Optimize the concurrency performance of Java frameworks using thread pools
Yes, using a thread pool can optimize the concurrency performance of the Java framework. Thread pools improve efficiency by pre-allocating and managing threads, thereby: reducing the overhead of creating and destroying threads and improving performance. Adjust the pool's parameters to handle increased load and improve scalability. Limit the number of threads active at the same time, reduce the risk of system overload and deadlock, and improve stability.
Use the thread pool to optimize the concurrency performance of the Java framework
Introduction:
The Java framework is Bottlenecks are often encountered when handling concurrent requests. Thread pools improve performance by efficiently managing tasks that are executed in parallel. This article will introduce how to use thread pools to optimize the concurrency performance of the Java framework and provide a practical case.
Thread pool overview:
The thread pool is a mechanism that pre-allocates and maintains a collection of threads. It allows applications to perform tasks asynchronously, thereby avoiding the overhead of creating and destroying threads. The thread pool can be configured with the following parameters:
- Number of core threads: The number of threads that are always active.
- Maximum number of threads: The maximum number of threads allowed in the pool.
- Queue capacity: The number of tasks stored when tasks that are not processed by the thread are waiting to be executed.
Advantages:
The advantages of using a thread pool include:
- Improving performance: By avoiding the frequent creation and destruction of threads, thereby improving efficiency.
- Improve scalability: Increased load can be easily handled by adjusting the parameters of the thread pool.
- Improve stability: The thread pool reduces the risk of system overload and deadlock by limiting the number of threads active at the same time.
Practical case:
The following code shows how to use the thread pool to optimize a simple task processing class in the Java framework:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.concurrent.ThreadPoolExecutor; public class TaskProcessor { private ThreadPoolExecutor threadPool; public TaskProcessor() { // 创建一个固定线程池,核心线程数为 4,最大线程数为 8。 threadPool = (ThreadPoolExecutor) Executors.newFixedThreadPool(4, 8); } public void processTask(Runnable task) { // 将任务提交到线程池。 threadPool.execute(task); } }
Above In the example, the TaskProcessor
class uses a thread pool to execute tasks asynchronously. The thread pool has 4 core threads and can be scaled up to 8 threads to handle concurrent requests.
Conclusion:
By using thread pools, the concurrency performance of the Java framework can be significantly improved. Thread pools provide efficient, scalable, and stable task execution, thereby reducing overhead, improving throughput, and increasing application stability.
The above is the detailed content of Optimize the concurrency performance of Java frameworks using thread pools. For more information, please follow other related articles on the PHP Chinese website!
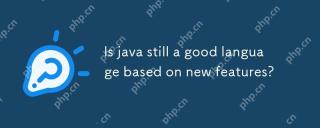
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
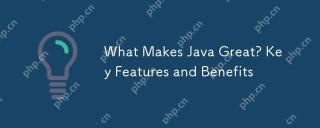
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
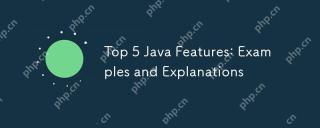
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.
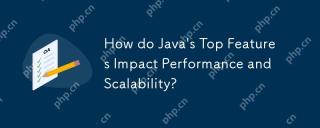
Java'stopfeaturessignificantlyenhanceitsperformanceandscalability.1)Object-orientedprincipleslikepolymorphismenableflexibleandscalablecode.2)Garbagecollectionautomatesmemorymanagementbutcancauselatencyissues.3)TheJITcompilerboostsexecutionspeedafteri
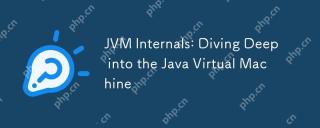
The core components of the JVM include ClassLoader, RuntimeDataArea and ExecutionEngine. 1) ClassLoader is responsible for loading, linking and initializing classes and interfaces. 2) RuntimeDataArea contains MethodArea, Heap, Stack, PCRegister and NativeMethodStacks. 3) ExecutionEngine is composed of Interpreter, JITCompiler and GarbageCollector, responsible for the execution and optimization of bytecode.
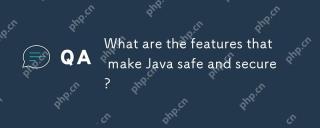
Java'ssafetyandsecurityarebolsteredby:1)strongtyping,whichpreventstype-relatederrors;2)automaticmemorymanagementviagarbagecollection,reducingmemory-relatedvulnerabilities;3)sandboxing,isolatingcodefromthesystem;and4)robustexceptionhandling,ensuringgr
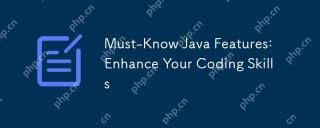
Javaoffersseveralkeyfeaturesthatenhancecodingskills:1)Object-orientedprogrammingallowsmodelingreal-worldentities,exemplifiedbypolymorphism.2)Exceptionhandlingprovidesrobusterrormanagement.3)Lambdaexpressionssimplifyoperations,improvingcodereadability
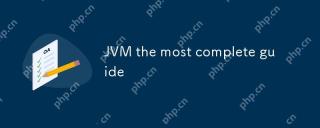
TheJVMisacrucialcomponentthatrunsJavacodebytranslatingitintomachine-specificinstructions,impactingperformance,security,andportability.1)TheClassLoaderloads,links,andinitializesclasses.2)TheExecutionEngineexecutesbytecodeintomachineinstructions.3)Memo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
