To improve the testability of classes in C++, you can take the following measures: Use a unit testing framework to organize and run test cases. Use friend classes to test private members. Use dependency injection to improve loose coupling of components. Provide clear error messages so it's easy to understand why a test failed. Write unit tests to cover various functionality of the class.
How to enhance the testability of classes in C++
Testable code is an important part of the modern software development process. It allows us to confidently release new features in production while also reducing the number of bugs. In this article, we'll explore how to design a C++ class to improve its testability.
1. Use a unit testing framework
Using a unit testing framework is the first step to improve the testability of your class. These frameworks provide support for test case organization, assertions, and simulations. Some popular C++ unit testing frameworks include Google Test, Catch2, and Boost.Test.
2. Use friend classes for white-box testing
Friend classes allow other classes to access private members. This is very useful in unit testing because it allows us to test the internal implementation that is usually hidden behind the class declaration. For example:
class MyClass { private: int secret_number; friend class MyClassTester; public: int get_secret_number() { return secret_number; } }; class MyClassTester { public: static void test_get_secret_number(MyClass& my_class) { int expected_value = 42; my_class.secret_number = expected_value; int actual_value = my_class.get_secret_number(); ASSERT_EQ(expected_value, actual_value); } };
3. Use dependency injection to improve loose coupling
Loosely coupled components are easier to test because it allows us to isolate and test individual parts. Dependency injection is a design pattern that allows us to pass the dependencies of an object instead of hardcoding them in the class constructor. For example:
class MyService { public: MyService(ILogger& logger) : logger_(logger) {} void do_something() { logger_.log("Doing something"); } private: ILogger& logger_; }; class MockLogger : public ILogger { public: MOCK_METHOD(void, log, (const std::string& message), (override)); }; TEST(MyServiceTest, DoSomething) { MockLogger mock_logger; EXPECT_CALL(mock_logger, log("Doing something")); MyService service(mock_logger); service.do_something(); }
4. Provide clear error messages
When a test fails, a clear error message is critical to solving the problem. Classes should be designed to throw useful exceptions or return codes when an error occurs so that we can easily understand why the test failed. For example:
class MyClass { public: int divide(int numerator, int denominator) { if (denominator == 0) { throw std::invalid_argument("Denominator cannot be zero."); } return numerator / denominator; } }; TEST(MyClassTest, DivideByZero) { MyClass my_class; EXPECT_THROW(my_class.divide(1, 0), std::invalid_argument); }
5. Writing Unit Tests
In addition to friend classes and dependency injection, writing unit tests for your classes is crucial to improving testability . Unit tests should cover every part of the class, including constructors, methods, and error handling.
Practical combat
Let’s take a practical example. Suppose we have a MyClass
class, which has an increment
method that increments the class's value
member variable when called.
class MyClass { public: MyClass() : value(0) {} int get_value() { return value; } void increment() { ++value; } private: int value; }; TEST(MyClassTest, Increment) { MyClass my_class; int expected_value = 1; my_class.increment(); int actual_value = my_class.get_value(); ASSERT_EQ(expected_value, actual_value); }
This is just a simple example of how to design a C++ class to improve its testability. By following these principles, we can create code that is easier to test and maintain.
The above is the detailed content of How to improve code testability in C++ class design?. For more information, please follow other related articles on the PHP Chinese website!
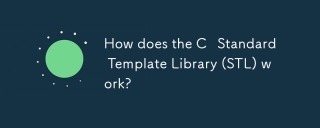
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
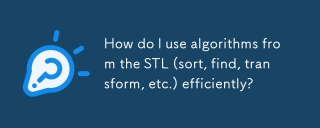
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
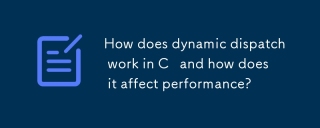
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
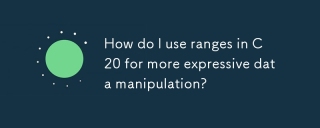
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
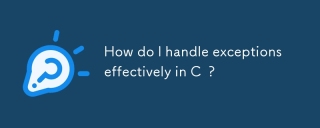
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
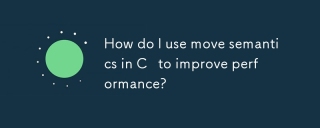
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
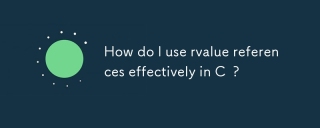
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
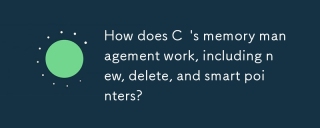
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
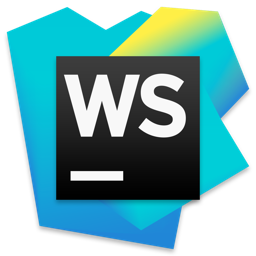
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
