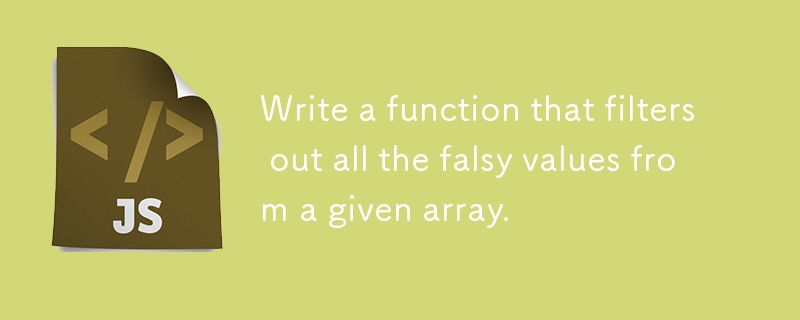
const removeFalsyValues = (arr) => {
let truthy = []
for(let i = 0; i < arr.length; i++){
if(arr[i]) {
truthy.push(arr[i])
}
}
return truthy;
}
console.log('removeFalsyValues:', removeFalsyValues([0, 1, false, 2, "", 3, undefined, NaN, null]))
Falsy values in JavaScript are values that are considered false when evaluated in a Boolean context. These include0, false, "" (an empty string), undefined, NaN, and null.
Here's how the function works:
- Initialize an empty array: The function starts by creating an empty array called truthy. This will be used to store the values from the original array that are not falsy.
- Loop through the array: The function uses a for loop to go through each element in the input array arr.
- Check if the element is truthy: Inside the loop, there's an if statement that checks if the current element (arr[i]) is truthy. If the element is truthy (meaning it's not one of the falsy values), it gets added to the truthy array.
- Return the truthy array: After the loop has gone through all the elements, the function returns the truthy array, which now contains only the truthy values.
- The input array is [0, 1, false, 2, "", 3, undefined, NaN, null].
- The function will loop through each element and remove the falsy ones (0, false, "", undefined, NaN, null).
- The remaining truthy values (1, 2, 3) are returned in a new array:[1, 2, 3].
So, the output of this code will be:removeFalsyValue [1, 2, 3].
The above is the detailed content of Write a function that filters out all the falsy values from a given array.. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn