Hello, in this article, which seems like a tutorial, we will address a topic that in particular at first gave me a lot of headaches. However, this difficulty led me to study, study and study to make the abstraction of everyday life my own and thus bring to lines of code, the representation of something tangible (believe me, this can be a titanic task at times) . I became so passionate about the topic that I now share in this article important data to understand it, so let's get to the heart of the matter.
I will explain or try to do it in the best way possible, object-oriented programming or by its acronym (OOP) using JavaScript as my language of choice. To understand how to apply OOP to real-world situations, you have to internalize that object-oriented programming is not just a technique, it's an approach to life! In this article, we will explore fundamental OOP concepts and apply them to tangible examples from everyday life.
What is Object Oriented Programming?
Object-oriented programming is a programming paradigm that is based on the concept of "objects", you can imagine at this very moment literally an object of life: an apple, a dog, a house, a car, a rubber daddy. Now, visualize that these objects can contain data in the form of properties or characteristics and functionalities, that is, they can do things. Imagine that you are modeling a virtual world where each entity can be represented as an independent object with unique characteristics.
Objects in Real Life and in OOP
To better understand OOP, let's take a look at a real-life example: a car. A car can have properties such as model, color and speed, as well as methods such as starting and stopping. Transpolating this to the world of OOP would be quite simple:
class Auto { constructor(modelo, color) { this.modelo = modelo; this.color = color; this.velocidad = 0; } arrancar() { console.log(`El auto ${this.modelo} ha arrancado.`); } detener() { console.log(`El auto ${this.modelo} se ha detenido.`); this.velocidad = 0; } acelerar(kmh) { this.velocidad += kmh; console.log(`El auto ${this.modelo} acelera a ${this.velocidad} km/h.`); } } // Crear un objeto auto const miAuto = new Auto('Sedán', 'Rojo'); // Utilizar métodos del objeto auto miAuto.arrancar(); miAuto.acelerar(50); miAuto.detener();
In this example, we have created a class Auto with properties such as model, color and speed, as well as methods, that is, the things it can do: like start, stop and accelerate. Then, we create a myAuto object based on this class and use it to simulate real-life actions.
Inheritance: The Key to the Hierarchy
Imagine now that we want to model not only cars, but also motorcycles. They both share some similarities, but also have unique characteristics. This is where the concept of inheritance in OOP comes into play.
class Camioneta extends Auto { constructor(modelo, color, tipo) { super(modelo, color); this.tipo = tipo; } realizarTruco() { console.log(`La camioneta ${this.modelo} ${this.tipo} realiza un asombroso truco.`); } } // Crear un objeto camioneta const miCamioneta = new Camioneta('Explorer', 'Negra', '4x4'); // Utilizar métodos del objeto camioneta miCamioneta.arrancar(); miCamioneta.acelerar(80); miCamioneta.realizarTruco(); miCamioneta.detener();
Here, we have created a new Truck class that extends the Car class. The extends keyword allows us to inherit all the properties and methods of the parent class (Auto). Additionally, the child class (Pickup) can have additional properties and methods.
Encapsulation: Protecting your Secrets
Encapsulation is another pillar of OOP that allows us to protect the internal details of an object and expose only what is necessary. Let's look at a simple example using a "Bank Account":
class CuentaBancaria { constructor(titular, saldoInicial) { this.titular = titular; this._saldo = saldoInicial; // El saldo se designa con el prefijo _ para indicar que es privado } get saldo() { return this._saldo; } depositar(cantidad) { if (cantidad > 0) { this._saldo += cantidad; console.log(`${cantidad} depositados. Nuevo saldo: ${this._saldo}`); } else { console.log("Error: La cantidad debe ser mayor que cero."); } } retirar(cantidad) { if (cantidad > 0 && cantidad <p>In this example, we have encapsulated the account balance using a get method to access it. This protects the balance from being modified directly from outside the class, maintaining the integrity of our bank account.</p> <h3> Polymorphism: The Magic of Versatility </h3> <p>Polymorphism allows different classes to share the same method name, but with specific behaviors for each one. To illustrate this, let's imagine a zoo with animals that make sounds.<br> </p> <pre class="brush:php;toolbar:false"> class Animal { hacerSonido() { console.log('Algunos sonidos genéricos de animal.'); } } class Perro extends Animal { hacerSonido() { console.log('¡Guau! ¡Guau!'); } } class Gato extends Animal { hacerSonido() { console.log('¡Miau! ¡Miau!'); } } // Crear objetos animales const miAnimal = new Animal(); const miPerro = new Perro(); const miGato = new Gato(); // Utilizar el método hacerSonido de cada objeto miAnimal.hacerSonido(); miPerro.hacerSonido(); miGato.hacerSonido();
In this example, the Dog and Cat classes inherit from the Animal class, but each overrides the makeSound method with its own unique implementation. This allows different types of animals to use the same method differently.
Conclusion: OOP... put it into Action
I really appreciate you reaching this point! We explore key concepts such as objects, inheritance, encapsulation and polymorphism, and have applied them to real-life situations. Remember, OOP is a way of thinking that allows you to model and understand the world more effectively...and put it into code.
So the next time you see a car, a bank account, or even your pet, think about how you could represent them as objects in your code. Object-oriented programming is not only a powerful tool, it's a way to bring your programs to life!
I hope you have enjoyed this article and that you can take advantage of it in your projects. Leave me your comments to let me know what you thought and if you have any other real-life abstractions. ;)
The above is the detailed content of Object Oriented Programming - An abstraction of reality. For more information, please follow other related articles on the PHP Chinese website!
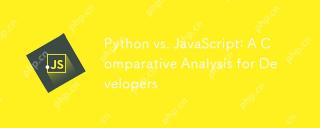
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
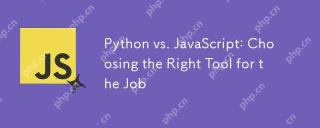
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
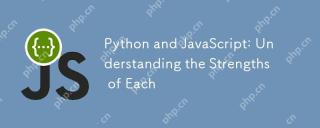
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
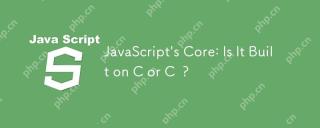
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
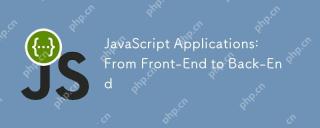
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
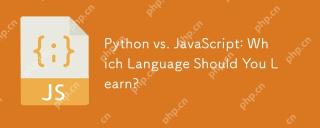
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
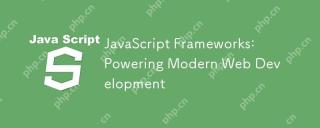
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
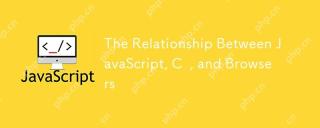
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
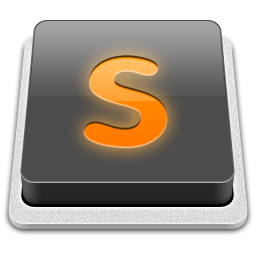
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
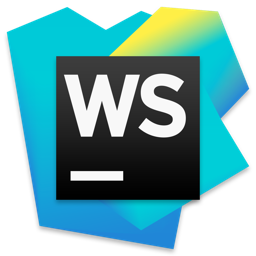
WebStorm Mac version
Useful JavaScript development tools
