Performance tuning principles for C++ container libraries
Principles for optimizing the performance of C++ container libraries: Choose an appropriate container, such as vector for fast access and list for insertion/deletion. Pre-allocate container capacity to avoid memory reallocation. Use references or pointers to avoid unnecessary copies. Reduce search and sort operations, use appropriate comparators and efficient algorithms.
Performance Tuning Principles of C++ Container Library
The C++ Standard Template Library (STL) provides a series of powerful container classes that can greatly simplify Code organization and management. However, without proper tuning, containers can become a bottleneck for application performance.
Choose the right container
First, choosing the right container is critical for performance. Depending on your application's specific needs, you can choose from a variety of containers, including vector, list, map, and set.
- vector is a dynamic array used for quick sorting and random access.
- list is a doubly linked list, used for frequent insertion and deletion operations.
- map and set are associative containers used to find and sort by key value.
Capacity preallocation
When creating a container, preallocating enough capacity can avoid multiple memory reallocations when adding elements. This is particularly important for performance as it reduces memory fragmentation and increases insert speed.
vector<int> v(100); // 预分配容量为 100
Avoid unnecessary copies
By using references or pointers, unnecessary copy operations can be avoided. For example:
vector<string>& v = my_func(); // 获取引用,避免拷贝
Reduce search and sort operations
Frequent search or sort operations on containers may affect performance. These operations can be reduced by:
- Using appropriate comparators for map and set.
- Use binary search to efficiently find elements in vector.
Practical case
In an image processing application, vector
vector<int>* image_data = new vector<int>(10000); // 预分配容量 ... // 从文件中读取图像数据 image = cv::Mat(1000, 1000, CV_8UC3, image_data); // 使用指针避免拷贝
By applying these principles, you can significantly improve the performance of container libraries in C++ applications. By carefully selecting containers, preallocating capacity, avoiding unnecessary copies, and reducing lookup and sort operations, you can create efficient and scalable code.
The above is the detailed content of Performance tuning principles for C++ container libraries. For more information, please follow other related articles on the PHP Chinese website!
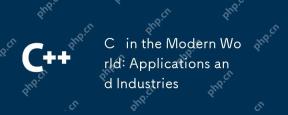
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
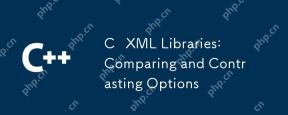
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
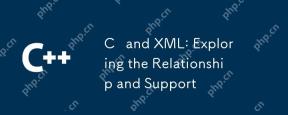
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
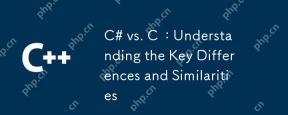
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
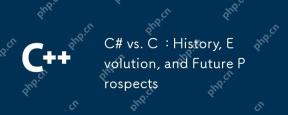
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
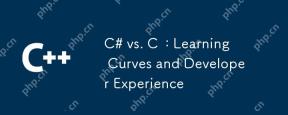
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
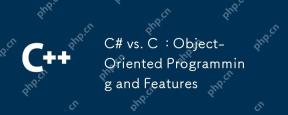
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
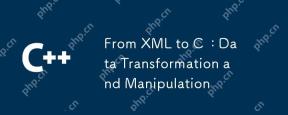
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
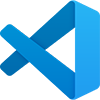
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!