Theory and practice of performance tuning of java framework
Java framework performance tuning involves identifying bottlenecks, optimizing code and configuration. Common techniques include caching, concurrency, load balancing, and code optimization. A practical case (Spring Boot application) demonstrates the significant improvement in application performance after using caching. By understanding the theoretical foundations and adopting best practices, developers can optimize the performance of Java framework applications, improve user experience and system stability.
The theory and practice of Java framework performance tuning
Theoretical basis
- Performance indicators: response time, throughput, memory usage, CPU utilization
- Performance tuning principles: identify bottlenecks, optimize code, optimize configuration
- Common performance tuning tips: Caching, concurrency, load balancing, code optimization
Practical cases
Identification of bottlenecks
- Usage Performance monitoring tools (such as JMeter, New Relic)
- Analyze log files
Optimize code
- Avoid using blocking operations
- Optimize data structures and algorithms
- And release resources when destroying objects
Optimize configuration
- Adjust the thread pool size
- Adjust the garbage collector settings
- Optimize the database connection pool
##The following is a practical case that shows how performance tuning can improve Spring Boot application performance:
@RestController public class MyController { // 原代码(存在性能问题) @GetMapping("/data") public ResponseEntity<List<Entity>> getData() { List<Entity> data = entityService.findAll(); return ResponseEntity.ok(data); } // 优化后的代码(使用缓存) private Cache<String, List<Entity>> dataCache = CacheManager.getCache("myData"); @GetMapping("/data") public ResponseEntity<List<Entity>> getData() { String key = "allData"; List<Entity> data = dataCache.get(key); if (data == null) { data = entityService.findAll(); dataCache.put(key, data); } return ResponseEntity.ok(data); } }By using caching, we avoid querying data from the database on every request, thus significantly improving performance.
Conclusion
By understanding the theory and practice of performance tuning, developers can significantly improve the performance of Java framework applications. With the proper techniques, you can significantly reduce response times, increase throughput, and optimize resource utilization.The above is the detailed content of Theory and practice of performance tuning of java framework. For more information, please follow other related articles on the PHP Chinese website!
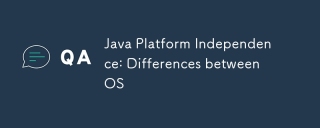
There are subtle differences in Java's performance on different operating systems. 1) The JVM implementations are different, such as HotSpot and OpenJDK, which affect performance and garbage collection. 2) The file system structure and path separator are different, so it needs to be processed using the Java standard library. 3) Differential implementation of network protocols affects network performance. 4) The appearance and behavior of GUI components vary on different systems. By using standard libraries and virtual machine testing, the impact of these differences can be reduced and Java programs can be ensured to run smoothly.
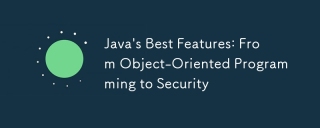
Javaoffersrobustobject-orientedprogramming(OOP)andtop-notchsecurityfeatures.1)OOPinJavaincludesclasses,objects,inheritance,polymorphism,andencapsulation,enablingflexibleandmaintainablesystems.2)SecurityfeaturesincludetheJavaVirtualMachine(JVM)forsand
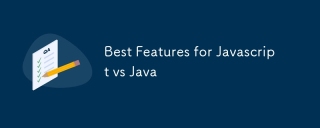
JavaScriptandJavahavedistinctstrengths:JavaScriptexcelsindynamictypingandasynchronousprogramming,whileJavaisrobustwithstrongOOPandtyping.1)JavaScript'sdynamicnatureallowsforrapiddevelopmentandprototyping,withasync/awaitfornon-blockingI/O.2)Java'sOOPf
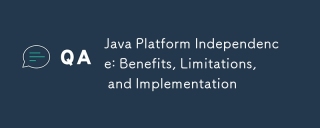
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM)andbytecode.1)TheJVMinterpretsbytecode,allowingthesamecodetorunonanyplatformwithaJVM.2)BytecodeiscompiledfromJavasourcecodeandisplatform-independent.However,limitationsincludepotentialp
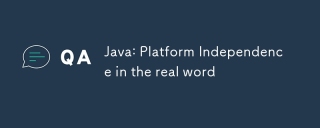
Java'splatformindependencemeansapplicationscanrunonanyplatformwithaJVM,enabling"WriteOnce,RunAnywhere."However,challengesincludeJVMinconsistencies,libraryportability,andperformancevariations.Toaddressthese:1)Usecross-platformtestingtools,2)
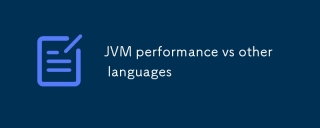
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
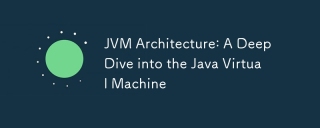
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
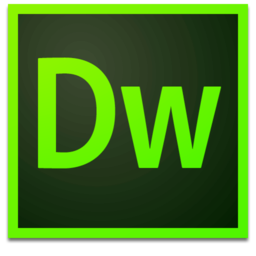
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
