Java is a computing platform for application development and an object-oriented, Class-based, and Concurrent programming language which means many statements can be executed at the same time instead of sequentially executing it. It can run on all platforms and is free to access.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
The following are important points:
- Java is class-based and object-oriented.
- It is platform-independent which means Java code can be compiled on any operating system and made to run on the same or any other operating system.
- It supports concurrency, which means multiple processes can execute the code at the same time. Many Java programming statements can be executed together instead of executing one by one in sequence.
Why do we Use Java?
Since its evolution, it has continuously gained popularity in the market, and it is one of the most commonly used languages for developing applications today.
The following are the most important features:
- Simplicity: Java was developed keeping in mind the complexities in other programming languages like C or C++. It has made the developer’s life comparatively easy as Java does not make use of pointers and has a built-in memory management system.
- Portability: As already covered, java source code can be developed and compiled on one machine and can be made to run on the same or any other operating system. Therefore a Java program can be easily ported onto any other machine, thus providing portability.
- Object-Oriented: Everything is treated as an object, and all the operations involved in application development are completed using these objects.
- Dynamic Capability: Applications developed using Java can adapt to changing execution environments. This is because this programming language has features like dynamic memory allocation in which the amount of memory allocated changes according to the environment, which in turn provides high performance.
- High Security: In terms of security, it is operated on byte code which is not readable in nature. It runs source code inside a secured sandbox and does not allow any external intervention. Therefore it allows developers to build tamper-resilient and virus-free applications, thus providing high security.
- Robust: It is developed keeping in mind all possibilities, and therefore there is no existence of error. It allows development code that handles all possible errors. Also, it has a strong type of checking that makes our source code robust.
- Multithreading: It supports creating multiple threads for execution, thus providing high performance. Also, it supports the synchronization mechanism to maintain synchronization between different threads.
- Interpreted: The code is converted into byte code, which is interpreted by the Java run time environment.
- Distributed: It supports developing distributed applications. It provides features like Remote Method Invocation, through which a program can communicate with another program present in the remote machines through the network and generates the desired output.
- Performance: It provides high performance as it uses bytecode, which can be translated into machine code with ease and high speed. It has Just in time compiler through which high performance is achieved.
Because of all the above-explained features, java is commonly used and popular for application development.
What can we do with Java?
Now we will see different applications:
Here are some of the common domains in which it is used:
1. Banking and Financial Domain: The banking domain makes use of Java for handling transactions. It performs exceptionally well while efficiently handling millions of transactions.
2. Information Technology: Using Java following types of applications can be developed.
- Web Application Development: This refers to website development. In web application development, java provides server-side technologies that receive data from front-end user interfaces and provide data to the back-end logical processing layer, and after processing, data is returned to the front end through these server-side technologies.
The following are some server-side technologies provided:
- Spring Framework
- Spring Boot
Apart from server-side technologies, one of the most commonly used application server, apache tomcat, is developed using java.
- Android Application Development: Java is also used for the development of android applications. Android applications are developed using Java API.
- Desktop Applications: It is also used to develop standalone desktop applications. It has Abstract Windowing Toolkit (AWT) to allow GUI development.
- Enterprise Applications: It provides Java Enterprise Edition, which is popular for developing enterprise applications. Applications developed using Java EE are mainly business applications that are scalable, reliable, large-scale, multi-tier, and secure applications.
- Scientific Applications: Applications involving scientific and mathematical calculations can be developed as the core programming language. Also, it is preferred for performing scientific analysis on huge data.
- Big Data: Map-reduce framework for handling big data processing is written in Java.
- Cloud Applications: It provides features that can be used to build applications used in Saas, Paas, and Iaas cloud development. It helps companies to develop their applications remotely or serve them to share their data or information with others over the network.
- Gaming Applications: It has the support of one of the most popular 3D-Engine, jMonkeyEngine, which can be used to design three-dimensional games.
3. Stock Market: It is used for developing algorithms involving stock market analysis.
4. Retail: It is also used in developing billing applications used in malls, shops, and other places.
Examples
Let us see a basic example. The main method is the entry point to the java application.
Example #1
In this example, we will see how the main method is defined.
Code:
package com.edubca.javademo; public class JavaDemo{ public static void main(String args[]){ String str="This is Edubca Java Training"; System.out.println(str); //printing on console } }
Output:
Explanation:
- We have seen how a class is declared and the main method’s declaration.
- Inside the main method, System.out.println () is used, which accepts a string as an argument and is used to print the specified string on the console.
Example #2
This example shows how mathematical calculations like add, subtract, multiply, and divide are implemented.
Code:
package com.edubca.javademo; public class JavaDemo{ public int add(int a, int b){ int c= a+b; return c; } public int substract(int a, int b){ int c= a-b; return c; } public int multiply(int a, int b){ int c= a*b; return c; } public int divide(int a, int b){ int c= a/b; return c; } public static void main(String[] args) throws Exception { // Declare variables int a= 10; int b= 5; //create instance of JavaDemo class JavaDemo jdemo= new JavaDemo (); int addresult=jdemo.add(a,b); // invoke add method of JavaDemo int substractresult=jdemo. substract (a,b); // invoke substract method of JavaDemo int multiplyresult=jdemo.multiply(a,b); // invoke multiply method of JavaDemo int divideresult=jdemo.divide(a,b); // invoke divide method of JavaDemo System.out.println("Addition of 10 and 5 is " + addresult ); System.out.println("Subtraction of 10 and 5 is " + substractresult ); System.out.println("Multiplication of 10 and 5 is " + multiplyresult ); System.out.println("Division of 10 and 5 is "+ divideresult ); } }
Output:
Explanation:
- In the above example, we have seen how to create a class, declare methods, create the class object, and call declared methods through the object.
Example #3
In this example, we will see a program to find all prime numbers between two numbers.
Code:
package com.edubca.javademo; public class JavaDemo{ public static void main(String args[]){ //declare variables int low=10; int high=80; System.out.println("Prime numbers between 10 and 80 are : "); // while loop while(low <p><strong>Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172500159819341.png?x-oss-process=image/resize,p_40" class="lazy" alt="What is Java?" ></p> <p><strong>Explanation:</strong></p>
- The above program shows the use of a while loop and a loop.
- The above program will produce the following output.
Example #4
In this example, we will see how to print the Fibonacci series up to a number.
Code:
package com.edubca.javademo; public class JavaDemo{ // declaring static method printfabonacci static int printfabonacci ( int i){ if(i==0){ return 0; } if(i==1 || i==2){ return 1; } // calling method recursively else{ return printfabonacci (i-1)+ printfabonacci (i-2); } } public static void main(String args[]){ int maxnumbers=10; // max numbers in Fibonacci String str=""; for(int i=0; i<maxnumbers str="str" printfabonacci system.out.println series of numbers is> <p><strong> Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172500160023570.png?x-oss-process=image/resize,p_40" class="lazy" alt="What is Java?" ></p> <h3 id="Conclusion">Conclusion</h3> <p>From the above discussion, we have a clear understanding of the features and different applications. Also, we have seen some code examples showing the creation of the java class, declaration of the main method, creation of methods, object creation, and calling java methods through the object.</p></maxnumbers>
The above is the detailed content of What is Java?. For more information, please follow other related articles on the PHP Chinese website!
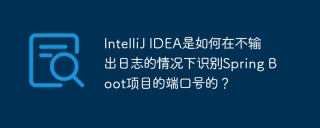
Start Spring using IntelliJIDEAUltimate version...
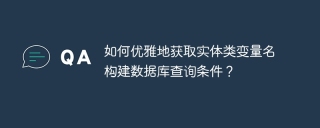
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
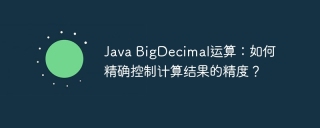
Java...
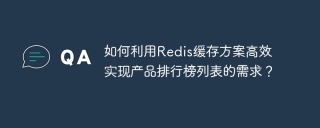
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
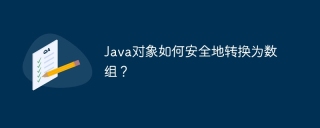
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
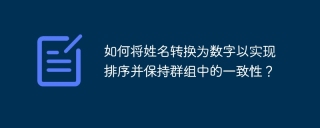
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
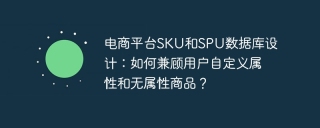
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
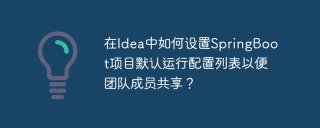
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
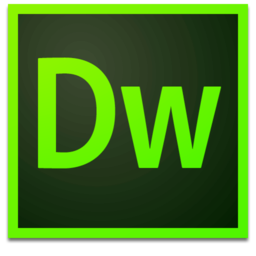
Dreamweaver Mac version
Visual web development tools
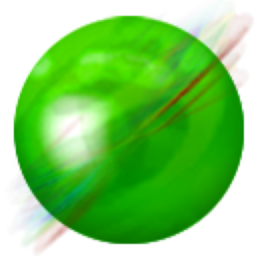
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software