Params are a very important keyword in the C#. We used param when we wanted to give the number of arguments as the variable. So it is used when the developer does not know the number of parameters that will be used. After the C# Params keyword, no additional param will be allowed in the function. If we do not pass any arguments, then the length of params will remain zero. We can send comma-separated values or arrays.
Keyword: params
using System; namespace Examples { class Test { // function containing params parameters public static int Addittion(params int[] ListNumbers) { int total = 0; // foreach loop foreach(int i in ListNumbers) { total += i; } return total; } // Driver Code static void Main(string[] args) { // Calling function by passing 5 // arguments as follows int y = Addittion (12,13,10,15,56); // Displaying result Console.WriteLine(y); } } }
Output:
With params Keyword:
static public int add(params int[] args) { int add1 = 0; foreach (var item in args) add1= add1+item + 2; return add1; }
Without params Keyword:
static public int add(int[] args) { int add = 0; foreach (var item in args) add1 = add1+item + 2; return add1; }
With param, we can call the method like add(1,4,5), but without param, we cant.
Examples of C# Params
The following examples show how we can implement params in C#.
Example #1
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Params { class Demo { public void Show(params int[] num) // Params Paramater { for (int a = 0; a <p>In the above example, a param keyword is used, allowing any type and number of types. Then, after creating the object, we pass several arguments to display.</p> <p><strong>Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534794463969.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="C# Params" ></p> <h4 id="Example">Example #2</h4> <pre class="brush:php;toolbar:false">using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Params { class Demo { public void Show(params object[] val) // Params Paramater { for (int a = 0; a <p>In the above example, a param keyword is used, allowing any type and number of types. After creating an object, we pass several arguments to display. Here you can see the arguments of the different data types.</p> <p><strong>Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534794513546.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="C# Params" ></p> <h4 id="Example">Example #3</h4> <pre class="brush:php;toolbar:false">using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Params { class Demo { public static int Addition(params int[] num) // params parameter { int add = 0; // foreach loop foreach (int a in num) { add += a; } return add; } static void Main(string[] args) { int x = Addition(23, 45, 2, 36, 76); // call function // Displaying result Console.WriteLine(x); Console.ReadLine(); } } }
In the above example, an array is used, and there is no need to mention the size of an array as a param keyword is used, which will allow any type and number of the argument. The numbers will be in the following format.
[0] 25[1] 45
[2] 2
[3] 36
[4] 76
Output:
Example #4
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Params { class Demo { static void Main() { // Call params method with five integer type arguments int cal1 = calParameters(7); int cal2 = calParameters(11, 23); int cal3 = calParameters(46, 8, 45); int cal4 = calParameters(23, 31, 21, 45); int cal5 = calParameters(12, 12, 54, 76); // display result of each calculations Console.WriteLine(cal1); Console.WriteLine(cal2); Console.WriteLine(cal3); Console.WriteLine(cal4); Console.WriteLine(cal5); Console.ReadLine(); } static int calParameters(params int[] values) { int sum = 0; foreach (int value in values) // foreach loop and sum of integers { sum += value; } return sum; } } }
There are five arguments for which a param keyword is used in the above example. All the arguments are of integer type. A foreach loop is used to display the sum of each argument.
Output:
How is it Different From an Array?
public void test(params int[] args) { } test(); // no compile-time error, args will be empty
But if You Use an Array:
public void test(int[] args) { } test(); // error: No overload for 'Foo' takes 0 arguments
So, If we do not pass any arguments, then the length of params will remain zero. Param keyword must be the last in the parameter list; otherwise, it will give an error.
Example:
public void test(params int[] args,int value) { }
This declaration is not allowed.
The above is the detailed content of C# Params. For more information, please follow other related articles on the PHP Chinese website!
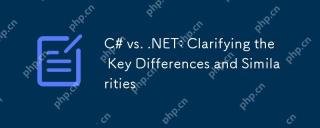
C# is a programming language, while .NET is a software framework. 1.C# is developed by Microsoft and is suitable for multi-platform development. 2..NET provides class libraries and runtime environments, and supports multilingual. The two work together to build modern applications.
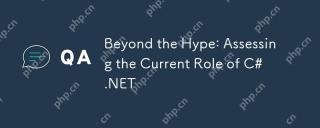
C#.NET is a powerful development platform that combines the advantages of the C# language and .NET framework. 1) It is widely used in enterprise applications, web development, game development and mobile application development. 2) C# code is compiled into an intermediate language and is executed by the .NET runtime environment, supporting garbage collection, type safety and LINQ queries. 3) Examples of usage include basic console output and advanced LINQ queries. 4) Common errors such as empty references and type conversion errors can be solved through debuggers and logging. 5) Performance optimization suggestions include asynchronous programming and optimization of LINQ queries. 6) Despite the competition, C#.NET maintains its important position through continuous innovation.
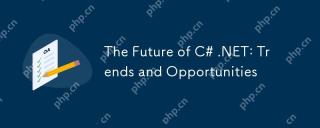
The future trends of C#.NET are mainly focused on three aspects: cloud computing, microservices, AI and machine learning integration, and cross-platform development. 1) Cloud computing and microservices: C#.NET optimizes cloud environment performance through the Azure platform and supports the construction of an efficient microservice architecture. 2) Integration of AI and machine learning: With the help of the ML.NET library, C# developers can embed machine learning models in their applications to promote the development of intelligent applications. 3) Cross-platform development: Through .NETCore and .NET5, C# applications can run on Windows, Linux and macOS, expanding the deployment scope.
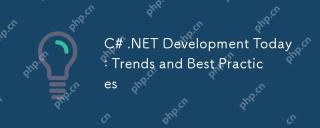
The latest developments and best practices in C#.NET development include: 1. Asynchronous programming improves application responsiveness, and simplifies non-blocking code using async and await keywords; 2. LINQ provides powerful query functions, efficiently manipulating data through delayed execution and expression trees; 3. Performance optimization suggestions include using asynchronous programming, optimizing LINQ queries, rationally managing memory, improving code readability and maintenance, and writing unit tests.
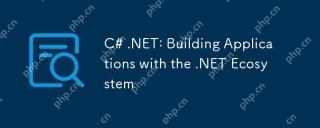
How to build applications using .NET? Building applications using .NET can be achieved through the following steps: 1) Understand the basics of .NET, including C# language and cross-platform development support; 2) Learn core concepts such as components and working principles of the .NET ecosystem; 3) Master basic and advanced usage, from simple console applications to complex WebAPIs and database operations; 4) Be familiar with common errors and debugging techniques, such as configuration and database connection issues; 5) Application performance optimization and best practices, such as asynchronous programming and caching.
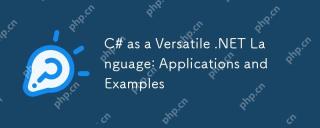
C# is widely used in enterprise-level applications, game development, mobile applications and web development. 1) In enterprise-level applications, C# is often used for ASP.NETCore to develop WebAPI. 2) In game development, C# is combined with the Unity engine to realize role control and other functions. 3) C# supports polymorphism and asynchronous programming to improve code flexibility and application performance.
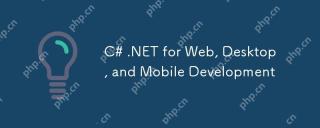
C# and .NET are suitable for web, desktop and mobile development. 1) In web development, ASP.NETCore supports cross-platform development. 2) Desktop development uses WPF and WinForms, which are suitable for different needs. 3) Mobile development realizes cross-platform applications through Xamarin.
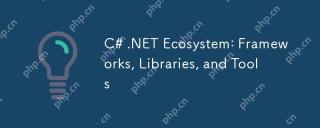
The C#.NET ecosystem provides rich frameworks and libraries to help developers build applications efficiently. 1.ASP.NETCore is used to build high-performance web applications, 2.EntityFrameworkCore is used for database operations. By understanding the use and best practices of these tools, developers can improve the quality and performance of their applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
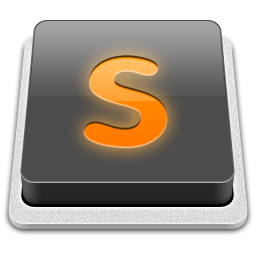
SublimeText3 Mac version
God-level code editing software (SublimeText3)
