


The transaction processing mechanism in PHP is designed to ensure the integrity of database operations. It allows a set of operations to be executed in full or in none. This is accomplished by starting a transaction, performing operations, and committing or rolling back the transaction as appropriate. By using transactions, you can ensure that the database remains consistent during updates.
Detailed explanation of transaction processing mechanism in PHP database connection
Transaction processing
Transaction processing is a series of operations that maintain the integrity of the database and ensure the consistency of the database. In PHP, you can use transactions to ensure that either all or none of a set of operations are performed.
Transaction Functions
To start a transaction, you can use the following functions:
mysqli_begin_transaction($mysqli);
Once the transaction has started, you can perform operations. After all operations have been performed, the transaction can be committed or rolled back:
mysqli_commit($mysqli); //提交事务 mysqli_rollback($mysqli); //回滚事务
Practical Case
Suppose we have an e-commerce website through which users can purchase goods. When a user places an order, the following three tables need to be updated:
-
users
table - user information -
orders
table - order information -
order_items
Table - Items included in the order
In order to ensure the integrity of these three tables, we can use transaction processing during the order process:
<?php $mysqli = new mysqli("localhost", "root", "password", "ecomm"); // 开启事务 $mysqli->begin_transaction(); try { // 更新 users 表 $sql = "UPDATE users SET balance = balance - ? WHERE id = ?"; $stmt = $mysqli->prepare($sql); $stmt->bind_param("di", $_POST['balance'], $_POST['user_id']); $stmt->execute(); // 更新 orders 表 $sql = "INSERT INTO orders (user_id, total_amount) VALUES (?, ?)"; $stmt = $mysqli->prepare($sql); $stmt->bind_param("di", $_POST['user_id'], $_POST['total_amount']); $stmt->execute(); // 更新 order_items 表 foreach ($_POST['items'] as $item) { $sql = "INSERT INTO order_items (order_id, product_id, quantity, unit_price) VALUES (?, ?, ?, ?)"; $stmt = $mysqli->prepare($sql); $stmt->bind_param("iiii", $last_inserted_order_id, $item['product_id'], $item['quantity'], $item['unit_price']); $stmt->execute(); } // 提交事务 $mysqli->commit(); echo "Order placed successfully!"; } catch (Exception $e) { // 如果出现异常,回滚事务 $mysqli->rollback(); echo "An error occurred while placing the order."; } ?>
In the above code, we first start a transaction, and then try to perform three operations to update the table. If all operations succeed, the transaction is committed. If one of the operations fails, the transaction is rolled back and an error message is displayed to the user.
Notes
When using transaction processing, you need to pay attention to the following points:
- All operations in the transaction must be in the same database Executed during connection.
- All operations in a transaction must be atomic, meaning that they are either all executed or none of them are executed.
- Transactions may cause deadlocks, so care needs to be taken when designing transaction processing code.
The above is the detailed content of Detailed explanation of transaction processing mechanism in PHP database connection. For more information, please follow other related articles on the PHP Chinese website!
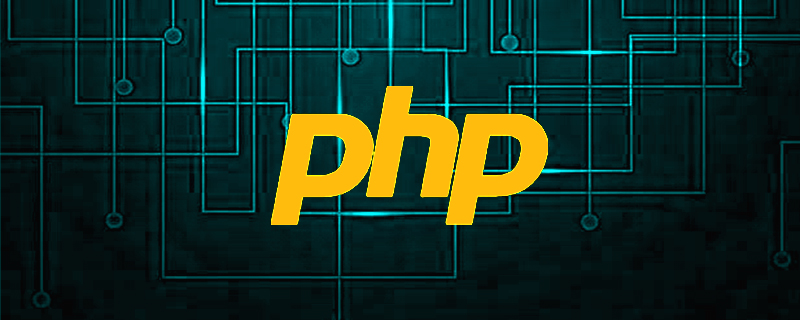
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
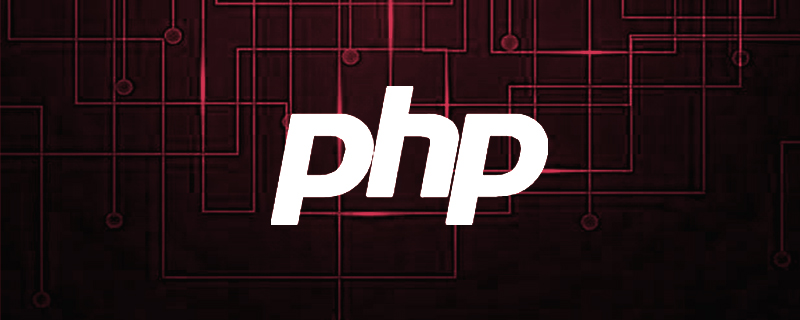
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
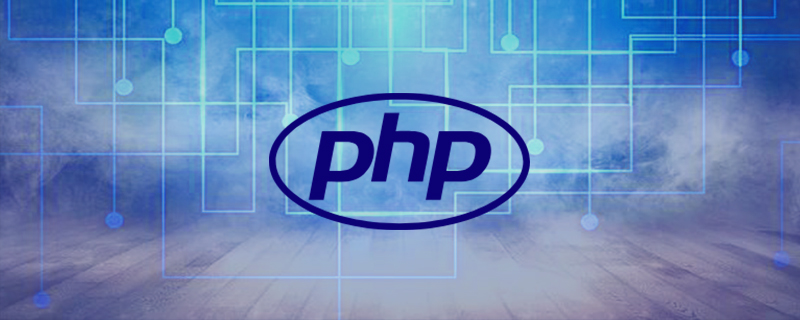
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
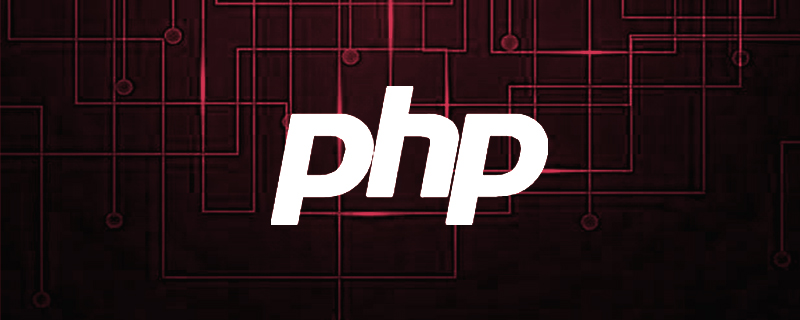
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
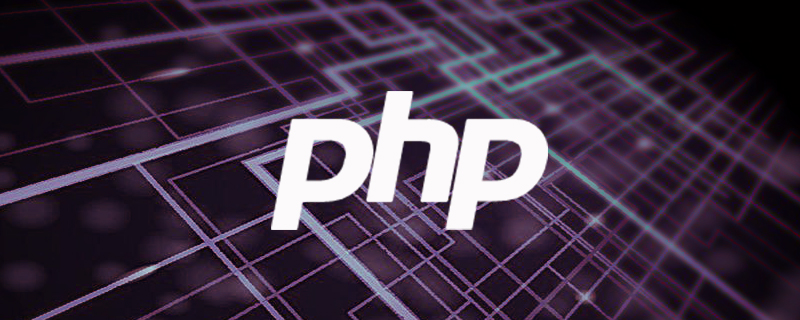
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
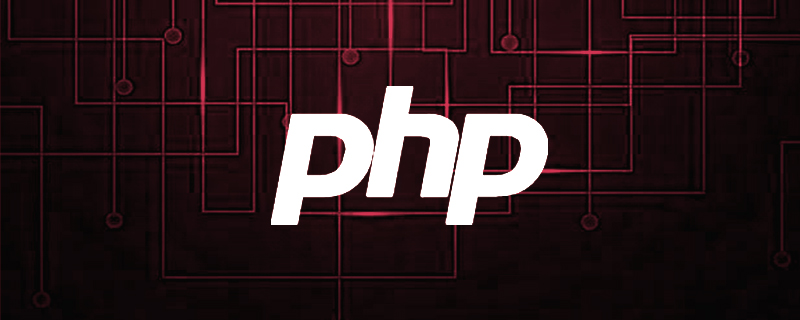
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
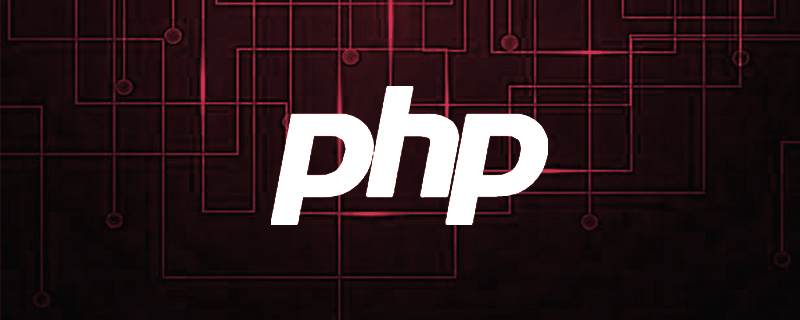
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
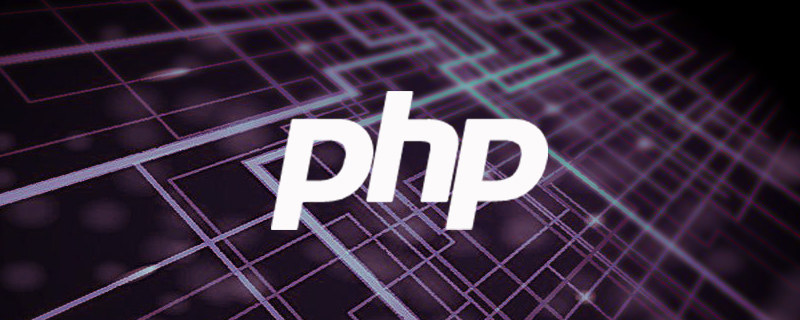
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
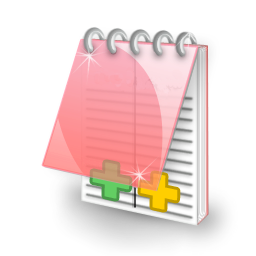
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!
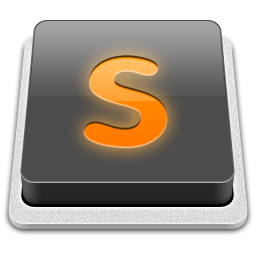
SublimeText3 Mac version
God-level code editing software (SublimeText3)
