Constructor plays a very important role in Object-Oriented Programming. Let us understand the role of constructor in C# with the help of the following points:
- A constructor is a special method present inside a class responsible for initializing the class variables.
- Its name is the same as the class name.
- It automatically gets executed when we create an instance of the class.
- A constructor does not return any value.
- If we do not define a constructor, an implicit constructor is always provided by the class, which is called the default constructor.
Syntax:
public class Student() { //constructor public Student() { //code } }
Here, public Student() is a method that does not have any return type, not even void, and its name is the same as the class name, i.e. ‘Student’. Thus, this method is the constructor of this class.
When we create an object of this class using:
Student obj = new Student();
Then the code inside the constructor will be executed.
Working of Constructor in C#
1. The constructor initializes data members for the new object. It is invoked by the ‘new’ operator immediately after memory gets allocated to the new object.
2. Explicit constructors (constructors defined by the user) can be parameterless or parameterized. If it is parameterized, then the values passed to the constructor can be assigned to the class’s data members.
3. The implicit constructor initializes variables of the class with the same value even if we create multiple instances of that class.
Example:
Code:
using System; public class ConstructorDemo { public int num = 10; public static void Main() { ConstructorDemo obj1 = new ConstructorDemo(); ConstructorDemo obj2 = new ConstructorDemo(); ConstructorDemo obj3 = new ConstructorDemo(); Console.WriteLine("obj1.num = "+obj1.num+"\nobj2.num = "+obj2.num +"\nobj3.num = "+obj3.num); } }
Output:
Pictorial Representation of Above Program:
4. Explicit constructor with parameters allows us to initialize variables of the class with a different value each time we create an instance of that class.
Example:
Code:
using System; public class ConstructorDemo { public int num; //explicit constructor public ConstructorDemo(int num) { this.num = num; } public static void Main() { ConstructorDemo obj1 = new ConstructorDemo(10); ConstructorDemo obj2 = new ConstructorDemo(20); ConstructorDemo obj3 = new ConstructorDemo(30); Console.WriteLine("obj1.num = "+obj1.num+"\nobj2.num = "+obj2.num +"\nobj3.num = "+obj3.num); } }
Output:
Pictorial Representation of Above Program:
Top 5 Types of Constructor in C#
C# provides five types of constructors. They are as follows:
1. Default Constructor
- A constructor without any parameter is called Default Constructor. If we do not define it explicitly, then it will be implicitly provided by the compiler.
- In such a case, we can call it an implicit constructor. Default or implicit constructor initializes all data members of the class with their default values, such as all numeric fields to zero and all string and object fields to null.
Example:
Code:
using System; public class DefaultConstructor { public int num; public string str; } public class Demo { public static void Main() { DefaultConstructor obj = new DefaultConstructor(); Console.WriteLine("obj.num = "+obj.num+"\nobj.str = "+obj.str); } }
Output:
2. Parameterized Constructor
A constructor with at least one parameter is called Parameterized Constructor. Parameters to the constructor can be passed while creating the instance of the class. It allows us to initialize each instance of a class with different values.
Example:
Code:
using System; public class ParameterizedConstructor { public int num; public string str; //parameterized constructor public ParameterizedConstructor(int num, string str) { this.num = num; this.str = str; } } public class Demo { public static void Main() { //passing values to constructor while creating instance ParameterizedConstructor obj = new ParameterizedConstructor(50, "constructor"); Console.WriteLine("obj.num = "+obj.num+"\nobj.str = "+obj.str); } }
Output:
3. Copy Constructor
It is a parameterized constructor that takes the same class’s object as a parameter. It copies the existing object’s value (which is passed as a parameter) to the newly created object instantiated by the constructor. We can say that it copies data of one object to another object.
Example:
Code:
using System; public class CopyConstructor { public int num; public CopyConstructor(int num) { this.num = num; } //copy constructor public CopyConstructor(CopyConstructor obj) { num = obj.num; } } public class Demo { public static void Main() { CopyConstructor obj1 = new CopyConstructor(50); //passing same class's object as parameter CopyConstructor obj2 = new CopyConstructor(obj1); Console.WriteLine("Original object:"); Console.WriteLine("obj1.num = "+obj1.num); Console.WriteLine("\nCopied object:"); Console.WriteLine("obj2.num = "+obj2.num); } }
Output:
4. Static Constructor
- It can be defined by prefixing the constructor name with a keyword. It is implicitly defined by the compiler (if not defined explicitly) if the class contains any static variable.
- It is the first block to be executed in the class and will be called automatically. It will be executed only once, irrespective of the number of class instances. It is parameterless and doesn’t accept any access modifier.
Example:
Code:
using System; public class StaticConstructor { //static constructor static StaticConstructor() { Console.WriteLine("Static constructor executed"); } public static void Display() { Console.WriteLine("\nDisplay method executed"); } } public class Demo { public static void Main() { StaticConstructor.Display(); } }
Output:
5. Private Constructor
A constructor created with a private specifier is called a private constructor. We cannot create an instance of the class if it contains only a private constructor, and it does not allow other classes to derive from this class. Used in class that contains only static members.
Example:
Code:
using System; public class PrivateConstructor { public static int num = 100; //private constructor private PrivateConstructor() { } } public class Demo { public static void Main() { //PrivateConstructor obj = new PrivateConstructor(); //Error Console.WriteLine("num = "+PrivateConstructor.num); } }
Output:
Conclusion
If we define any type of constructor in a class, then there will not be any implicit constructor in the class provided by the compiler. Like methods, parameterized constructors can also be overloaded with different numbers of parameters. Constructors defined implicitly by the compiler are always public.
Recommended Article
This is a guide to Constructor in C#. Here we discuss the types of Constructor in C# and its Work along with Code Implementation and Output. You can also go through our other suggested articles to learn more –
- Constructor in JavaScript
- Constructor in C++
- Copy Constructor in C#
- Static Constructor in C#
The above is the detailed content of Constructor in C#. For more information, please follow other related articles on the PHP Chinese website!
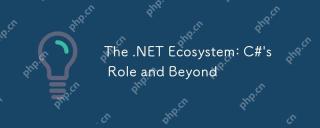
C# plays a core role in the .NET ecosystem and is the preferred language for developers. 1) C# provides efficient and easy-to-use programming methods, combining the advantages of C, C and Java. 2) Execute through .NET runtime (CLR) to ensure efficient cross-platform operation. 3) C# supports basic to advanced usage, such as LINQ and asynchronous programming. 4) Optimization and best practices include using StringBuilder and asynchronous programming to improve performance and maintainability.
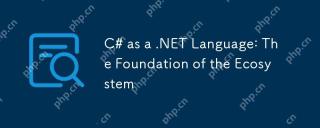
C# is a programming language released by Microsoft in 2000, aiming to combine the power of C and the simplicity of Java. 1.C# is a type-safe, object-oriented programming language that supports encapsulation, inheritance and polymorphism. 2. The compilation process of C# converts the code into an intermediate language (IL), and then compiles it into machine code execution in the .NET runtime environment (CLR). 3. The basic usage of C# includes variable declarations, control flows and function definitions, while advanced usages cover asynchronous programming, LINQ and delegates, etc. 4. Common errors include type mismatch and null reference exceptions, which can be debugged through debugger, exception handling and logging. 5. Performance optimization suggestions include the use of LINQ, asynchronous programming, and improving code readability.
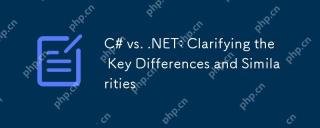
C# is a programming language, while .NET is a software framework. 1.C# is developed by Microsoft and is suitable for multi-platform development. 2..NET provides class libraries and runtime environments, and supports multilingual. The two work together to build modern applications.
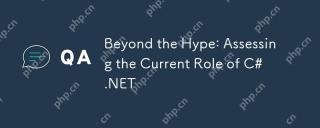
C#.NET is a powerful development platform that combines the advantages of the C# language and .NET framework. 1) It is widely used in enterprise applications, web development, game development and mobile application development. 2) C# code is compiled into an intermediate language and is executed by the .NET runtime environment, supporting garbage collection, type safety and LINQ queries. 3) Examples of usage include basic console output and advanced LINQ queries. 4) Common errors such as empty references and type conversion errors can be solved through debuggers and logging. 5) Performance optimization suggestions include asynchronous programming and optimization of LINQ queries. 6) Despite the competition, C#.NET maintains its important position through continuous innovation.
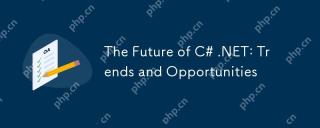
The future trends of C#.NET are mainly focused on three aspects: cloud computing, microservices, AI and machine learning integration, and cross-platform development. 1) Cloud computing and microservices: C#.NET optimizes cloud environment performance through the Azure platform and supports the construction of an efficient microservice architecture. 2) Integration of AI and machine learning: With the help of the ML.NET library, C# developers can embed machine learning models in their applications to promote the development of intelligent applications. 3) Cross-platform development: Through .NETCore and .NET5, C# applications can run on Windows, Linux and macOS, expanding the deployment scope.
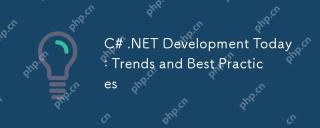
The latest developments and best practices in C#.NET development include: 1. Asynchronous programming improves application responsiveness, and simplifies non-blocking code using async and await keywords; 2. LINQ provides powerful query functions, efficiently manipulating data through delayed execution and expression trees; 3. Performance optimization suggestions include using asynchronous programming, optimizing LINQ queries, rationally managing memory, improving code readability and maintenance, and writing unit tests.
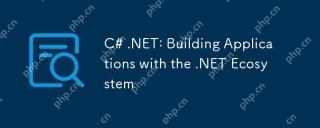
How to build applications using .NET? Building applications using .NET can be achieved through the following steps: 1) Understand the basics of .NET, including C# language and cross-platform development support; 2) Learn core concepts such as components and working principles of the .NET ecosystem; 3) Master basic and advanced usage, from simple console applications to complex WebAPIs and database operations; 4) Be familiar with common errors and debugging techniques, such as configuration and database connection issues; 5) Application performance optimization and best practices, such as asynchronous programming and caching.
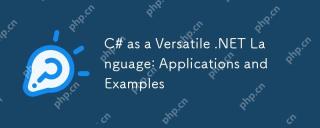
C# is widely used in enterprise-level applications, game development, mobile applications and web development. 1) In enterprise-level applications, C# is often used for ASP.NETCore to develop WebAPI. 2) In game development, C# is combined with the Unity engine to realize role control and other functions. 3) C# supports polymorphism and asynchronous programming to improve code flexibility and application performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
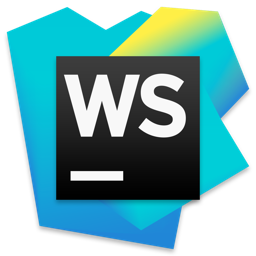
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
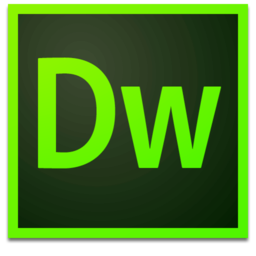
Dreamweaver Mac version
Visual web development tools
