Static constructors in C# are constructors implemented to be invoked only once and only during the creation of the reference for a static member implemented in the class. The primary function for a static constructor is to initialize the static members for the class and only once execution. The static constructor as the name suggests does not allow explicit control to the user but is executed automatically upon the invoke of the instance of the class, Further, the static constructor doesn’t take any parameter or access declaration in its definition, thus it can’t be called directly. Static constructors cannot be inherited or overloaded and only accessible to the CLR (Common Language Runtime).
Features and Uses of Static Constructors in C#
Following are some features and uses of static constructors in c# explained in details:
Features for Static Constructor
The following features describe the static constructor:
- The user does not have any control over the static constructor on runtime. These are predefined in the code before precompiling and the value remains fixed for the entire duration.
- The static constructor operation can be further broken down into two stages i.e. static data members initializing and static action execution which occurs in the sequential order as defined.
- A static method does not have any access modifier in its definitions and hence cannot be called upon by other components in the program.
Uses for Static Constructor
Static constructors find its major use in log programs where it is used to write the parameter entries initialized during each instance. Static constructors are the ideal positions to create database connections as they are loaded first and remain static throughout. In C# programming language the static constructors following the following Syntax.
Syntax:
class ABC { //defining the static constructor using the same name as class static ABC() { //constructor specific code comes here } }
How Static Constructors Work in C#?
Here are some basic working principle of static constructor in C# which are as follows:
- When a class or an object of the class is created as a struct, constructors are called upon to create the data members associated with the class. These constructors have the same name as the class.
- In order to understand the concept of static constructors, we would first need to understand the concept behind static methods and classes.
- A static class is differentiated from a regular class due to the fact that the static class cannot be instantiated i.e. the new keyword cannot be used for creating the new instance of the class.
- Hence the class cannot be accessed using the new instance and needs to be called upon by the static class name itself. These classes are used to work upon the input fields and operated to create an initial and fixed value of the input parameters.
- A static constructor as the name suggest is used to set up or initialize any static data required at the pre-rendered stages of the class construct. The static constructor is called upon before the first instance of the class is created or the reference is looked upon for any static members.
- Thus one can define static constructors as the first instance of the class that is created in run time, also this is a single occurrence event and is not repeated again.
Examples to Implement Static Constructors
Here are some of the examples of static constructor in C# which are given below:
Example #1
Code:
using System; namespace HappyConstructor { class Happy { //let us declare and initialise the static data members private static int id = 7; public static int Id { get { return id; } } public static void printVariable() { Console.WriteLine("Happy.id = " + id); } static void Main(string[] args) { //let us print the value of ID from the class Happy. printVariable (); } } }
Output:
Explanation: In the above, the static member id is declared for use and initialized to the initial value of 10.
Example #2
Code:
using System; namespace Happy1Constructor { class Happy1 { private static int Id; //data member (id) is set conditionally here to show actions of a Static constructor static Happy1 () { if (Happy1.Id <p><strong>Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534757544672.png?x-oss-process=image/resize,p_40" class="lazy" alt="Static Constructor in C#" ></p> <p><strong>Explanation:</strong> In the example above the constructor is conditionally dependent upon the Happy.cs file generated in example1. Here the static constructor initializes itself. since the value is in the first case the Id field generated is 7 and as per the conditional operator if the value of the field is less than 10 then the value for the Id field for the Happy1 constructor shall be 25. This is a classic example of initializing a static constructor upon the first instance of loading of the class. The static constructor makes use of this feature to preload the input parameters for the program referencing.</p><h3 id="Conclusion">Conclusion</h3> <p>A static constructor is used to initialize any static data and or in performance of any particular actions that need to be performed once and only once for the program. This constructor is called upon before any of the objects of the class is initiated or any of the members are loaded on to the run time environment.</p>
The above is the detailed content of Static Constructor in C#. For more information, please follow other related articles on the PHP Chinese website!
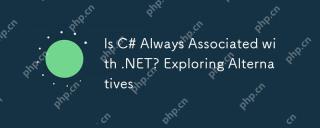
C# is not always tied to .NET. 1) C# can run in the Mono runtime environment and is suitable for Linux and macOS. 2) In the Unity game engine, C# is used for scripting and does not rely on the .NET framework. 3) C# can also be used for embedded system development, such as .NETMicroFramework.
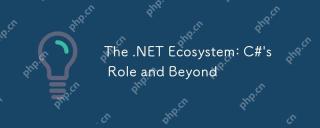
C# plays a core role in the .NET ecosystem and is the preferred language for developers. 1) C# provides efficient and easy-to-use programming methods, combining the advantages of C, C and Java. 2) Execute through .NET runtime (CLR) to ensure efficient cross-platform operation. 3) C# supports basic to advanced usage, such as LINQ and asynchronous programming. 4) Optimization and best practices include using StringBuilder and asynchronous programming to improve performance and maintainability.
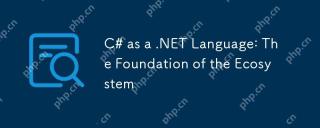
C# is a programming language released by Microsoft in 2000, aiming to combine the power of C and the simplicity of Java. 1.C# is a type-safe, object-oriented programming language that supports encapsulation, inheritance and polymorphism. 2. The compilation process of C# converts the code into an intermediate language (IL), and then compiles it into machine code execution in the .NET runtime environment (CLR). 3. The basic usage of C# includes variable declarations, control flows and function definitions, while advanced usages cover asynchronous programming, LINQ and delegates, etc. 4. Common errors include type mismatch and null reference exceptions, which can be debugged through debugger, exception handling and logging. 5. Performance optimization suggestions include the use of LINQ, asynchronous programming, and improving code readability.
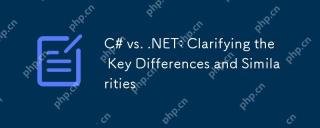
C# is a programming language, while .NET is a software framework. 1.C# is developed by Microsoft and is suitable for multi-platform development. 2..NET provides class libraries and runtime environments, and supports multilingual. The two work together to build modern applications.
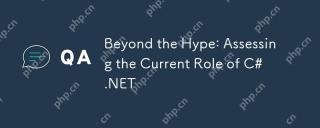
C#.NET is a powerful development platform that combines the advantages of the C# language and .NET framework. 1) It is widely used in enterprise applications, web development, game development and mobile application development. 2) C# code is compiled into an intermediate language and is executed by the .NET runtime environment, supporting garbage collection, type safety and LINQ queries. 3) Examples of usage include basic console output and advanced LINQ queries. 4) Common errors such as empty references and type conversion errors can be solved through debuggers and logging. 5) Performance optimization suggestions include asynchronous programming and optimization of LINQ queries. 6) Despite the competition, C#.NET maintains its important position through continuous innovation.
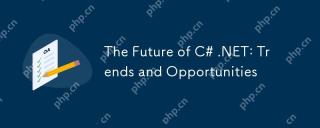
The future trends of C#.NET are mainly focused on three aspects: cloud computing, microservices, AI and machine learning integration, and cross-platform development. 1) Cloud computing and microservices: C#.NET optimizes cloud environment performance through the Azure platform and supports the construction of an efficient microservice architecture. 2) Integration of AI and machine learning: With the help of the ML.NET library, C# developers can embed machine learning models in their applications to promote the development of intelligent applications. 3) Cross-platform development: Through .NETCore and .NET5, C# applications can run on Windows, Linux and macOS, expanding the deployment scope.
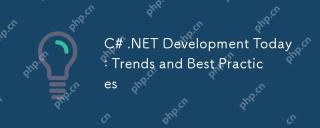
The latest developments and best practices in C#.NET development include: 1. Asynchronous programming improves application responsiveness, and simplifies non-blocking code using async and await keywords; 2. LINQ provides powerful query functions, efficiently manipulating data through delayed execution and expression trees; 3. Performance optimization suggestions include using asynchronous programming, optimizing LINQ queries, rationally managing memory, improving code readability and maintenance, and writing unit tests.
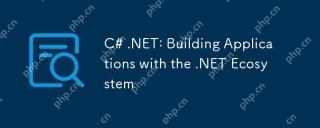
How to build applications using .NET? Building applications using .NET can be achieved through the following steps: 1) Understand the basics of .NET, including C# language and cross-platform development support; 2) Learn core concepts such as components and working principles of the .NET ecosystem; 3) Master basic and advanced usage, from simple console applications to complex WebAPIs and database operations; 4) Be familiar with common errors and debugging techniques, such as configuration and database connection issues; 5) Application performance optimization and best practices, such as asynchronous programming and caching.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
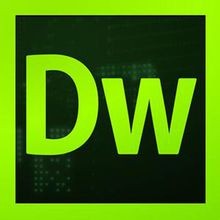
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
