on single variables. These classes are collectively referred to as atomic variables. The most commonly used atomic classes include AtomicInteger , AtomicLong , AtomicBoolean , and AtomicReference.
Atomic variables are designed to be updated atomically, meaning that their operations (such as incrementing, decrementing, or comparing and setting values) are performed as a single, indivisible step. This ensures that no other thread can observe the variable in an intermediate state.
Example: Using AtomicInteger
import java.util.concurrent.atomic.AtomicInteger; public class AtomicExample { private AtomicInteger counter = new AtomicInteger(0); public void incrementCounter() { counter.incrementAndGet(); } public int getCounter() { return counter.get(); } public static void main(String[] args) { AtomicExample example = new AtomicExample(); for (int i = 0; i <p>In this example, <strong>AtomicInteger</strong> is used to maintain a counter that can be safely incremented by multiple threads without causing inconsistencies. </p> <h3> 1.2 Atomicity and Thread Safety </h3> <p>The term "atomicity" refers to operations that are completed in a single step without the possibility of interference from other operations. In the context of multithreading, this means that a variable update occurs as an all-or-nothing operation. With regular primitive types, operations like increment <strong>(i++)</strong> are not atomic, meaning that if multiple threads try to update the same variable simultaneously, data corruption can occur. </p> <p><strong>Example: Non-Atomic Operation with Primitive Types</strong><br> </p> <pre class="brush:php;toolbar:false">public class NonAtomicExample { private int counter = 0; public synchronized void incrementCounter() { counter++; } public int getCounter() { return counter; } public static void main(String[] args) { NonAtomicExample example = new NonAtomicExample(); for (int i = 0; i <p>Even though synchronization is applied, this approach can lead to performance bottlenecks due to thread contention. Atomic classes, however, avoid this by using low-level CPU instructions to ensure atomicity without locking. </p> <h2> 2. Differences Between Atomics and Regular Primitives </h2> <p>Now that we understand what atomic variables are and how they function, let’s explore how they differ from regular primitive types in terms of atomicity and thread safety. </p> <h3> 2.1 Atomicity in Regular Primitives vs. Atomics </h3> <p>Regular primitives like <strong>int</strong> , <strong>long</strong> , <strong>boolean</strong> , etc., are not atomic by nature. Operations on these variables, such as incrementing or setting a value, can be interrupted by other threads, leading to inconsistent or corrupt data. In contrast, atomic variables ensure that these operations are performed as a single, uninterruptible step. </p> <p><strong>Example: Race Condition with Primitive Types</strong><br> </p> <pre class="brush:php;toolbar:false">public class RaceConditionExample { private int counter = 0; public void incrementCounter() { counter++; } public static void main(String[] args) { RaceConditionExample example = new RaceConditionExample(); for (int i = 0; i <p>In this example, the final counter value may not be 1000 due to race conditions. Multiple threads can access and modify the counter simultaneously, leading to unpredictable results. </p> <h3> 2.2 Thread Safety in Regular Primitives vs. Atomics </h3> <p>Thread safety is a key consideration in concurrent programming. Regular primitives require explicit synchronization to be thread-safe, which can be cumbersome and error-prone. Atomics, however, are inherently thread-safe, as they provide built-in atomic operations. </p> <p><strong>Performance Considerations</strong></p> <p>Using synchronization with regular primitives can lead to performance bottlenecks due to the overhead of acquiring and releasing locks. On the other hand, atomic classes provide a more efficient solution by using non-blocking algorithms to achieve thread safety without locks. </p> <h2> 3. Conclusion </h2> <p>Atomic variables in Java provide a powerful and efficient way to handle concurrency and ensure data consistency. They differ significantly from regular primitive types in terms of atomicity and thread safety, offering a more performant solution in multi-threaded environments. </p> <p>By understanding the concept of atomics, you can write safer and more efficient concurrent code in Java. If you have any questions or need further clarification, feel free to leave a comment below! </p> <p><strong>Read posts more at</strong> : What is an Atomic in Java? Understanding Atomicity and Thread Safety in Java</p>
The above is the detailed content of What is an Atomic in Java? Understanding Atomicity and Thread Safety in Java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
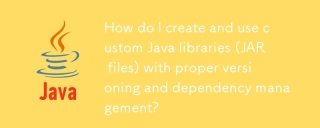
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
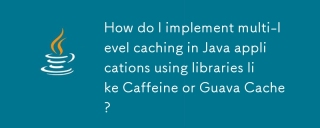
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
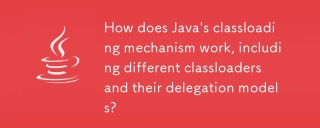
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
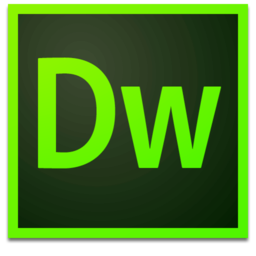
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
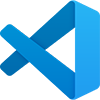
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft