Real-time performance and reliability of C++ in embedded systems
C++ is competent in real-time and reliability requirements in embedded systems: Real-time: low latency, priority control, hardware-level access Reliability: type safety, resource management, exception handling Practical case: controlling wind turbines, requiring low Latency and reliability
Real-time and reliability of C++ in embedded systems
Embedded systems usually require real-time and reliability, and C++ has significant advantages in these aspects.
1. Real-time
- Low latency: The C++ compiler will optimize the code into efficient machine instructions to achieve low latency. Delayed execution.
- Priority control: C++ supports multi-threading and priority mechanisms, allowing programmers to control the execution order and priority of tasks.
- Hardware-level access: C++ allows direct access to hardware registers and devices, which enables fast response to real-time events.
2. Reliability
- Type safety: C++’s type system helps avoid memory errors and data corruption and other common software defects.
- Resource management: C++’s resource management mechanism (such as RAII) ensures that resources are properly cleaned up and prevents problems such as memory leaks and resource deadlocks.
- Exception handling: C++’s exception handling mechanism allows programmers to catch and handle runtime errors, thereby enhancing the reliability of applications.
Practical case:
Controlling a wind turbine
An embedded system that controls a wind turbine requires real-time monitoring wind speed and blade position, and sends precise control signals to the actuators. C++ was used for this system because its low latency and priority control capabilities ensure real-time response and reliability of the system.
Code example:
// 实时风速监测线程 void windSpeedMonitorThread() { while (true) { float windSpeed = readWindSpeedSensor(); // ... // 使用互斥锁保护共享数据 std::lock_guard<std::mutex> lock(windSpeedMutex); // 更新风速数据 currentWindSpeed = windSpeed; } } // 叶片位置控制线程 void bladePositionControlThread() { while (true) { float bladePosition = readBladePositionSensor(); // ... // 根据参考位置和当前位置计算控制信号 float controlSignal = calculateControlSignal(referencePosition, bladePosition); // 发送控制信号给执行器 sendControlSignal(controlSignal); } }
In this code, the windSpeedMonitorThread
thread is responsible for real-time monitoring of wind speed, and the bladePositionControlThread
thread Responsible for calculating and sending control signals based on real-time wind speed data. C++'s thread mechanism and mutex protection mechanism ensure synchronization and reliability between the two threads.
The above is the detailed content of Real-time performance and reliability of C++ in embedded systems. For more information, please follow other related articles on the PHP Chinese website!
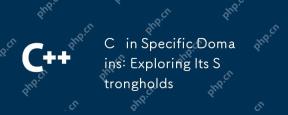
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
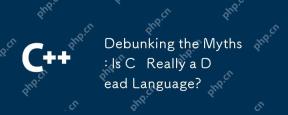
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
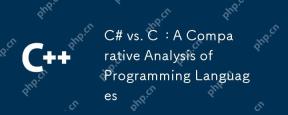
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
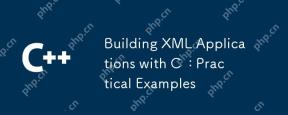
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
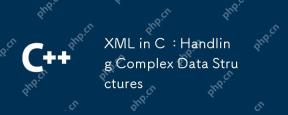
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
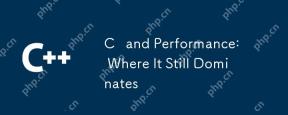
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
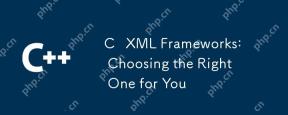
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
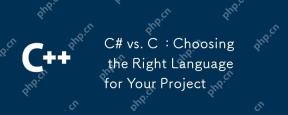
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
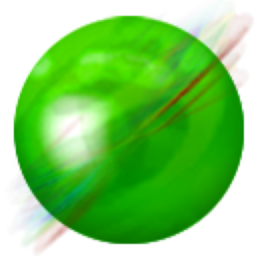
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
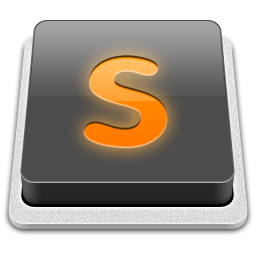
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
