Below are three main User Interface (UI) options that create webapps using JavaScript.
The code below shows how to insert inputs in the Document Object Model (DOM) via HTML and the corresponding JavaScript to obtain selection information.
Radio button
// Radio button selection <!-- Radio button menu: put in body -->// Put in <script> async function processEvent_radioOption0(event) { if (this.getAttribute("checked") == false) { document.getElementById("text_input0").style.display = "none"; document.getElementById("text_input1").style.display = "none"; } else { document.getElementById("text_input0").style.display = "block"; document.getElementById("text_input1").style.display = "block"; } } async function processEvent_radioOption1(event) { if (this.getAttribute("checked") == false) { document.getElementById("text_input0").style.display = "none"; document.getElementById("text_input1").style.display = "none"; } else { document.getElementById("text_input0").style.display = "none"; document.getElementById("text_input1").style.display = "none"; } } document.getElementById("radioOption0").addEventListener("click", processEvent_radioOption0, false); document.getElementById("radioOption1").addEventListener("click", processEvent_radioOption1, false); // Put in a function in <script> const radioOption0 = document.getElementById("radioOption0").checked; const radioOption1 = document.getElementById("radioOption1").checked; if (radioOption0 == false && radioOption1 == false) { document.getElementById('notification').innerHTML = "Please select radioOption0 or radioOption1."; } if (radioOption0 == true && radioOption1 == false) { console.log('radioOption0'); } if (radioOption0 == false && radioOption1 == true) { console.log('radioOption1'); } <p><strong>Drop down<br> <pre class="brush:php;toolbar:false">// Drop down selection <!-- Drop down menu: put in body --> <label for="select_dropdown_option_label">Select a drop down option: <select name="dropdown_options" id="dropdown_options" style="display:block"> <option value="---">Select an option <option value="drop_down_option0">drop_down_option0 <option value="drop_down_option1">drop_down_option1 <option value="drop_down_option2">drop_down_option2 // Put in <script> async function processEvent_dropdown_options(event) { // there is nothing in event that tells which drop down was selected // console.log('event: ', event); const element = document.getElementById("dropdown_options"); console.log('element.selectedIndex: ', element.selectedIndex); // OR // console.log('element.options.selectedIndex: ', element.options.selectedIndex); if (document.getElementById("dropdown_options").selectedIndex == 1) { document.getElementById("text_input").style.display = 'block'; } else { document.getElementById("text_input").style.display = 'none'; } } document.getElementById("dropdown_options").addEventListener("change", processEvent_dropdown_options, false); // Put in a function in <script> var dropdown_option_type = document.getElementById("dropdown_options").value; if (dropdown_option_type == 'drop_down_option0') { } else if (dropdown_option_type == 'drop_down_option1') { } else if (dropdown_option_type == 'drop_down_option2') { } else { document.getElementById('notification').innerHTML = "Please select a drop down option type."; } <p><strong>Text input<br> <pre class="brush:php;toolbar:false"><!-- Text input: put in body --> <input type="text" name="text_input0" id="text_input0" value="" style="display:block;"> <button id="button0" onclick="button0_task()" style="display:none;">button0 // Put in <script> async function processEvent_text_input(event) { // Make something change when text is typed into the text input if (document.getElementById("text_input0").value.length > 0) { // If something is typed into the text input box // Make a button appear document.getElementById("button0").style.display = 'block'; } else { document.getElementById("button0").style.display = 'none'; } } document.getElementById("text_input0").addEventListener("change", processEvent_text_input, false); // Put in a function in <script> async function button0_task() { var text_input0 = document.getElementById("text_input0").value; <p>Happy Practicing! ? </script>
The above is the detailed content of EventListener UI in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
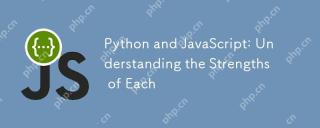
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
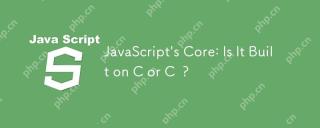
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
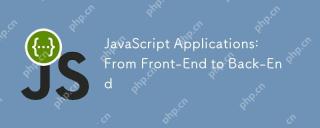
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
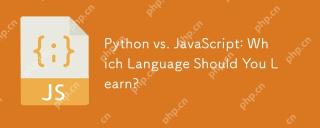
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
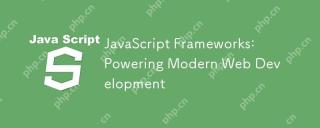
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
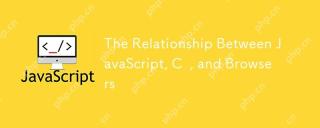
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
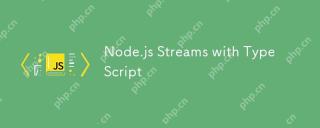
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
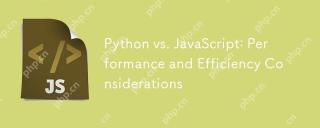
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
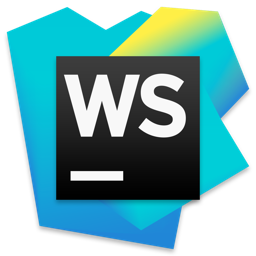
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
