PHP Loops are a type of code that can help us to run some code inside of the loop to run over and over again according to our requirement as the input, and these loops will help run the code and complete the task infinitely as we want to execute the same code inside of the loop again and again until our condition becomes false or else the code runs continuously. The word says that it will be repeated only if a certain condition is true, which is mentioned in the loop parameters to check the condition for the PHP loop/loops.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Different Loops of PHP
Like the other programming languages, PHP offers different loop concepts. They are: WHILE LOOP, DO WHILE LOOP, FOR LOOP, FOREACH LOOP. You will get a detailed explanation of each and every loop concept of PHP below.
1. While loop
While loop will run the specific/ some block of code inside the while loop parenthesis of PHP only if the condition mentioned in the loop is true; if condition is false, the While Loop will break the code, which is in the continuous process of running the code.
Syntax:
While(Condition to check){ //Code which is need to executed or the code statements which is to run }
Explanation:
In the above syntax, a while loop is mentioned with the condition inside of the parenthesis to run statements inside of the loop only if the condition mentioned is True, or else the code inside of the loop will not run by breaking the loop get out of the loop/while loop.
Example:
The example below consists of while loop programming to print the list of numbers from 1 to 10. In the example below, variable 1 is assigned with the number 1, and then the loop program starts with the help of the $i variable value and the while loop. While loop started with the condition i
Code:
<?php $i = 1; while($i <= 10){ echo " Now The number is " . $i . "<br>"; $i=$i+1; } ?>
Output:
2. Do While Loop
The Do While loop executes the programming code inside the loop first and then checks the loop condition, while the While Loop checks the loop condition before running the code inside the loop.
Syntax:
do{ //Programming statements which is need to be executed only if the loop condition is true } While(condition to check);
Example:
The below program contains 2 do while programs to print the list of even numbers between 1-10 and the list of odd numbers between 1-10. The program also prints the sum of odd numbers, even numbers, and the sum of all the natural numbers between 1-10. The first do-while loop checks the value of the variable $i to see if it is completely divisible by the value “2”. If True, then the value will be printed, and the $k value will store the $i value; else, nothing happens, just the incrementation of the $i variable value.
Likewise, the loop continues until the $i value reaches the value “10”. Like that, others do while loop also runs by checking whether the $j value will not be divided by 2 values. If True, the $j value will be printed, and the $m will store the value. Finally, store the sum of even numbers in the variable $k and store the sum of odd numbers in the variable $l. Also, store the total sum of all natural numbers in the variable $m. Display these values in the output, as shown in the picture.
Code:
<?php $i = 1; echo "List of Even Numbers between 1-10:: "; $k = 0; $m = 0; do{ if($i%2==0){ echo " $i " ." , "; $k=$k+$i; } $m=$m+$i; $i=$i+1; }while($i <= 10); echo "<br>"." Sum of the total even numbers between 1-10 ::"." $k"; echo "<br>"; $j = 1; $l = 0; echo "List of the ODD Numbers between 1-10:: "; do{ if($j%2!=0){ echo " $j " ." , "; $l=$l+$j; } $j=$j+1; }while($j "." Sum of the total odd numbers between 1-10 ::"." $l"; echo "<br>"; echo "<br>"." Sum of the total natural numbers between 1-10 ::"." $m"; echo "<br>"; ?>
Output:
3. For loop
For loop is somewhat different when comparing the While Loop and the Do While Loop. The code will execute repeatedly if it meets a specific condition. The loop executes the code multiple times based on a specified condition.
For loop will have 3 parameters. They are initialization, condition, and the incrementation value inside the For Loop parenthesis.
Syntax:
for(initialization value; condition value; incrementing value){ //Programming code statements which is need to be executed when condition of the loop becomes TRUE }
Parameters of the For Loop:
- Initialization: In For Loop, this is the value/variable value to start the program.
- Condition: In For Loop, this is the value/variable value that must be checked. If the condition becomes true, then the program statements will run continuously by checking the condition.
- Incrementing/Incrementation: The program will increment the initial or running value of the program statements by 1 or another value as required, depending on our needs, in a For loop.
Example:
The below for loop example will print the list of the natural numbers between 1-30 and the sum of all the values between 1-30.
To begin, we set $i as 1 for the initial value. The condition is that $i should be less than or equal to 30, with an increment of $i+1. For loop will print the $i value until the i value becomes 30, and the $j variable’s value will store all the numbers/values of the $i variable and then sum them one by one in the loop until the I value reaches 30. After printing all the natural numbers using the For Loop, the sum of all natural numbers between 1-30 will be displayed.
Code:
<?php echo "List of the Natural Numbers between 1-30 :: "; $j=0; for($i=1; $i<=30; $i++){ echo "$i" . " , "; $j=$j+$i; } echo "<br>"; echo "Sum of all the natural numbers between 1-30 :: "; echo "$j"; echo "<br>"; ?>
Output:
4. Foreach Loop
PHP uses the “foreach” loop concept to iterate over arrays or multiple arrays.
Syntax:
foreach($array as $value){ //Programming code which is need to be executed }
Example:
The below example will print the values from the $colors1 variable. $colors1 variable values are the list of the colors. Using the foreach loop concept will print the colors in the array individually.
Code:
<?php $colors1 = array("Yellow", "Red", "Blue", "Green",); // Colors array accessing in the loop foreach($colors1 as $value1){ echo $value1 . "<br>"; } ?>
Output:
The above is the detailed content of PHP Loops. For more information, please follow other related articles on the PHP Chinese website!
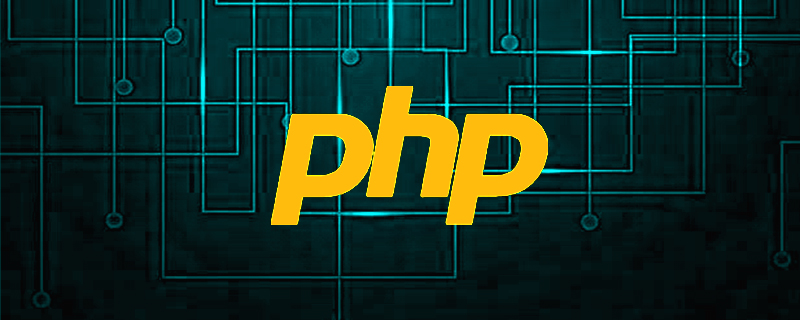
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
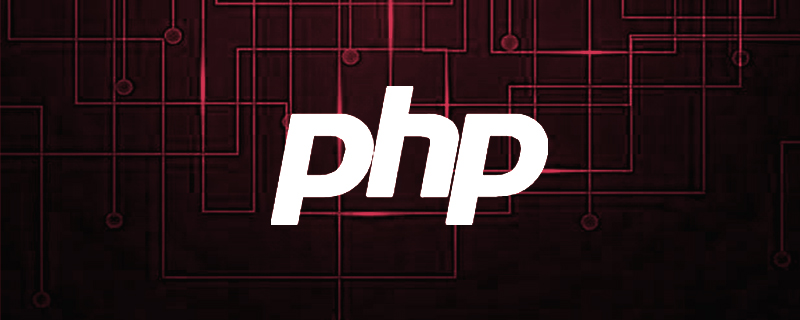
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
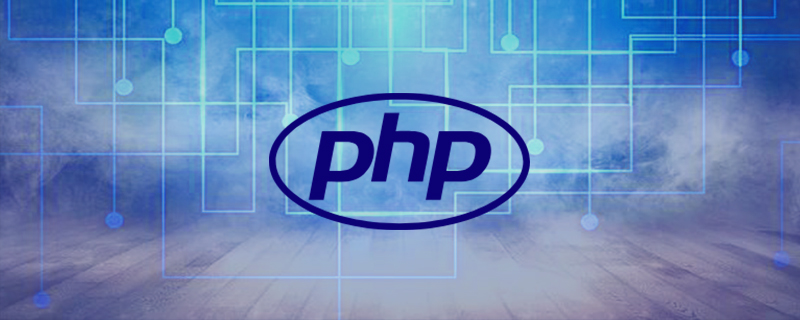
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
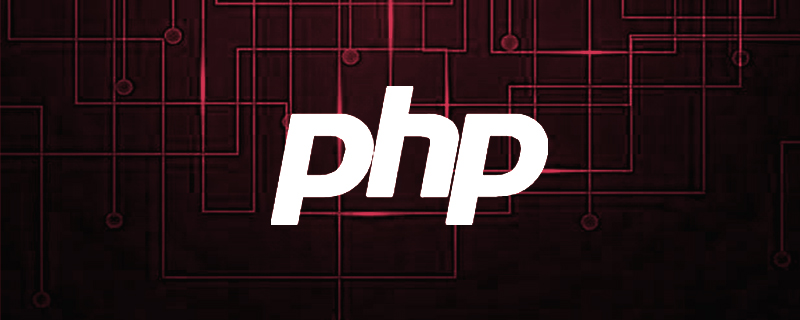
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
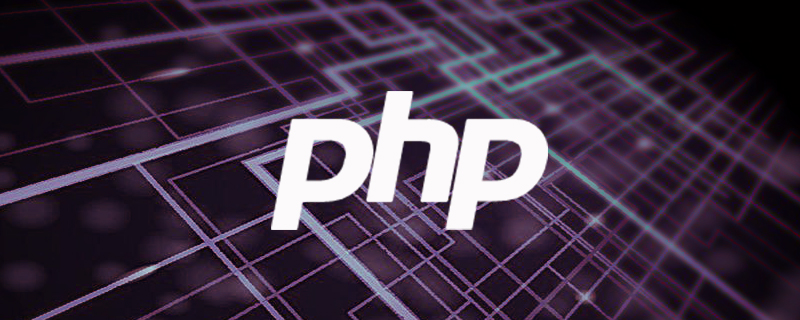
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
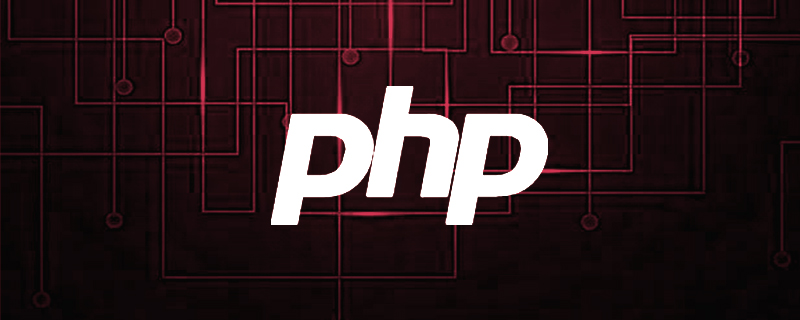
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
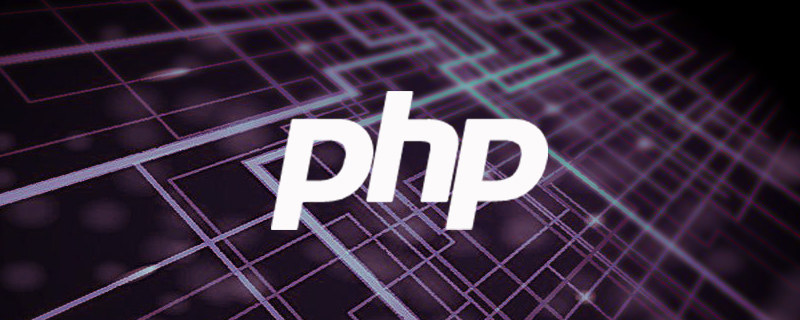
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
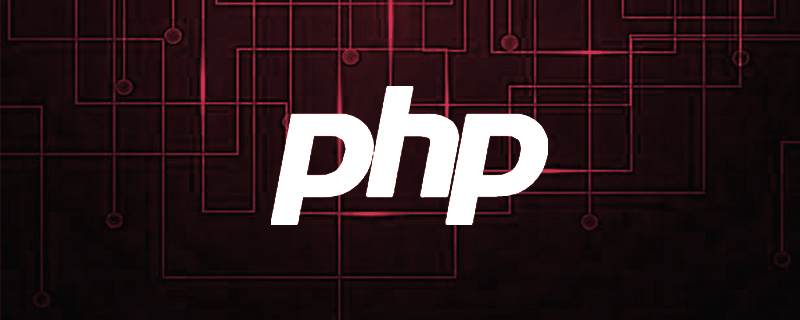
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
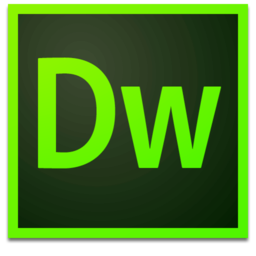
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
