String initialization is one of the basic parts of programming. String initialization means assigning a value to the variable before it’s used in the java program. String initialization can be done in two ways:
- Object Initialization
- Direct Initialization
The string is an object in java. String refers to some sequence of the characters. It’s also known as an array of characters. A String is always indicated in the quotes; even quotes are not part of the String. The string initialization also takes place in two steps, the first one is declaration & the second one is initialization. String declaration refers to declaring a String without assigning any value explicitly. In most of the use cases, the only declaration of the String requires not initialization.
ADVERTISEMENT Popular Course in this category JAVA MASTERY - Specialization | 78 Course Series | 15 Mock TestsTypes of String Initialization in Java
In java, a String is initialized in multiple ways. Either by using constructor or by using Literal. There is a difference in initializing String by using a new keyword & using Literal. Initializing String using new keywords every time create a new java object. A variable that is defined but not initialized can not be used in the program because it’s not holding any value.
1. Object Initialization
String initialization using the new keyword. Initializing String using a new keyword creates a new object in the heap of memory. String initialized through an object is mutable means to say the value of the string can be reassigned after initialization.
String strMsg = new String("Be specific towards your goal!");
When initiating objects using the constructor, java compilers create a new object in the heap memory. Heap memory is a reserved memory for the JVM.
2. Direct Initialization
String initialization using Literals. String initializing using the Literal creates an object in the pooled area of memory.
String strMsg = "Be specific towards your goal!";
When initializing String using Literal & value assigned to it already initialized in another String. In such a scenario, String created through Literal doesn’t create a new object. It just passed the reference to the earlier created object.
Examples of String Initialization in Java
Following explained are the different examples of string initialization in java.
Example #1
In the below-created String, both the String are created using the Literal.
Code:
String ObjStringFirst="Welcome"; String ObjStringSecond="Welcome";
In the above-given String, we are creating objects using String Literal. In such a situation, if the object is already existing with the same value, it will return the same object; otherwise, it will create a new object.
Example #2
In the below-given example, we can see how Strings are initialized.
Code:
public class StringExample { public static void main(String[] args) { String nameFirst = "Alister"; String nameSecond = "Campbell"; String nameThird = new String("Adam"); String nameFourth = new String("Martin"); //String nameFifth; String strNullFirst = null; String strNullSecond = null; String strNullThird = null; System.out.println("String initialized :"); //printing variable which are initialized System.out.println("First : " + nameFirst); System.out.println("Second : " + nameSecond); System.out.println("Third : " + nameThird); //printing variable which are declared but not initialized //System.out.println("String declared but not initialized :"); //System.out.println("Fifth : " + nameFifth); } }
Output:
Example #3
In this example, a declaration of the String variable is given. It contains a variable that is declared but not initialized on compiling the program.
Code:
public class StringExample { public static void main(String[] args) { String nameFirst; //printing variable which are declared but not initialized System.out.println("String declared but not initialized :"); System.out.println("Fifth : " + nameFirst); } }
Output:
The output of the above program is given below. The above-given program will generate the error on compilation. The string is declared but not initialized.
After the String declaration, if any value is assigned later for the declared String variable, then compilation will not throw any error (as String initialization takes in two parts). The first one is declaration & the second one is an assignment.
Example #4
In this example, let’s go with the String initialization with empty values. All the String variables initiated through empty values will have different objects for all three as String initialized through Literal will create an Object in the String pool while String initialized through new keyword will create objects in the heap area of memory.
Code:
public class StringExample { public static void main(String[] args) { String strVal1 = ""; String strVal2 = new String(); String strVal3 = new String(""); //printing variable which are initialized with empty values System.out.println("String Values :"); System.out.println("First : " + strVal1); System.out.println("Second : " + strVal2); System.out.println("Third : " + strVal3); } }
Output:
In the above-given program, even the object for Strings is different, but the value assigned to the String is the same. Below given output shows how empty values are assigned to each String variable.
Example #5
In the below-given program, a comparison of the above-initialized strings is given. Using here three-way of comparing the String.
1. == operator: In java == operator compare the references, not the values.
2. equals() method: It compares the variable’s value, not the references; hence, if the value matches both the String variable, it returns true other false.
3. compareTo() method: compareTo() method check the values & if values are identical then it return 0 otherwise it return + & – based on the below given comparison.
- It returns 0 if str1 == str2
- It returns positive value if str1 > str2
- It returns negative value if str1
Code:
public class StringExample { public static void main(String[] args) { String strVal1 = ""; String strVal2 = new String(); String strVal3 = new String(""); //printing variable which are initialized with empty values System.out.println("String Values :"); System.out.println("First : " + strVal1); System.out.println("Second : " + strVal2); System.out.println("Third : " + strVal3); System.out.println(" \nComparing strings using == operator :"); System.out.println("Comparing first two strings :"); System.out.println(strVal1 == strVal2); System.out.println("Comparing last two strings :"); System.out.println(strVal2 == strVal3); System.out.println("Comparing first & last strings :"); System.out.println(strVal1 == strVal3); System.out.println(" \nComparing strings using equals method :"); System.out.println("Comparing first two strings :"); System.out.println(strVal1.equals(strVal2)); System.out.println("Comparing last two strings :"); System.out.println(strVal2.equals(strVal3)); System.out.println("Comparing first & last strings :"); System.out.println(strVal1.equals(strVal3)); System.out.println(" \nComparing strings using compareTo method :"); System.out.println("Comparing first two strings :"); System.out.println(strVal1.compareTo(strVal2)); System.out.println("Comparing last two strings :"); System.out.println(strVal2.compareTo(strVal3)); System.out.println("Comparing first & last strings :"); System.out.println(strVal1.compareTo(strVal3)); } }
Output:
The output of the comparing string program is given below. It contains the output after comparing the Strings in three different ways; thus, outcomes of the comparison are also different according to the method applied.
Conclusion
The above-given article explains the initialization of String & different types of ways to initialize the String in java. Also given examples in the article describe the internal creation of objects when initiating String using Literal. It also describes the difference between declaration & initialization.
The above is the detailed content of String Initialization in Java. For more information, please follow other related articles on the PHP Chinese website!
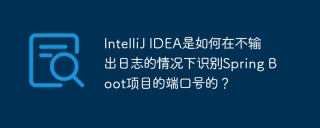
Start Spring using IntelliJIDEAUltimate version...
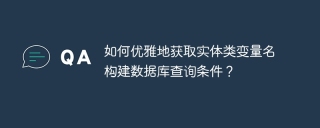
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
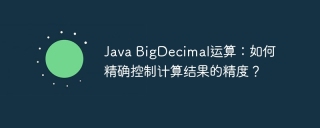
Java...
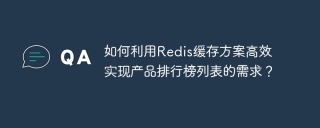
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
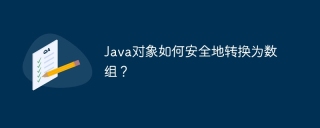
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
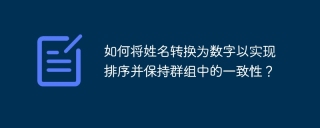
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
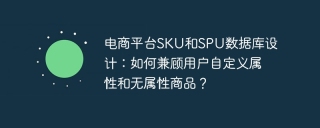
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
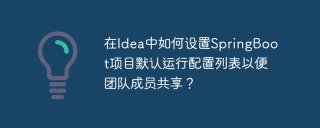
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
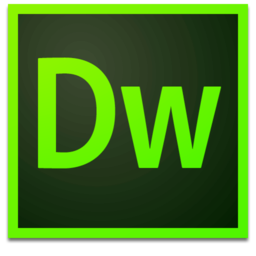
Dreamweaver Mac version
Visual web development tools
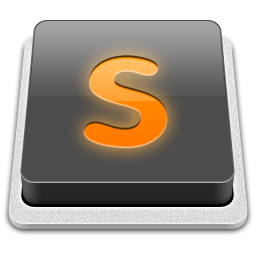
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
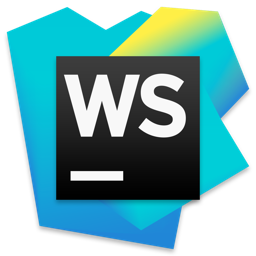
WebStorm Mac version
Useful JavaScript development tools