JSON WEB TOKEN (JWT)
JWT (JSON Web Token) is a method for securely transmitting information between two parties (such as a client and a server) as a JSON object. It's designed to be compact and URL-safe, making it easy to pass around in URLs, headers.
- Header
- Payload
- Signature
Header
The header typically consist two parts: the type of the token (JWT) and the signing algorithm being used, such as HMAC SHA256 or RSA.
{
"alg":"HS256",
"typ":"JWT"
}
Payload
This is where the actual data is stored. It can include information like the user ID, roles, expiration time, and other claims (data about the user or session).
{
"email":"ayushstwt@gmail.com",
"name":"Ayush"
}
Signature
Ensures the integrity of the token. This is a security feature that ensures the token hasn’t been altered. It’s created by combining the encoded header and payload with a secret key using the specified algorithm. The signature helps the server verify that the token is legitimate and hasn’t been tampered with.
The benefits of JWT
No Need to Repeatedly Send Credentials: With JWT, you don't have to send your username and password with every request. Instead, you log in once, and the server gives you a token. You then send this token with each request to prove your identity, making the process more secure and efficient.
Built-in Expiration: Each JWT comes with an expiration time, meaning it’s only valid for a specific period. This reduces the risk of long-term misuse if a token is somehow intercepted. After it expires, the user needs to log in again to get a new token, adding an extra layer of security.
JWT with Spring Boot securely manages user authentication by issuing tokens after login. These tokens are sent with each request, ensuring secure, stateless communication without repeatedly sending credentials.
Stateless communication means the server doesn't remember past requests. Each request carries everything needed (like a JWT), so the server doesn't store session info.
Implementing JWT in a Java Spring Boot application involves several steps. Here's a simplified outline to get you started:
1. Add Dependencies
Include the necessary dependencies in your pom.xml file
<dependency> <groupid>io.jsonwebtoken</groupid> <artifactid>jjwt-api</artifactid> <version>0.12.5</version> </dependency> <dependency> <groupid>io.jsonwebtoken</groupid> <artifactid>jjwt-impl</artifactid> <version>0.12.5</version> <scope>runtime</scope> </dependency> <dependency> <groupid>io.jsonwebtoken</groupid> <artifactid>jjwt-jackson</artifactid> <version>0.12.5</version> <scope>runtime</scope> </dependency>
All the dependencies that we need to create the spring-boot application with JWT
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemalocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelversion>4.0.0</modelversion> <parent> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-parent</artifactid> <version>3.3.3</version> <relativepath></relativepath> <!-- lookup parent from repository --> </parent> <groupid>com.tier3Hub</groupid> <artifactid>user-auth-service</artifactid> <version>0.0.1-SNAPSHOT</version> <name>user-auth-service</name> <description>The user-auth-service is a microservice responsible for handling user authentication and authorization within a distributed system. It is designed to manage user login, registration, and secure access to various services using robust security practices. This service implements authentication mechanisms like JSON Web Tokens (JWT) and integrates with OAuth 2.0 for third-party authentication. Built with Spring Boot, it ensures scalability, reliability, and easy integration with other microservices in the system.</description> <url></url> <licenses> <license></license> </licenses> <developers> <developer></developer> </developers> <scm> <connection></connection> <developerconnection></developerconnection> <tag></tag> <url></url> </scm> <properties> <java.version>21</java.version> </properties> <dependencies> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-actuator</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-data-jpa</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-security</artifactid> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-web</artifactid> </dependency> <dependency> <groupid>io.jsonwebtoken</groupid> <artifactid>jjwt-api</artifactid> <version>0.12.5</version> </dependency> <dependency> <groupid>io.jsonwebtoken</groupid> <artifactid>jjwt-impl</artifactid> <version>0.12.5</version> <scope>runtime</scope> </dependency> <dependency> <groupid>io.jsonwebtoken</groupid> <artifactid>jjwt-jackson</artifactid> <version>0.12.5</version> <scope>runtime</scope> </dependency> <dependency> <groupid>com.mysql</groupid> <artifactid>mysql-connector-j</artifactid> <scope>runtime</scope> </dependency> <dependency> <groupid>org.projectlombok</groupid> <artifactid>lombok</artifactid> <optional>true</optional> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-test</artifactid> <scope>test</scope> </dependency> <dependency> <groupid>org.springframework.security</groupid> <artifactid>spring-security-test</artifactid> <scope>test</scope> </dependency> <dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-validation</artifactid> </dependency> <dependency> <groupid>org.springdoc</groupid> <artifactid>springdoc-openapi-starter-webmvc-ui</artifactid> <version>2.5.0</version> </dependency> <dependency> <groupid>org.modelmapper</groupid> <artifactid>modelmapper</artifactid> <version>3.1.1</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-maven-plugin</artifactid> <configuration> <excludes> <exclude> <groupid>org.projectlombok</groupid> <artifactid>lombok</artifactid> </exclude> </excludes> </configuration> </plugin> </plugins> </build> </project>
we are using different types of dependencies like
- Spring Boot Starter Actuator: 3.3.3 - Adds production-ready features like monitoring and health checks.
- Spring Boot Starter Data JPA: 3.3.3 - Simplifies database interactions with JPA support.
- Spring Boot Starter Security: 3.3.3 - Provides security features like authentication and authorization.
- Spring Boot Starter Web: 3.3.3 - Supports building web applications, including RESTful services.
- JJWT API: 0.12.5 - Handles JWT creation and parsing for secure token management.
- JJWT Impl: 0.12.5 - Implements core JWT functionalities.
- JJWT Jackson: 0.12.5 - Enables JWT JSON parsing using Jackson.
- MySQL Connector: Runtime - Connects your application to a MySQL database.
- Lombok: Not specified - Reduces boilerplate code with annotations.
- Spring Boot Starter Test: 3.3.3 - Provides testing support for Spring Boot applications.
- Spring Security Test: 3.3.3 - Helps with testing security configurations.
- Spring Boot Starter Validation: 3.3.3 - Adds validation support for request and response objects.
- SpringDoc OpenAPI Starter WebMVC UI: 2.5.0 - Integrates Swagger UI for API documentation.
- ModelMapper: 3.1.1 - Simplifies object mapping between different layers.
*2. Project structure *
3. Add the configuration in application.properties file
spring.application.name=user-auth-service server.port=8000 spring.datasource.url=jdbc:mysql://localhost:3306/auth_services spring.datasource.username=root spring.datasource.password=ayush@123 spring.jpa.hibernate.ddl-auto=update spring.jpa.show-sql=true #debug logs logging.level.org.springframework.security=debug spring.main.allow-circular-references=true
4. Create the USER entity
package com.tier3Hub.user_auth_service.entity; import jakarta.persistence.*; import lombok.AllArgsConstructor; import lombok.Builder; import lombok.Data; import lombok.NoArgsConstructor; import java.time.LocalDateTime; import java.util.List; @AllArgsConstructor @NoArgsConstructor @Data @Builder @Table @Entity(name = "User") public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String username; private String password; private String email; private String phoneNumber; private List<string> roles; private LocalDateTime createdAt; private LocalDateTime updatedAt; } </string>
** 5. create the service and repository class and interface**
Repository.java
package com.tier3Hub.user_auth_service.Repository; import com.tier3Hub.user_auth_service.entity.User; import org.springframework.data.jpa.repository.JpaRepository; public interface AuthRepository extends JpaRepository<user long> { User findByUsername(String username); } </user>
service.java
package com.tier3Hub.user_auth_service.service; import com.tier3Hub.user_auth_service.dto.LoginResponse; import com.tier3Hub.user_auth_service.dto.RegisterDTO; import com.tier3Hub.user_auth_service.dto.RegisterResponse; public interface AuthService { RegisterResponse register(RegisterDTO registerDTO); }
6. create the DTO's for login and signin request and response
CreateloginDTO.java
package com.tier3Hub.user_auth_service.dto; import jakarta.validation.constraints.NotBlank; import jakarta.validation.constraints.Size; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @AllArgsConstructor @NoArgsConstructor @Data public class LoginDTO { @NotBlank(message = "Username is required") @Size(min = 3, max = 20, message = "Username must be between 3 and 20 characters") private String username; @NotBlank(message = "Password is required") private String password; }
loginResponse.java
package com.tier3Hub.user_auth_service.dto; import lombok.AllArgsConstructor; import lombok.Builder; import lombok.Data; import lombok.NoArgsConstructor; @Data @AllArgsConstructor @NoArgsConstructor @Builder public class LoginResponse { private String accessToken; private String tokenType = "Bearer"; }
RegisterDTO.java
package com.tier3Hub.user_auth_service.dto; import jakarta.validation.constraints.Email; import jakarta.validation.constraints.NotBlank; import jakarta.validation.constraints.Size; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @AllArgsConstructor @NoArgsConstructor @Data public class RegisterDTO { @NotBlank(message = "Username is required") @Size(min = 3, max = 20, message = "Username must be between 3 and 20 characters") private String username; @NotBlank(message = "Password is required") @Size(min = 8, message = "Password must be at least 8 characters") private String password; @NotBlank(message = "Email is required") @Email(message = "Email should be valid") private String email; }
RegisterResponse.java
package com.tier3Hub.user_auth_service.dto; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; import java.time.LocalDateTime; @AllArgsConstructor @NoArgsConstructor @Data public class RegisterResponse { private Long id; private String username; private String email; private LocalDateTime createdAt; private LocalDateTime updatedAt; }
*7. for sending custom response from the API we use the ResponseHandler.java *
package com.tier3Hub.user_auth_service.utils; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import java.util.HashMap; import java.util.Map; public class ResponseHandler { public static ResponseEntity<object> generateResponse(String message, HttpStatus status, Object responseObj) { Map<string object> map = new HashMap<string object>(); map.put("message", message); map.put("status", status.value()); map.put("data", responseObj); return new ResponseEntity<object>(map, status); } } </object></string></string></object>
8. for storing some constants we create the class inside the utils package that is ApplicationConstants.java
package com.tier3Hub.user_auth_service.utils; public class AppConstants { public static final String[] PUBLIC_URLS = { "/v3/api-docs/**", "/swagger-ui/**", "/api/auth/register/**", "/api/auth/login/**","/api/auth/registerAdmin/**" }; }
9. for converting the object one to another we use the dependency that is model mapper for configuration that we create the class inside the config package that is ApplicationConfigs.java
package com.tier3Hub.user_auth_service.config; import org.modelmapper.ModelMapper; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class ApplicationConfigs { @Bean public ModelMapper modelMapper() { return new ModelMapper(); } }
**
This is the basic setup that we do for every spring-boot application we create now securing the rest endpoint with JWT we started.
**
now inside the security package we create the class called JWTFilter.java
The JWTFilter is a custom Spring Security filter that intercepts HTTP requests to validate JWTs. It checks for the "Authorization" header, extracts the token, and retrieves the username. If the token is valid, it creates an authentication token with user details and sets it in the security context, allowing the application to recognize the authenticated user for further processing.
package com.tier3Hub.user_auth_service.security; import jakarta.servlet.FilterChain; import jakarta.servlet.ServletException; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.security.authentication.UsernamePasswordAuthenticationToken; import org.springframework.security.core.context.SecurityContextHolder; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.web.authentication.WebAuthenticationDetailsSource; import org.springframework.stereotype.Service; import org.springframework.web.filter.OncePerRequestFilter; import java.io.IOException; @Service public class JWTFilter extends OncePerRequestFilter { @Autowired private UserDetailsService userDetailsService; @Autowired private JWTUtil jwtUtil; @Override protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws ServletException, IOException { String authorizationHeader = request.getHeader("Authorization"); String username = null; String jwt = null; if (authorizationHeader != null && authorizationHeader.startsWith("Bearer ")) { jwt = authorizationHeader.substring(7); username = jwtUtil.extractUsername(jwt); } if (username != null) { UserDetails userDetails = userDetailsService.loadUserByUsername(username); if (jwtUtil.validateToken(jwt)) { UsernamePasswordAuthenticationToken auth = new UsernamePasswordAuthenticationToken(userDetails, null, userDetails.getAuthorities()); auth.setDetails(new WebAuthenticationDetailsSource().buildDetails(request)); SecurityContextHolder.getContext().setAuthentication(auth); } } chain.doFilter(request, response); } }
create the class JWTUtil.java
The JWTUtil class manages JWT operations, including extracting usernames and expiration dates from tokens. It generates new tokens using a secret key and validates existing tokens by checking their expiration. The class uses HMAC for signing and includes methods to parse claims and determine if tokens are expired, ensuring secure authentication and authorization in the application.
package com.tier3Hub.user_auth_service.security; import io.jsonwebtoken.Claims; import io.jsonwebtoken.Jwts; import io.jsonwebtoken.security.Keys; import org.springframework.stereotype.Service; import javax.crypto.SecretKey; import java.util.Date; import java.util.HashMap; import java.util.Map; @Service public class JWTUtil { private String SECRET_KEY = "TaK+HaV^uvCHEFsEVfypW#7g9^k*Z8$V"; private SecretKey getSigningKey() { return Keys.hmacShaKeyFor(SECRET_KEY.getBytes()); } public String extractUsername(String token) { Claims claims = extractAllClaims(token); return claims.getSubject(); } public Date extractExpiration(String token) { return extractAllClaims(token).getExpiration(); } private Claims extractAllClaims(String token) { return Jwts.parser() .verifyWith(getSigningKey()) .build() .parseSignedClaims(token) .getPayload(); } private Boolean isTokenExpired(String token) { return extractExpiration(token).before(new Date()); } public String generateToken(String username) { Map<string object> claims = new HashMap(); return createToken(claims, username); } private String createToken(Map<string object> claims, String subject) { return Jwts.builder() .claims(claims) .subject(subject) .header().empty().add("typ","JWT") .and() .issuedAt(new Date(System.currentTimeMillis())) .expiration(new Date(System.currentTimeMillis() + 1000 * 60 * 50)) // 5 minutes expiration time .signWith(getSigningKey()) .compact(); } public Boolean validateToken(String token) { return !isTokenExpired(token); } } </string></string>
*configure the Spring security and add some modifictaion we create the class SecurityConfig.java *
The SecurityConfig class sets up security for the application using Spring Security. It defines access rules, allowing public endpoints while restricting others based on user roles. The class incorporates a JWT filter to validate tokens and uses BCrypt for password encoding. It also configures an authentication manager with a custom user details service for secure user authentication.
package com.tier3Hub.user_auth_service.config; import com.tier3Hub.user_auth_service.security.JWTFilter; import com.tier3Hub.user_auth_service.service.UserInfoConfigManager; import com.tier3Hub.user_auth_service.utils.AppConstants; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.authentication.AuthenticationManager; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.config.annotation.authentication.configuration.AuthenticationConfiguration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configurers.AbstractHttpConfigurer; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; import org.springframework.security.crypto.password.PasswordEncoder; import org.springframework.security.web.SecurityFilterChain; import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter; @Configuration @EnableWebSecurity public class SecurityConfig { @Autowired private JWTFilter jwtFilter; @Autowired private UserInfoConfigManager userInfoConfigManager; @Bean public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception { return http.authorizeHttpRequests(request -> request .requestMatchers(AppConstants.PUBLIC_URLS).permitAll() .requestMatchers("/api/test/public/hello/**").hasAnyRole("USER","ADMIN") .requestMatchers("/api/test/private/**").hasRole("ADMIN") .anyRequest() .authenticated()) .csrf(AbstractHttpConfigurer::disable) .addFilterBefore(jwtFilter, UsernamePasswordAuthenticationFilter.class) .build(); } @Autowired public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception { auth.userDetailsService(userInfoConfigManager).passwordEncoder(passwordEncoder()); } @Bean public PasswordEncoder passwordEncoder() { return new BCryptPasswordEncoder(); } @Bean public AuthenticationManager authenticationManager(AuthenticationConfiguration auth) throws Exception { return auth.getAuthenticationManager(); } }
The securityFilterChain method configures access rules for different API endpoints in the Spring application. It permits public URLs and applies role-based access control for user and admin roles. Role-based authentication restricts resource access based on user roles (e.g., USER, ADMIN). In Spring Boot, you define roles and configure security settings in the SecurityConfig class to specify access permissions. During user registration, assign roles, and use annotations like @PreAuthorize to enforce role checks in controllers. This approach enhances security, allows easy permission management, and simplifies user access rights as the application scales. Implementing role-based auth provides flexibility and maintainability for your user management system. CSRF protection is disabled, and a custom JWT filter is added to authenticate requests based on JSON Web Tokens, ensuring secure and controlled access to resources.
configureGlobal method handle configures global authentication settings in a Spring application. It uses a custom user details service for loading user data and a BCrypt password encoder for secure password hashing. Additionally, it provides an AuthenticationManager bean for handling authentication processes, ensuring a secure and efficient user authentication system that leverages strong password management practices.
create the endpoints for register and login
package com.tier3Hub.user_auth_service.Controller; import com.tier3Hub.user_auth_service.dto.LoginDTO; import com.tier3Hub.user_auth_service.dto.LoginResponse; import com.tier3Hub.user_auth_service.dto.RegisterDTO; import com.tier3Hub.user_auth_service.security.JWTUtil; import com.tier3Hub.user_auth_service.service.AuthService; import com.tier3Hub.user_auth_service.service.UserInfoConfigManager; import com.tier3Hub.user_auth_service.utils.ResponseHandler; import jakarta.validation.Valid; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.security.authentication.AuthenticationManager; import org.springframework.security.authentication.UsernamePasswordAuthenticationToken; import org.springframework.security.core.Authentication; import org.springframework.security.core.context.SecurityContext; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController @RequestMapping("/api/auth") public class AuthController { @Autowired JWTUtil jwtUtil; @Autowired AuthService authService; @Autowired AuthenticationManager authenticationManager; @Autowired private UserInfoConfigManager userInfoConfigManager; @PostMapping("/register") public ResponseEntity<object> register(@Valid @RequestBody RegisterDTO registerDTO) { return ResponseHandler.generateResponse("User registered successfully", HttpStatus.OK, authService.register(registerDTO)); } @PostMapping("/login") public ResponseEntity<object> login(@Valid @RequestBody LoginDTO loginDTO) { try { Authentication authenticate = authenticationManager .authenticate(new UsernamePasswordAuthenticationToken(loginDTO.getUsername(), loginDTO.getPassword())); UserDetails userDetails = userInfoConfigManager.loadUserByUsername(loginDTO.getUsername()); String jwt = jwtUtil.generateToken(userDetails.getUsername()); LoginResponse loginResponse = LoginResponse .builder() .accessToken(jwt) .build(); return ResponseHandler.generateResponse("User logged in successfully", HttpStatus.OK, loginResponse); } catch (Exception e) { return new ResponseEntity("Incorrect username or password", HttpStatus.BAD_REQUEST); } } } </object></object>
This login method in the AuthController handles user login requests. It takes a LoginDTO containing the username and password, validates them, and attempts authentication using the AuthenticationManager. Upon successful authentication, it retrieves user details and generates a JWT token using the JWTUtil class. The token is then included in a LoginResponse object and returned with a success message. If authentication fails, it catches the exception and returns a "Incorrect username or password" response with a 400 status code.
generateToken(String username): This method creates an empty claims map and calls the createToken method with the username as the subject. It serves as the entry point for token generation.
c*reateToken(Map claims, String subject):* This method builds the JWT using the Jwts.builder(). It sets the claims, subject, and token metadata, such as issue date and expiration time (set to 5 minutes). The token is then signed with a secret key and compacted into a string format for transmission.
Testing
now we run the application
and hit the URL here our application is runing on 8000 port
http://localhost:8000/swagger-ui/index.html
Using Swagger in your project enhances API documentation and testing. It provides a user-friendly interface for developers to explore your APIs, understand request/response structures, and test endpoints directly from the documentation. By integrating Swagger, you enable automatic generation of API docs based on your code annotations, making it easier for both front-end and back-end developers to collaborate efficiently.
first we register the user
we get the response like this
after that we login the user
we get the response like this
Conclusion
The project implements role-based authentication using JWT (JSON Web Tokens) in a Spring Boot application. It features a secure authentication mechanism where users can register and log in, receiving a JWT that grants access based on their assigned roles (like USER or ADMIN). The SecurityConfig class configures access permissions, ensuring that public endpoints are accessible to everyone while restricting sensitive operations to authorized users only. The JWTUtil class handles token creation, validation, and user extraction. Overall, this setup enhances security, enabling seamless and robust access control across the application.
The project employs a comprehensive security framework that leverages Spring Security for user authentication and authorization. The AuthController facilitates user registration and login, generating a JWT upon successful authentication. The application uses a JWTFilter to intercept requests and validate tokens, ensuring that only authenticated users can access protected resources. By integrating role-based access control, the project provides a flexible and secure user management system. This design not only improves security but also enhances user experience by minimizing the need for repeated logins. Overall, it lays a solid foundation for building scalable and secure microservices.
You can explore the complete source code for the User Authentication Service on my GitHub repository. This project showcases various features such as user registration, login, and secure access using JWT for authentication. Feel free to check it out, contribute, or use it as a reference for your own projects!
GitHub Repository: https://github.com/ishrivasayush/user-auth-service
For those interested in diving deeper into JSON Web Tokens (JWT), I recommend visiting jwt.io. This resource provides comprehensive information about JWT, including how it works, its structure, and practical examples. It's an excellent starting point for understanding token-based authentication and authorization, which are essential for modern web applications. Whether you're a beginner or looking to refresh your knowledge, jwt.io offers valuable insights into securely managing user sessions.
The above is the detailed content of Securing Microservices with Spring Security: Implementing JWT. For more information, please follow other related articles on the PHP Chinese website!
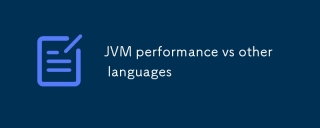
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
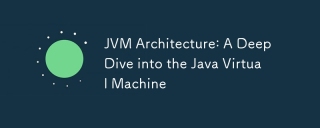
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
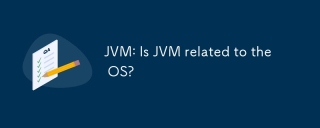
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
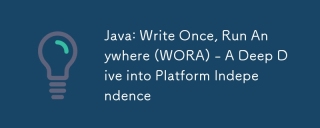
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
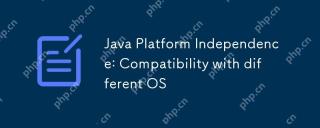
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
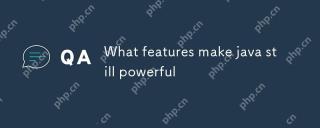
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
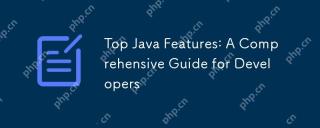
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
