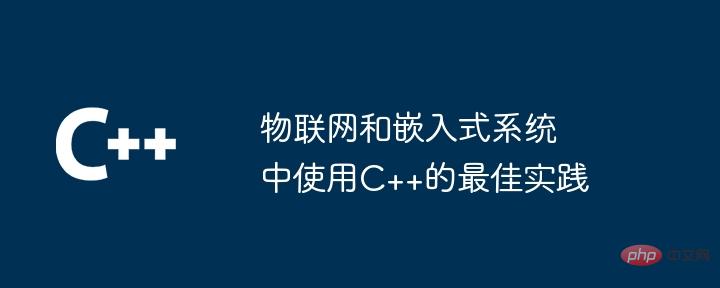
Best Practices for Using C++ in IoT and Embedded Systems
Introduction
C++ is a powerful language that is widely used in IoT and embedded systems. However, using C++ in these restricted environments requires following specific best practices to ensure performance and reliability.
Memory Management
-
Use smart pointers: Smart pointers automatically manage memory to avoid memory leaks and dangling pointers.
-
Consider using a memory pool: Memory pools provide a more efficient way to allocate and free memory than standard malloc()/free().
-
Minimize memory allocation: In embedded systems, memory resources are limited. Reducing memory allocation can improve performance.
Threads and multitasking
-
Use RAII principle: RAII (resource acquisition is initialization) ensures that during the object life cycle Release resources, including threads, when finished.
-
Synchronous access to shared resources: Use synchronization mechanisms such as mutexes and semaphores to ensure the integrity of data when accessing shared resources at the same time.
-
Beware of deadlocks: In a multi-threaded environment, avoid creating situations that may lead to deadlocks.
Real-time
-
Use non-blocking I/O: In a real-time system, blocking I/O will cause Unpredictable delays. With non-blocking I/O, a program can continue executing while waiting for data.
-
Optimize interrupt handling: Minimize the code path in the interrupt handler to reduce interrupt latency.
-
Use timer: Use timer to achieve precise timing and task scheduling.
Power management
-
Reduce object creation: Creating and destroying objects requires energy. Minimize unnecessary object creation.
-
Use static allocation: When possible, use static allocation instead of dynamic allocation because it requires less energy.
-
Consider using low-power mode: Put the processor into low-power mode when not in use.
Code organization
-
Keep the code modular:Organize the code into manageable modules to improve maintainability performance and debugging.
-
Documented API: Clearly document APIs and functions so others can easily understand and use them.
-
Use version control: Use a version control system to track code changes and collaborate on development.
Practical case:
Consider the following example implementation of an IoT device that uses C++ to manage LED status:
#include <Arduino.h>
// LED 引脚号
const int ledPin = 13;
// LED 状态
bool ledState = false;
void setup() {
// 设置 LED 引脚为输出
pinMode(ledPin, OUTPUT);
}
void loop() {
// 改变 LED 状态
ledState = !ledState;
// 根据 LED 状态设置 LED 引脚
digitalWrite(ledPin, ledState);
// 延迟一段时间
delay(500);
}
This example shows Understands the basic principles of using C++ to manage IoT devices, including the use of smart pointers and thread safety technology.
Follow these best practices to ensure efficient and reliable use of C++ in IoT and embedded systems.
The above is the detailed content of Best practices for using C++ in IoT and embedded systems. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn