In C#, the rectangular arrays or multidimensional arrays refer to the organization of the elements in a matrix format. A multidimensional array can only be of two or three dimensions. Dimensions of an array refer to the organization format of the data in the variable. Thus we can define a multidimensional array as an organization of elements in series or sequence as rows or columns.
Syntax:
Below is the syntax of Multidimensional Arrays:
Declaration of the 2D array.
int[,] x=new int[1,2];
Declaration of the 3D array.
int[,,] x=new int[1,2,3];
The syntax above specifies the format to declare two-dimensional and 3-dimensional arrays (x). the first array contains two elements, 1 and 2, whereas the three-dimensional array contains the element 1,2,3.
Initialization of the Multidimensional Arrays
A multidimensional array can be initialized in three different ways
1. Complete Declaration
int[,] x = new int[6,6];
The above specification initializes a two-dimensional array completely, including the use of array type, array size, and the new operator.
2. Initialising without Using the New Operator
int[,] x = { { 3,2,1 }, { 6,5,4 }, { 9,8,7 } };
3. Initializing the Array without Declaring the Size
int[,] x = new int[,]{ { 3,2,1 }, { 6,5,4 }, { 9,8,7 } };
Examples of C# multidimensional array
Below are the examples of Multidimensional Arrays in C#:
Example #1
Program to illustrate declaring and initialization of a multidimensional array. The example below illustrates the creation of a multidimensional array in C#.
Code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace ConsoleApplication1 { class Program { public static void Main(string[] args) { int[,] x = { { 3, 2, 1 }, { 6, 5, 4 }, { 9, 8, 7 } }; for (int a = 0; a <p><strong>Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534754320071.png?x-oss-process=image/resize,p_40" class="lazy" alt="C# Multidimensional Arrays" ></p> <h4 id="Example">Example #2</h4> <p>Program to illustrate the initialization, declaration of a two-dimensional array, and access the elements.</p> <p><strong>Code: </strong></p> <pre class="brush:php;toolbar:false">using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace ConsoleApplication1 { class Program { static void Main(string[] args) { /* declaring and initialising a two dimensional array*/ int[,] b = new int[6, 2] { { 1, 2 }, { 4, 3 }, { 5, 6 }, { 8,7 }, { 9 , 10 }, { 2, 3 } }; int i, j; /* accessing each of the elements value for the array */ for (i = 0; i <p><strong>Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534754473163.png?x-oss-process=image/resize,p_40" class="lazy" alt="C# Multidimensional Arrays" ></p> <p>The program above demonstrates the use of indices as a positional marker for accessing the elements of the array in a multidimensional array.</p> <h4 id="Example">Example #3</h4> <p>Program for the addition of two multidimensional arrays.</p> <p><strong>Code:</strong></p> <pre class="brush:php;toolbar:false">using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace ConsoleApplication1 { class Program { public static void Main() { int[,] array1 = new int[3, 3]; int[,] array2 = new int[3, 3]; int[,] resultArray = new int[3, 3]; int i, j; Console.WriteLine("specify the members of the first array: "); for (i = 0; i <p><strong>Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172534754562045.png?x-oss-process=image/resize,p_40" class="lazy" alt="C# Multidimensional Arrays" ></p> <p>Using the program above, we have completed the addition operation on the array, with each element in the first array added to the second array’s counter element. For example, the first element in array1 is 1; similarly, the first element in array2 is 9. The resultant of addition should contain an array with the first element as 10.</p> <h3 id="Advantages-amp-Disadvantages">Advantages & Disadvantages</h3> <p>Below are the advantages and disadvantages of Multidimensional Arrays:</p> <h4 id="Advantages">Advantages</h4>
- Multidimensional arrays can be used to organize subgroups of data within an array; multidimensional arrays can also be used to store data memory addresses in a pointer array.
- In the program, multidimensional arrays have a static size and initialization at the beginning. Any extension in size shall require the relevant size to be specified during initialization.
- Multidimensional arrays enable performing matrix operations and maintaining large data values under the same variable allocation.
- Multidimensional arrays are used to implement stacks, heaps and Queues, and hash tables.
Disadvantages
- The elements are located in contiguous memory locations for an array; hence any insertion and deletion of an element shall be more complex than similar operations on single elements.
- Also, the elements cannot be inserted into the middle of an array.
- Static allocation can cause wastage and failure to release unused memory if it allocates more memory than necessary. This can have a negative impact.
- Multidimensional arrays can be slower when compared to their single-dimensional array counterparts, which is a major disadvantage. To overcome this, we can replace jagged arrays with multidimensional arrays.
The above is the detailed content of C# Multidimensional Arrays. For more information, please follow other related articles on the PHP Chinese website!
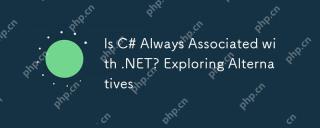
C# is not always tied to .NET. 1) C# can run in the Mono runtime environment and is suitable for Linux and macOS. 2) In the Unity game engine, C# is used for scripting and does not rely on the .NET framework. 3) C# can also be used for embedded system development, such as .NETMicroFramework.
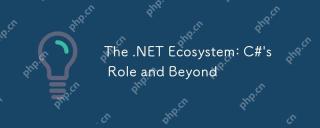
C# plays a core role in the .NET ecosystem and is the preferred language for developers. 1) C# provides efficient and easy-to-use programming methods, combining the advantages of C, C and Java. 2) Execute through .NET runtime (CLR) to ensure efficient cross-platform operation. 3) C# supports basic to advanced usage, such as LINQ and asynchronous programming. 4) Optimization and best practices include using StringBuilder and asynchronous programming to improve performance and maintainability.
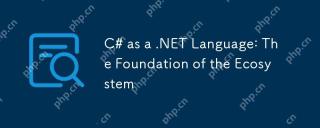
C# is a programming language released by Microsoft in 2000, aiming to combine the power of C and the simplicity of Java. 1.C# is a type-safe, object-oriented programming language that supports encapsulation, inheritance and polymorphism. 2. The compilation process of C# converts the code into an intermediate language (IL), and then compiles it into machine code execution in the .NET runtime environment (CLR). 3. The basic usage of C# includes variable declarations, control flows and function definitions, while advanced usages cover asynchronous programming, LINQ and delegates, etc. 4. Common errors include type mismatch and null reference exceptions, which can be debugged through debugger, exception handling and logging. 5. Performance optimization suggestions include the use of LINQ, asynchronous programming, and improving code readability.
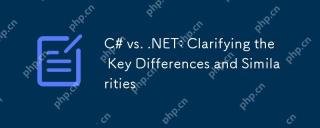
C# is a programming language, while .NET is a software framework. 1.C# is developed by Microsoft and is suitable for multi-platform development. 2..NET provides class libraries and runtime environments, and supports multilingual. The two work together to build modern applications.
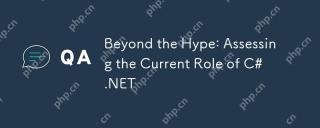
C#.NET is a powerful development platform that combines the advantages of the C# language and .NET framework. 1) It is widely used in enterprise applications, web development, game development and mobile application development. 2) C# code is compiled into an intermediate language and is executed by the .NET runtime environment, supporting garbage collection, type safety and LINQ queries. 3) Examples of usage include basic console output and advanced LINQ queries. 4) Common errors such as empty references and type conversion errors can be solved through debuggers and logging. 5) Performance optimization suggestions include asynchronous programming and optimization of LINQ queries. 6) Despite the competition, C#.NET maintains its important position through continuous innovation.
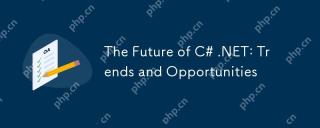
The future trends of C#.NET are mainly focused on three aspects: cloud computing, microservices, AI and machine learning integration, and cross-platform development. 1) Cloud computing and microservices: C#.NET optimizes cloud environment performance through the Azure platform and supports the construction of an efficient microservice architecture. 2) Integration of AI and machine learning: With the help of the ML.NET library, C# developers can embed machine learning models in their applications to promote the development of intelligent applications. 3) Cross-platform development: Through .NETCore and .NET5, C# applications can run on Windows, Linux and macOS, expanding the deployment scope.
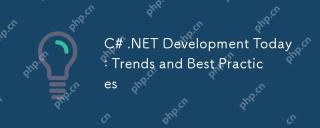
The latest developments and best practices in C#.NET development include: 1. Asynchronous programming improves application responsiveness, and simplifies non-blocking code using async and await keywords; 2. LINQ provides powerful query functions, efficiently manipulating data through delayed execution and expression trees; 3. Performance optimization suggestions include using asynchronous programming, optimizing LINQ queries, rationally managing memory, improving code readability and maintenance, and writing unit tests.
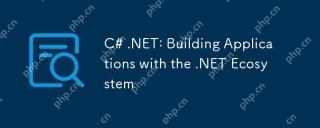
How to build applications using .NET? Building applications using .NET can be achieved through the following steps: 1) Understand the basics of .NET, including C# language and cross-platform development support; 2) Learn core concepts such as components and working principles of the .NET ecosystem; 3) Master basic and advanced usage, from simple console applications to complex WebAPIs and database operations; 4) Be familiar with common errors and debugging techniques, such as configuration and database connection issues; 5) Application performance optimization and best practices, such as asynchronous programming and caching.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
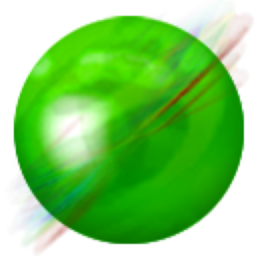
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
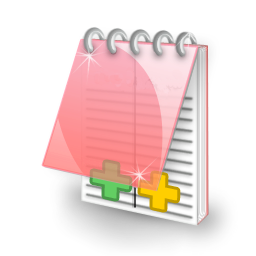
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
