The virtual pointer mechanism in C++ is implemented through a virtual table, which contains function pointers pointing to member functions of the class. When a base class pointer points to a derived class object, the virtual pointer stores the virtual table address, which is used by the compiler to find and call the correct virtual function. This mechanism allows polymorphism, that is, using base class pointers to operate derived class objects, improving the maintainability and scalability of the code. But it will increase memory overhead and reduce performance.
The implementation mechanism of virtual pointers in C++
Virtual pointers are the core mechanism for realizing polymorphism in object-oriented programming . It allows base class pointers to point to objects of derived classes and call methods in derived classes.
Virtual table
The virtual mechanism in C++ is implemented through virtual tables. Every class has a vtable, which is an array containing function pointers. Function pointers in the virtual table point to member functions of the class.
Virtual function
A virtual function is a function with a virtual table. When a base class pointer points to an object of a derived class, the compiler uses the vtable to find the correct method to call.
Virtual pointer
A virtual pointer is a pointer that stores the address of a virtual table. When the compiler needs to execute a virtual function, it uses a virtual pointer to look up the vtable.
Practical case
Consider the following code:
class Shape { public: virtual double area() = 0; }; class Rectangle : public Shape { public: double width; double height; double area() override { return width * height; } }; class Circle : public Shape { public: double radius; double area() override { return 3.14 * radius * radius; } }; int main() { Shape* shapes[] = {new Rectangle(5, 10), new Circle(5)}; for (Shape* shape : shapes) { cout << "Area: " << shape->area() << endl; } return 0; }
In this example, the area()
function is a virtual function. When the compiler calls area()
in the main function, it uses virtual pointers to find the correct version to call.
Implementation details
Virtual pointers and virtual tables are usually generated by the compiler at compile time. Virtual pointers are usually stored at the beginning of the object, while virtual tables are stored in a global data segment.
Advantages
- Allows polymorphism, that is, using base class pointers to operate derived class objects.
- Improves the maintainability and scalability of the code.
- Avoid the overhead of type conversion.
Disadvantages
- Increased memory overhead because each class has a virtual table.
- May reduce performance because the compiler needs to perform extra lookups when executing virtual functions.
The above is the detailed content of How is virtual pointer implemented in C++?. For more information, please follow other related articles on the PHP Chinese website!
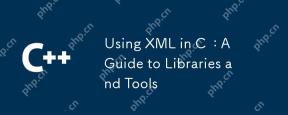
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
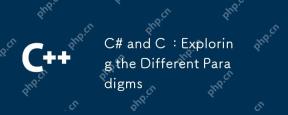
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
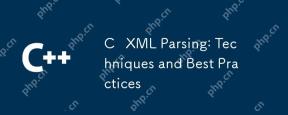
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
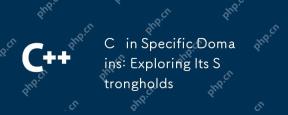
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
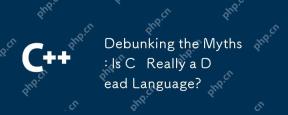
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
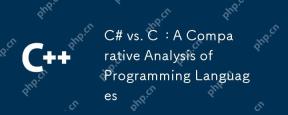
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
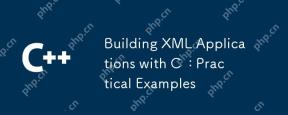
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
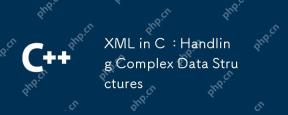
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
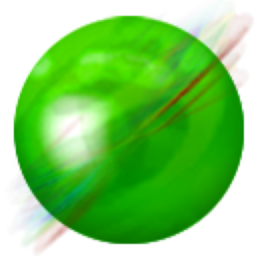
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
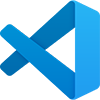
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
