C In graphics programming, object-oriented design (OOP) adopts the following principles: encapsulation, inheritance, polymorphism. The advantages of OOP include code readability, maintainability, reusability, and scalability. Examples include: using the Sphere class to encapsulate the sphere properties (radius) and drawing methods, creating a sphere object and displaying it on the screen through the drawing method.
C Object-oriented design ideas in graphics programming
Object-oriented design (OOP) is a programming paradigm that focuses on Create objects and use their properties and methods to represent real-world entities. In C graphics programming, OOP can greatly improve code readability, maintainability, and reusability.
OOP principles
OOP follows the following principles:
- Encapsulation: Encapsulate data and operations in objects , to hide internal implementation details.
- Inheritance: Allows subclasses to inherit the properties and methods of a parent class, thereby creating a hierarchy.
- Polymorphism: Allows subclass objects to use the same interface to express different behaviors.
Object-oriented graphics programming
In graphics programming, you can represent the following elements as objects:
- Shapes: Such as circles, rectangles and polygons.
- Images: Bitmaps and textures.
- Camera: Define viewpoint and projection.
- Mesh: A collection of polygons representing a 3D object.
Practical case: Drawing a sphere
The following is a C code example using OOP to draw a sphere:
class Sphere { public: Sphere(float radius) : _radius(radius) {} float getRadius() { return _radius; } void draw() { // 绘制球体的几何形状 } private: float _radius; }; int main() { Sphere sphere(1.0f); sphere.draw(); return 0; }
In this example :
-
Sphere
class encapsulates the properties (radius) and methods (drawing) of the sphere. - Creates a sphere object with a radius of 1.0.
- Draw the sphere on the screen by calling the
draw()
method.
Advantages
Adopting OOP for graphics programming brings many advantages:
- Code readability And maintainability: OOP code is easier to read and maintain because it organizes related code into objects.
- Code Reuse: Through inheritance, you can reuse common code, thereby reducing duplication.
- Extensibility: OOP design makes it easy to add new functionality without rewriting existing code.
The above is the detailed content of C++ graphics programming object-oriented design ideas. For more information, please follow other related articles on the PHP Chinese website!
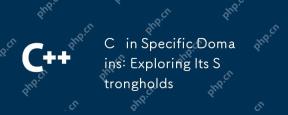
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
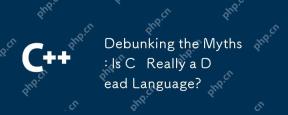
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
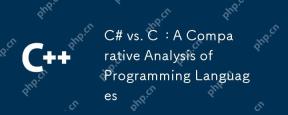
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
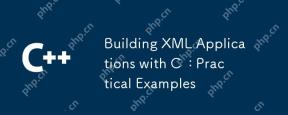
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
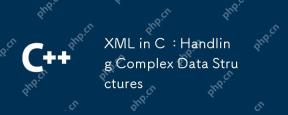
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
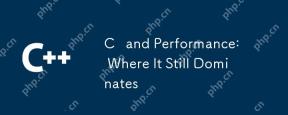
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
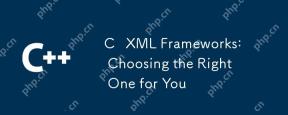
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
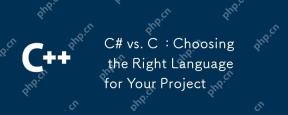
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
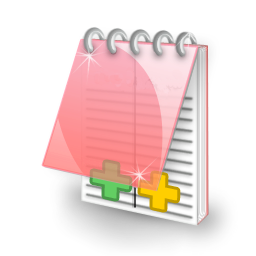
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
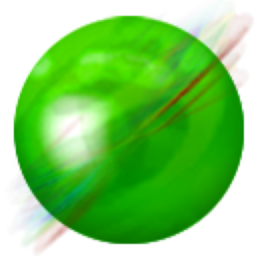
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
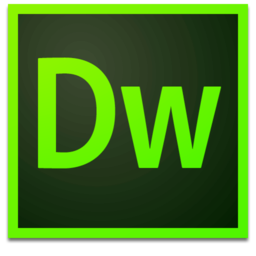
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
