Reverse is used to reverse a string or a number from back to front like for string educba the reverse string is abcude. For number 9436 reverse is 6349. With the help of a reverse string, we can reverse any string. We can implement this method in many ways. Every method has its log(n). Some methods are faster than the other method.
Some methods use more lines of code and are complex in nature which are sometimes hard to understand. We can also reverse the number.
Methods
Here are few methods by using them we can reverse the string:
- Using ReverseString method.
- Using ToCharArray method.
- Programmatically Approach: By using this approach first we will store the string in any variable and then calculate the length of that variable or string. Then we pick one character at one time and can print from starting from the backside or reverse side. Before using this method, we should ensure that this approach should not affect the performance of the program.
So we can use any of the above approaches depending upon the requirements.
Examples of Reverse String in C#
Given below are the examples of Reverse String in C#:
Example #1
Code:
using System ; using System.Collections.Generic ; using System.Linq ; using System.Text ; using System.Threading.Tasks ; namespace ReverseString { class Program { static void Main(string[] args) { string Str, rev; Str = "Programming" ; rev = ""; Console.WriteLine("Value of given String is: {0}", Str) ; // find string length int a; a = Str.Length - 1 ; while (a >= 0) { rev = rev + Str[a] ; a--; } Console.WriteLine("Reversed string is: {0}", rev) ; Console.ReadLine() ; } } }
In the above program, Str is used as a variable to store the value of a string. While loop is used to reverse the string and the logic behind is to change the position of characters one by one from right to left.
Output:
Example #2
Code:
using System ; using System.Collections.Generic; using System.Linq ; using System.Text ; using System.Threading.Tasks ; static class StringHelper { public static string ReverseString(string str) { char[] array = str.ToCharArray() ; Array.Reverse(array) ; return new string(array) ; } } class Program { static void Main() { Console.WriteLine(StringHelper.ReverseString("This")) ; Console.WriteLine(StringHelper.ReverseString("is")) ; Console.WriteLine(StringHelper.ReverseString("C#")) ; Console.WriteLine(StringHelper.ReverseString("programming")) ; Console.ReadLine(); } }
In the above example, the ReverseString method is used to get the value of string which needs to be reversed Array. The reverse is used for the modification of the order of the characters.
Output:
Example #3
Code:
using System; using System.Collections.Generic ; using System.Linq ; using System.Text ; using System.Threading.Tasks ; namespace reverseString { class Program { static void Main(string[] args) { string s = "", rev = "" ; int len = 0 ; Console.WriteLine("Enter a string") ; s = Console.ReadLine(); len = s.Length - 1; while (len >= 0) { rev = rev + s[len] ; len--; } Console.WriteLine("Reverse of string is {0}", rev) ; Console.ReadLine(); } } }
In the above program, we are taking input from the user to reverse the string. A variable is used to store the value of a string. While loop is used to reverse the string and the logic behind is to change the position of characters one by one from right to left.
Output:
Example #4
Code:
using System; using System.Collections.Generic ; using System.Linq ; using System.Text ; using System.Threading.Tasks ; namespace reverseString { class Program { static void Main(string[] args) { string s; Console.Write(" Enter the string : ") ; s = Console.ReadLine(); s = StringReverse(s); Console.Write(" Reverse is : ") ; Console.Write(s) ; Console.ReadLine() ; } public static string StringReverse(string s) { if (s.Length > 0) return s[s.Length - 1] + StringReverse(s.Substring(0, s.Length - 1)) ; else return s ; } } }
Output:
Example #5
Code:
using System ; using System.Collections.Generic ; using System.Linq ; using System.Text ; using System.Threading.Tasks ; namespace reverseString { class Program { public static void Main() { Console.WriteLine(reverse("Hello")) ; Console.ReadLine(); } public static string reverse(string s) { string reverseStr = "" ; for (int a = s.Length - 1; a >= 0; a--) { reverseStr = reverseStr + s[a] ; } return reverseStr ; } } }
In the above example, for loop is used to reverse the given string.
Output:
Example #6
Code:
using System ; using System.Collections.Generic ; using System.Linq ; using System.Text ; using System.Threading.Tasks ; namespace reverseString { class Program { public static void Main() { string s; Console.WriteLine("The value of given string is ") ; s = Console.ReadLine() ; char[] array = s.ToCharArray() ; Array.Reverse(array); Console.WriteLine(new String(array)) ; Console.ReadLine() ; } } }
Output:
Conclusion
So we can reverse any given string or number by using the number of approaches. But we should ensure that our choice should not affect the performance because each method has its own time complexity.
The above is the detailed content of Reverse String in C#. For more information, please follow other related articles on the PHP Chinese website!
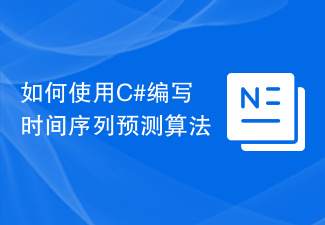
如何使用C#编写时间序列预测算法时间序列预测是一种通过分析过去的数据来预测未来数据趋势的方法。它在很多领域,如金融、销售和天气预报中有广泛的应用。在本文中,我们将介绍如何使用C#编写时间序列预测算法,并附上具体的代码示例。数据准备在进行时间序列预测之前,首先需要准备好数据。一般来说,时间序列数据应该具有足够的长度,并且是按照时间顺序排列的。你可以从数据库或者
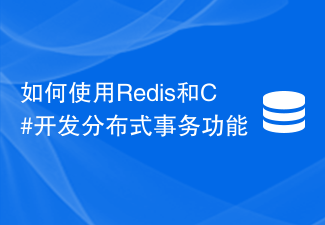
如何使用Redis和C#开发分布式事务功能引言分布式系统的开发中,事务处理是一项非常重要的功能。事务处理能够保证在分布式系统中的一系列操作要么全部成功,要么全部回滚。Redis是一种高性能的键值存储数据库,而C#是一种广泛应用于开发分布式系统的编程语言。本文将介绍如何使用Redis和C#来实现分布式事务功能,并提供具体代码示例。I.Redis事务Redis
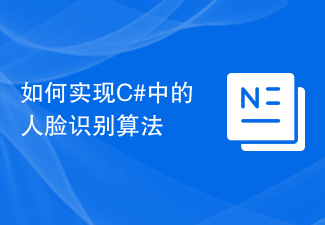
如何实现C#中的人脸识别算法人脸识别算法是计算机视觉领域中的一个重要研究方向,它可以用于识别和验证人脸,广泛应用于安全监控、人脸支付、人脸解锁等领域。在本文中,我们将介绍如何使用C#来实现人脸识别算法,并提供具体的代码示例。实现人脸识别算法的第一步是获取图像数据。在C#中,我们可以使用EmguCV库(OpenCV的C#封装)来处理图像。首先,我们需要在项目
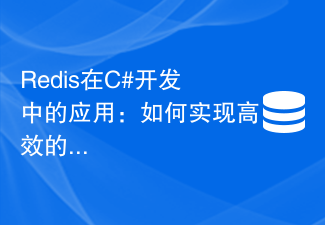
Redis在C#开发中的应用:如何实现高效的缓存更新引言:在Web开发中,缓存是提高系统性能的常用手段之一。而Redis作为一款高性能的Key-Value存储系统,能够提供快速的缓存操作,为我们的应用带来了不少便利。本文将介绍如何在C#开发中使用Redis,实现高效的缓存更新。Redis的安装与配置在开始之前,我们需要先安装Redis并进行相应的配置。你可以
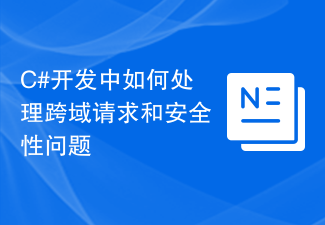
C#开发中如何处理跨域请求和安全性问题在现代的网络应用开发中,跨域请求和安全性问题是开发人员经常面临的挑战。为了提供更好的用户体验和功能,应用程序经常需要与其他域或服务器进行交互。然而,浏览器的同源策略导致了这些跨域请求被阻止,因此需要采取一些措施来处理跨域请求。同时,为了保证数据的安全性,开发人员还需要考虑一些安全性问题。本文将探讨C#开发中如何处理跨域请
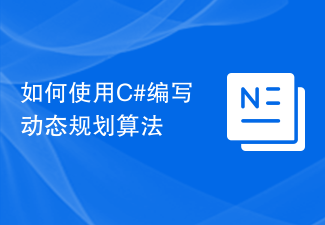
如何使用C#编写动态规划算法摘要:动态规划是求解最优化问题的一种常用算法,适用于多种场景。本文将介绍如何使用C#编写动态规划算法,并提供具体的代码示例。一、什么是动态规划算法动态规划(DynamicProgramming,简称DP)是一种用来求解具有重叠子问题和最优子结构性质的问题的算法思想。动态规划将问题分解成若干个子问题来求解,通过记录每个子问题的解,
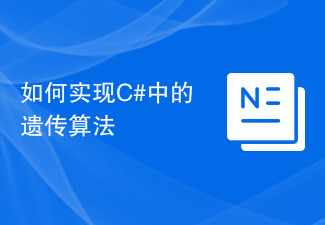
如何在C#中实现遗传算法引言:遗传算法是一种模拟自然选择和基因遗传机制的优化算法,其主要思想是通过模拟生物进化的过程来搜索最优解。在计算机科学领域,遗传算法被广泛应用于优化问题的解决,例如机器学习、参数优化、组合优化等。本文将介绍如何在C#中实现遗传算法,并提供具体的代码示例。一、遗传算法的基本原理遗传算法通过使用编码表示解空间中的候选解,并利用选择、交叉和
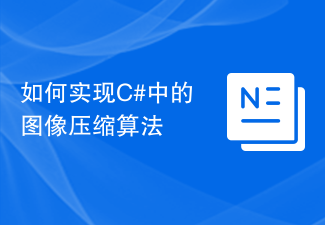
如何实现C#中的图像压缩算法摘要:图像压缩是图像处理领域中的一个重要研究方向,本文将介绍在C#中实现图像压缩的算法,并给出相应的代码示例。引言:随着数字图像的广泛应用,图像压缩成为了图像处理中的重要环节。压缩能够减小存储空间和传输带宽,并能提高图像处理的效率。在C#语言中,我们可以通过使用各种图像压缩算法来实现对图像的压缩。本文将介绍两种常见的图像压缩算法:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
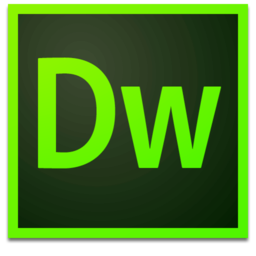
Dreamweaver Mac version
Visual web development tools