


What are the best practices for using PHP frameworks in microservices architecture?
Best practices for using PHP frameworks in microservices architecture include: Choose a lightweight and modular framework such as Laravel, Symfony or Zend Framework. Use a modular architecture to break your application into smaller components. Leverage containers to simplify the deployment and management of microservices. Use an API gateway to route and secure requests between microservices. Use microservice communication patterns such as REST API, message queue, or event bus.
Best practices for using PHP framework in microservice architecture
In microservice architecture, choose the appropriate PHP framework for Creating applications that are modular, scalable, and maintainable is critical. This article introduces some best practices that show how to effectively use PHP frameworks in a microservices environment.
1. Choose the right framework
There are many PHP frameworks to choose from, each with its own advantages and disadvantages. For microservice architecture, it is recommended to use lightweight and modular frameworks, such as:
- [Laravel](https://laravel.com/)
- [Symfony]( https://symfony.com/)
- [Zend Framework](https://framework.zend.com/)
2. Use modular architecture
Microservice architecture is modular in nature. Each service should be built as an independent unit, with its own code base, configuration, and dependencies. A PHP framework should support modularity, allowing you to break down your application into smaller components.
For example, in Laravel, you can use Service Providers and Facades to define modules and services.
3. Using containers
Containers can simplify the deployment and management of microservices. Using container technologies like Docker or Kubernetes, you can package your microservices, including all dependencies, and easily deploy them in different environments.
4. Adopt API Gateway
API Gateway is a middle layer responsible for routing and protecting requests between microservices. It simplifies communication and provides centralized access control.
In PHP, you can easily build an API gateway using frameworks such as [Lumen](https://lumen.laravel.com/) or [Slim](https://www.slimframework.com/) .
5. Using microservice communication patterns
Microservices can communicate using a variety of mechanisms, such as REST APIs, message queues, and event buses. Choose the mode that best suits your scenario and use libraries supported by the PHP framework to implement communication.
Practical Case: Building Microservices using Laravel
To illustrate these best practices, let’s create a simple microservice using Laravel:
// 定义服务提供器 class ExampleServiceProvider extends ServiceProvider { public function register() { $this->app->bind('ExampleService', 'ExampleService'); } } // 定义服务 class ExampleService { public function getHello() { return 'Hello from ExampleService!'; } }
You can then use the service in your controller:
class ExampleController extends Controller { public function index() { // 获取服务 $service = $this->app->make('ExampleService'); // 调用服务的方法 $message = $service->getHello(); // 返回响应 return $message; } }
This example shows how to use Laravel to build a simple modular microservice that implements the service provider pattern and dependency injection.
The above is the detailed content of What are the best practices for using PHP frameworks in microservices architecture?. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
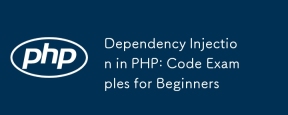
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
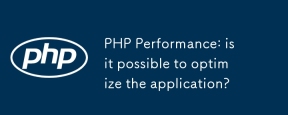
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
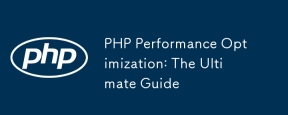
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
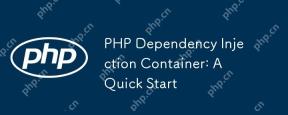
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
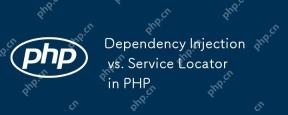
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
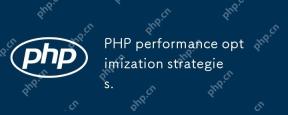
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
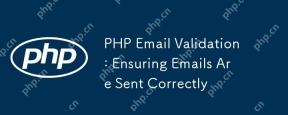
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
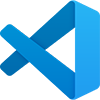
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
