Before discussing how to create a JSON object in C#, let us first understand what JSON is. JSON stands for JavaScript Object Notation. It is a very lightweight text format which is used to exchange data. JSON can be expressed in three styles i.e. object, array, and string. Here, we will discuss the JSON object. A JSON object begins with “{” and ends with “}”. Data in JSON object is stored as key-value pair where a key and its value are separated by a colon “:” and each key-value pair is separated by a comma “,”.
Syntax:
The syntax to create JSON using Newtonsoft package is as follows:
ClassName objectName = new ClassName(); string jsonStr = JsonConvert.SerializeObject(objectName);
Explanation: In the above syntax, first we created the object of the class for which we need data in JSON format then we used JsonConvert.Serialize() method of Newtonsoft package and passed our class object as a parameter to this method which then returns a JSON string after converting the data of the object to JSON string.
After this, we can store this JSON data to a file using the below statements:
using(var streamWriter = new StreamWriter(filePath, true)) { streamWriter.WriteLine(jsonStr.ToString()); streamWriter.Close(); }
In the above statements, we created an object of StreamWriter to write JSON data to a file specified by location ‘filePath’. Then, with the help of this object we have written JSON data to the file using the WriteLine() method.
How we can Create JSON Object in C#?
In C#, we can create JSON objects in many ways i.e. by using a .NET native library or by using third party packages.
If we want to use the native .NET library to create a JSON object then we need to add System. ServiceModel.Web as a reference to our project after this we will be able to import System.Runtime.Serialization.Json namespace in our code which contains a class called DataContractJsonSerializer which is responsible to serialize the objects to the JSON data and deserialize the JSON data to objects.
Apart from this, we can use third party packages to work with JSON. Like Newtonsoft.Json package. To install this package and to add it to our project, we need to follow below steps in visual studio:
- Go to Tools > NuGet Package Manager > Manage NuGet Packages for Solution.
- Under the ‘Browse’ tab search for “Newtonsoft.Json” package and select it from the results displayed then select your project to which you want to add this package.
- Click on the install button.
After following these steps when we will check the references of our project then we will get “Newtonsoft.Json” added to it.
We can now import Newtonsoft.Json namespace in our code which contains a class called JsonConvert which provides methods for conversion between .NET types and JSON types.
Examples of C# Create JSON Object
Example showing the creation of JSON object using .NET native library.
Example #1
Code:
using System; using System.IO; using System.Collections.Generic; using System.Linq; using System.Web; using System.Runtime.Serialization.Json; using System.Runtime.Serialization; namespace ConsoleApp4 { [DataContractAttribute] public class Student { [DataMemberAttribute] public int RollNo { get; set; } [DataMemberAttribute] public string FirstName { get; set; } [DataMemberAttribute] public string LastName { get; set; } [DataMemberAttribute] public string Address { get; set; } [DataMemberAttribute] public float TotalMarks { get; set; } public Student(int RollNo, string FirstName, string LastName, string Address, float TotalMarks) { this.RollNo = RollNo; this.FirstName = FirstName; this.LastName = LastName; this.Address = Address; this.TotalMarks = TotalMarks; } } public class Program { public static void Main(string[] args) { string jsonStr; Student student = new Student(1, "Gaurang", "Pandya", "Thane, Mumbai", 800); try { MemoryStream memoryStream = new MemoryStream(); //serializing object to JSON DataContractJsonSerializer ser = new DataContractJsonSerializer(student.GetType()); //writing JSON ser.WriteObject(memoryStream, student); memoryStream.Position = 0; StreamReader streamReader = new StreamReader(memoryStream); jsonStr = streamReader.ReadToEnd(); Console.WriteLine(jsonStr); } catch(Exception ex) { Console.WriteLine(ex.Message); } Console.ReadLine(); } } }
Output:
Example #2
Example showing the creation of JSON object using .NET native library and then writing the resulted JSON data to a file using StreamWriter.
Code:
using System; using System.IO; using System.Collections.Generic; using System.Linq; using System.Web; using System.Runtime.Serialization.Json; using System.Runtime.Serialization; namespace ConsoleApp4 { [DataContractAttribute] public class Student { [DataMemberAttribute] public int RollNo; [DataMemberAttribute] public string FirstName; [DataMemberAttribute] public string LastName; [DataMemberAttribute] public string Address; [DataMemberAttribute] public float TotalMarks; public Student(int RollNo, string FirstName, string LastName, string Address, float TotalMarks) { this.RollNo = RollNo; this.FirstName = FirstName; this.LastName = LastName; this.Address = Address; this.TotalMarks = TotalMarks; } } public class Program { public static void Main(string[] args) { string jsonStr; string filePath = @"E:\Content\student.json"; Student student = new Student(1, "Gaurang", "Pandya", "Thane, Mumbai", 800); try { MemoryStream memoryStream = new MemoryStream(); //serializing object to JSON DataContractJsonSerializer ser = new DataContractJsonSerializer(student.GetType()); //writing JSON ser.WriteObject(memoryStream, student); memoryStream.Position = 0; StreamReader streamReader = new StreamReader(memoryStream); jsonStr = streamReader.ReadToEnd(); //checking if the file already exists if (File.Exists(filePath)) { //deleting file if it exists File.Delete(filePath); } //creating StreamWriter to write JSON data to file using (StreamWriter streamWriter = new StreamWriter(filePath, true)) { streamWriter.WriteLine(jsonStr.ToString()); streamWriter.Close(); } } catch (Exception ex) { Console.WriteLine(ex.Message); } Console.ReadLine(); } } }
Please find below the screenshot of the output from the above program in student.json file opened in notepad.
Output:
Example #3
Example showing the creation of JSON object using Newtonsoft package.
Code:
using System; using Newtonsoft.Json; namespace ConsoleApp4 { public class Student { public int RollNo; public string FirstName; public string LastName; public string Address; public float TotalMarks; public Student(int RollNo, string FirstName, string LastName, string Address, float TotalMarks) { this.RollNo = RollNo; this.FirstName = FirstName; this.LastName = LastName; this.Address = Address; this.TotalMarks = TotalMarks; } } public class Program { public static void Main(string[] args) { string jsonStr; Student student = new Student(1, "Gaurang", "Pandya", "Thane, Mumbai", 800); try { //serializing student object to JSON string jsonStr = JsonConvert.SerializeObject(student); Console.WriteLine(jsonStr); } catch(Exception ex) { Console.WriteLine(ex.Message); } Console.ReadLine(); } } }
Output:
Conclusion
JSON objects are enclosed under curly braces and contain key-value pairs. A key and its value are separated by colon where the key must be string and value can be of any valid data type. Each key-value pair in the JSON object is separated by a comma.
The above is the detailed content of C# Create JSON Object. For more information, please follow other related articles on the PHP Chinese website!
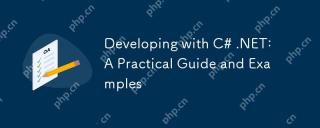
C# and .NET provide powerful features and an efficient development environment. 1) C# is a modern, object-oriented programming language that combines the power of C and the simplicity of Java. 2) The .NET framework is a platform for building and running applications, supporting multiple programming languages. 3) Classes and objects in C# are the core of object-oriented programming. Classes define data and behaviors, and objects are instances of classes. 4) The garbage collection mechanism of .NET automatically manages memory to simplify the work of developers. 5) C# and .NET provide powerful file operation functions, supporting synchronous and asynchronous programming. 6) Common errors can be solved through debugger, logging and exception handling. 7) Performance optimization and best practices include using StringBuild
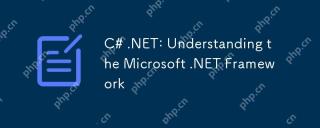
.NETFramework is a cross-language, cross-platform development platform that provides a consistent programming model and a powerful runtime environment. 1) It consists of CLR and FCL, which manages memory and threads, and FCL provides pre-built functions. 2) Examples of usage include reading files and LINQ queries. 3) Common errors involve unhandled exceptions and memory leaks, and need to be resolved using debugging tools. 4) Performance optimization can be achieved through asynchronous programming and caching, and maintaining code readability and maintainability is the key.
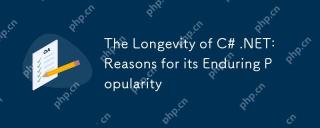
Reasons for C#.NET to remain lasting attractive include its excellent performance, rich ecosystem, strong community support and cross-platform development capabilities. 1) Excellent performance and is suitable for enterprise-level application and game development; 2) The .NET framework provides a wide range of class libraries and tools to support a variety of development fields; 3) It has an active developer community and rich learning resources; 4) .NETCore realizes cross-platform development and expands application scenarios.
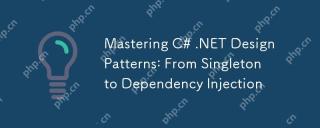
Design patterns in C#.NET include Singleton patterns and dependency injection. 1.Singleton mode ensures that there is only one instance of the class, which is suitable for scenarios where global access points are required, but attention should be paid to thread safety and abuse issues. 2. Dependency injection improves code flexibility and testability by injecting dependencies. It is often used for constructor injection, but it is necessary to avoid excessive use to increase complexity.
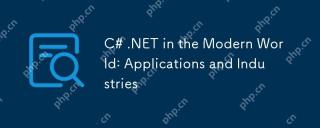
C#.NET is widely used in the modern world in the fields of game development, financial services, the Internet of Things and cloud computing. 1) In game development, use C# to program through the Unity engine. 2) In the field of financial services, C#.NET is used to develop high-performance trading systems and data analysis tools. 3) In terms of IoT and cloud computing, C#.NET provides support through Azure services to develop device control logic and data processing.
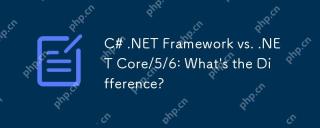
.NETFrameworkisWindows-centric,while.NETCore/5/6supportscross-platformdevelopment.1).NETFramework,since2002,isidealforWindowsapplicationsbutlimitedincross-platformcapabilities.2).NETCore,from2016,anditsevolutions(.NET5/6)offerbetterperformance,cross-
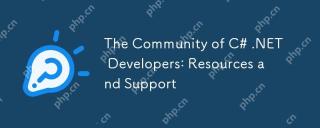
The C#.NET developer community provides rich resources and support, including: 1. Microsoft's official documents, 2. Community forums such as StackOverflow and Reddit, and 3. Open source projects on GitHub. These resources help developers improve their programming skills from basic learning to advanced applications.
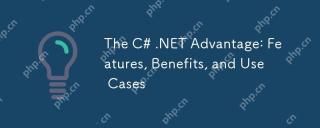
The advantages of C#.NET include: 1) Language features, such as asynchronous programming simplifies development; 2) Performance and reliability, improving efficiency through JIT compilation and garbage collection mechanisms; 3) Cross-platform support, .NETCore expands application scenarios; 4) A wide range of practical applications, with outstanding performance from the Web to desktop and game development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
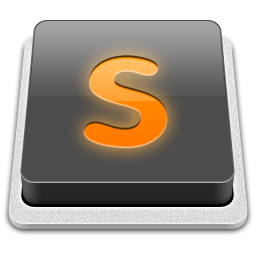
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
