


How to simplify PHP database connections using ORM (Object Relational Mapping)?
Use ORM to simplify PHP database connection and map tables and data in the relational database to objects in the application, which can greatly simplify the interaction with the database. Benefits of ORM include simplified CRUD operations, automatic mapping, object relations, and improved maintainability. Practical example: Use the Doctrine framework to create and persist entities in PHP, interacting with the database by adding them to a persistence context and committing changes.
Simplify PHP database connection using ORM
Object-relational mapping (ORM) is a pattern that combines the Tables and data are mapped to objects in the application. Using an ORM can greatly simplify interaction with the database, thereby improving development efficiency and code maintainability.
What is ORM?
ORM acts as a layer of abstraction between object and relational databases. It automatically maps object properties to columns in database tables and provides convenient methods for create, read, update, and delete (CRUD) operations.
Benefits
The main benefits of using ORM include:
- Simplified CRUD operations: ORM provides simple API to easily create, read, update and delete data from the database.
- Automatic mapping: ORM automatically maps object properties to columns in the database without writing cumbersome SQL queries.
- Object relationship: ORM can automatically manage the relationship between objects, such as one-to-many or many-to-many relationships.
- Improve maintainability: By using ORM, the database interaction logic can be separated from the business logic, improving the maintainability and readability of the code.
Practical Case
Using Doctrine, a popular ORM framework in PHP, to provide a practical case.
Install Doctrine
composer require doctrine/orm
Configuration file
Configuration file in config/orm.yml
As follows:
doctrine: dbal: url: 'mysql://root:@localhost:3306/doctrine_db' driver: pdo_mysql orm: auto_generate_proxy_classes: true metadata_cache_driver: array query_cache_driver: array
Entity definition
Create entities for mapping to database tables:
// src/Entity/Product.php namespace App\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity */ class Product { /** * @ORM\Id * @ORM\GeneratedValue * @ORM\Column(type="integer") */ private $id; /** * @ORM\Column(type="string", length=255) */ private $name; /** * @ORM\Column(type="float") */ private $price; // 省略其他代码... }
Use ORM
Create a new product using Doctrine in the controller:
// src/Controller/ProductController.php namespace App\Controller; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\Request; use App\Entity\Product; use Doctrine\ORM\EntityManagerInterface; class ProductController extends AbstractController { public function create(Request $request, EntityManagerInterface $entityManager) { $product = new Product(); $product->setName('New Product'); $product->setPrice(10.99); $entityManager->persist($product); $entityManager->flush(); return $this->redirectToRoute('product_index'); } }
In the above example, the persist()
method adds the new product to the management associated with the persistence context in the object list. flush()
The method commits all changes to the persistence context to the database.
The above is the detailed content of How to simplify PHP database connections using ORM (Object Relational Mapping)?. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
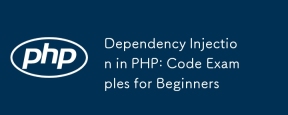
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
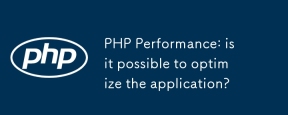
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
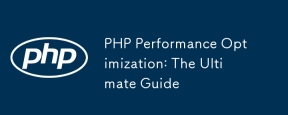
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
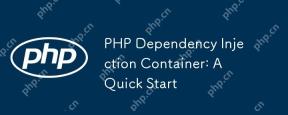
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
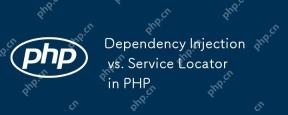
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
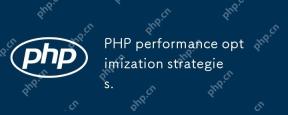
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
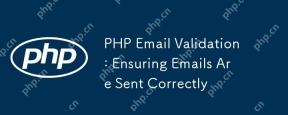
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
