Checkout the original post https://devaradise.com/date-in-javascript-dayjs-momentjs/ to read with Table of Content
Handling dates and times is a common task in JavaScript development. While JavaScript's native Date object offers a variety of methods to work with dates, there are scenarios where using external libraries like Day.js or Moment.js can simplify your workflow and provide more robust solutions.
In this article, we’ll explore common cases that can be solved by JavaScript’s native Date object and those that are best handled by external libraries. We’ll also compare Day.js and Moment.js to help you choose the right tool for your needs.
Common Cases Solvable by Native JS Date
The native JavaScript Date object provides a range of methods for working with dates and times. Here are some common use cases where the native Date object is sufficient:
1. Creating Dates
const now = new Date(); // Current date and time const specificDate = new Date('2024-07-16'); // Specific date
2. Getting Date and Time Components
const now = new Date(); const year = now.getFullYear(); const month = now.getMonth() + 1; // Months are zero-indexed const day = now.getDate(); const hours = now.getHours(); const minutes = now.getMinutes(); const seconds = now.getSeconds();
3. Setting Date and Time Components
const now = new Date(); now.setFullYear(2025); now.setMonth(11); // December now.setDate(25); now.setHours(10); now.setMinutes(11); now.setSeconds(12);
4. Date Arithmetic
const tomorrow = new Date(); tomorrow.setDate(tomorrow.getDate() + 1);
5. Formatting Dates
const now = new Date(); const dateString = now.toDateString(); // Example: 'Tue Jul 16 2024' const timeString = now.toTimeString(); // Example: '14:12:34 GMT+0200 (Central European Summer Time)'
6. Parsing Dates
const parsedDate = new Date(Date.parse('2024-07-16T14:12:34Z'));
7. Comparing Dates
const date1 = new Date('2024-07-16'); const date2 = new Date('2024-07-17'); const isSame = date1.getTime() === date2.getTime(); // false const isBefore = date1.getTime() <h3> 8. Getting the Day of the Week </h3> <pre class="brush:php;toolbar:false">const dayOfWeek = now.getDay(); // 0 for Sunday, 1 for Monday, etc.
9. Converting to UTC and ISO String
const now = new Date(); const isoDate = now.toISOString(); // 2024-07-22T11:30:59.827Z const utcDate = now.toUTCString(); // Mon, 22 Jul 2024 11:30:42 GMT
Calculating Date Differences
const date1 = new Date('2024-01-01'); const date2 = new Date('2024-12-31'); const differenceInTime = date2 - date1; const differenceInDays = differenceInTime / (1000 * 3600 * 24); console.log(differenceInDays);
Cases Best Solved by External Libraries Like Day.js and Moment.js
While the native Date object covers basic date manipulation, certain tasks are more efficiently handled by external libraries like Day.js and Moment.js. Here are some scenarios where these libraries excel:
1. Advanced Formatting
// Day.js const Day.jsDate = Day.js().format('YYYY-MM-DD HH:mm:ss'); // Moment.js const momentDate = moment().format('YYYY-MM-DD HH:mm:ss');
2. Relative Time
// Day.js Day.js.extend(relativeTime); // require RelativeTime plugin Day.js('2024-01-01').from(Day.js('2023-01-01')); // a year ago // Moment.js moment('2024-01-01').from(moment('2023-01-01')); // a year ago
3. Time Zone Handling
Day.js.extend(utc); Day.js.extend(timezone); Day.js('2024-07-16 14:12:34').tz('America/New_York'); // Moment.js with moment-timezone moment.tz('2024-07-16 14:12:34', 'America/New_York');
4. Internationalization (i18n)
// Day.js Day.js.locale('fr'); const frenchDate = Day.js().format('LLLL'); // dimanche 15 juillet 2012 11:01 // Moment.js moment.locale('fr'); const frenchMomentDate = moment().format('LLLL'); // dimanche 15 juillet 2012 11:01
5. Handling Invalid Dates
// Day.js const invalidDay.js = Day.js('invalid date'); if (!invalidDay.js.isValid()) { console.log('Invalid date'); } // Moment.js const invalidMoment = moment('invalid date'); if (!invalidMoment.isValid()) { console.log('Invalid date'); }
6. Parsing Complex Date Formats
// Day.js with customParseFormat plugin Day.js.extend(customParseFormat); const complexDate = Day.js('05/02/69 1:02:03 PM -05:00', 'MM/DD/YY H:mm:ss A Z'); // Moment.js const complexMoment = moment('05/02/69 1:02:03 PM -05:00', 'MM/DD/YY H:mm:ss A Z');
7. Working with Durations
// Day.js Day.js.extend(duration); const durationDay.js = Day.js.duration(5000); // 5 seconds console.log(durationDay.js.asMinutes()); // 0.083333... const humanizedDurationDay.js = Day.js.duration(5000).humanize(); // 'a few seconds' // Moment.js const durationMoment = moment.duration(5000); // 5 seconds console.log(durationMoment.asMinutes()); // 0.083333... const humanizedDurationMoment = moment.duration(5000).humanize(); // 'a few seconds'
Day.js vs Moment.js: A Detailed Comparison
Day.js and Moment.js are two popular libraries for date manipulation in JavaScript. While both serve the same purpose, they have distinct differences in terms of performance, features, and usability. This comparison will help you decide which library is better suited for your project.
Day.js
Pros
- Lightweight: Day.js is only 2KB in size, significantly smaller than Moment.js, which makes it ideal for performance-sensitive applications.
- Immutable: Day.js promotes immutability, meaning every operation returns a new instance, reducing bugs related to date manipulation.
- Plugin Architecture: Day.js has a modular plugin system, allowing you to include only the functionality you need. This keeps your bundle size minimal.
- Modern Syntax: Day.js follows modern JavaScript syntax, making it easier to integrate with modern codebases.
- Compatibility: Day.js is designed to be a drop-in replacement for Moment.js, with a similar API.
Cons
- Fewer Built-in Features: Day.js has fewer built-in features compared to Moment.js. Advanced functionality requires additional plugins.
- Limited Timezone Support: Day.js relies on third-party plugins for comprehensive timezone support, whereas Moment.js has Moment Timezone.
- Less Mature Ecosystem: As a newer library, Day.js has fewer integrations and community support compared to the well-established Moment.js.
Moment.js
Pros
- Feature-Rich: Moment.js provides a comprehensive set of features for almost any date and time manipulation task.
- Mature Ecosystem: Moment.js has been around since 2011, resulting in extensive community support, plugins, and integrations.
- Timezone Handling: Moment.js, with its Moment Timezone plugin, offers robust timezone support, making it ideal for applications that need to handle multiple time zones.
- Detailed Documentation: Moment.js has detailed and well-maintained documentation, making it easier for developers to find help and examples.
Cons
- Large Size: Moment.js is significantly larger than Day.js, which can impact performance, especially in client-side applications.
- Mutable Operations: Moment.js operations mutate the original date object, which can lead to unexpected behavior if not managed carefully.
- Deprecated: The Moment.js team has deprecated the library, recommending alternatives like Day.js for new projects.
Comparison Table
Feature | Day.js | Moment.js | |||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
~2KB | ~70KB | |||||||||||||||||||||||||||||||||
Immutability |
Yes | No | |||||||||||||||||||||||||||||||||
Plugin Architecture |
Yes | Limited | |||||||||||||||||||||||||||||||||
Feature Set |
Basic (extensible via plugins) | Comprehensive | |||||||||||||||||||||||||||||||||
|
Limited (via plugins) | Extensive (Moment Timezone) | |||||||||||||||||||||||||||||||||
Ecosystem |
Growing | Mature | |||||||||||||||||||||||||||||||||
Documentation | Good | Extensive | |||||||||||||||||||||||||||||||||
Modern Syntax |
Yes | No | |||||||||||||||||||||||||||||||||
API Compatibility |
Similar to Moment.js | N/A | |||||||||||||||||||||||||||||||||
Status | Actively maintained | Deprecated |
When working with dates in JavaScript, it’s essential to choose the right tool for the task. Native JavaScript Date objects are sufficient for basic date manipulation tasks, but for more advanced operations, libraries like Day.js and Moment.js offer powerful and convenient features.
When deciding between Day.js and Moment.js, consider the specific needs of your project.
The above is the detailed content of Working with Date in Javascript: new Date() vs Day.js vs Moment.js. For more information, please follow other related articles on the PHP Chinese website!
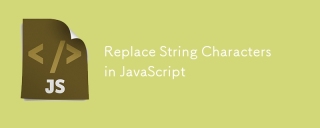
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
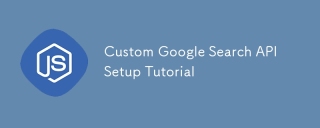
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
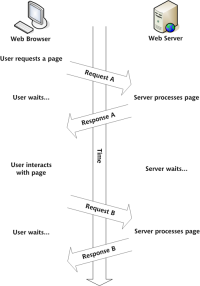
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
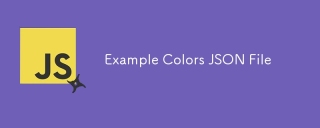
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
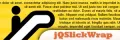
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
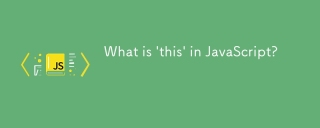
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
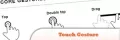
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
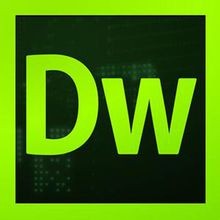
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
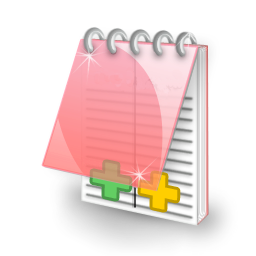
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!
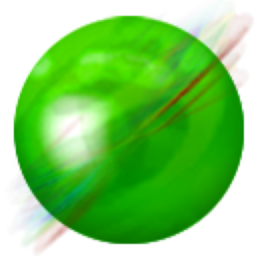
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
