


By integrating artificial intelligence technology into C++ graphics programming, developers can create more intelligent and interactive applications. These include image classification, object detection, image generation, game AI, path planning, scene generation and other functions. Artificial intelligence technologies such as neural networks, reinforcement learning, and generative adversarial networks can be integrated with C++ through frameworks such as TensorFlow, OpenAI Gym, and PyTorch to realize these functions.
#C++ Graphics Programming Guide to Integrating Artificial Intelligence Technology
Artificial Intelligence (AI) technology is rapidly changing various industries, including graphics programming. By incorporating AI technology into C++ graphics applications, developers can create smarter, more interactive applications.
Neural Networks in Machine Learning
Machine learning is a subfield of AI that enables computers to perform tasks that are not explicitly programmed. One common type of neural network is a convolutional neural network (CNN), which is particularly useful for processing image data.
Integrating CNN in C++ helps to develop the following functions:
- Image classification
- Object detection
- Image generation
// 使用 TensorFlow C++ API 编写一个 CNN 模型以进行图像分类 #include <tensorflow/cc/ops/standard_ops.h> #include <tensorflow/core/framework/graph.pb.h> #include <tensorflow/core/framework/tensor.h> #include <tensorflow/core/public/session.h> using namespace tensorflow; using namespace tensorflow::ops; int main() { // 定义模型结构 GraphDef graph; auto input = Placeholder(graph, DT_FLOAT, {128, 128, 3}); auto conv1 = Conv2D(graph, input, 3, {3, 3}, {1, 1}, "SAME"); auto relu1 = Relu(graph, conv1); auto conv2 = Conv2D(graph, relu1, 3, {3, 3}, {1, 1}, "SAME"); auto relu2 = Relu(graph, conv2); auto pool1 = MaxPool(graph, relu2, {2, 2}, {2, 2}, "SAME"); auto flat = Flatten(graph, pool1); auto dense1 = Dense(graph, flat, 1024); auto relu3 = Relu(graph, dense1); auto dropout1 = Dropout(graph, relu3, 0.5); auto dense2 = Dense(graph, dropout1, 10); // 定义输入数据 Tensor image = Tensor(DT_FLOAT, TensorShape({1, 128, 128, 3})); // ... // 创建 TensorFlow 会话 Session session(graph); // 执行推断 std::vector<Tensor> outputs; session.Run({{input, image}}, {dense2}, {}, &outputs); // 处理结果 const auto& output = outputs[0].scalar<float>(); // ... }
Reinforcement Learning
Reinforcement learning is another subfield of AI that enables computers to learn optimal behaviors to maximize rewards. In C++ graphics applications, reinforcement learning techniques can be leveraged to develop the following features:
- Game AI
- Path Planning
- Scene Generation
// 使用 OpenAI Gym 创建一个强化学习环境 #include <gym/gym.h> using namespace gym; int main() { // 创建环境 auto env = make_env("CartPole-v1"); // 训练代理 auto agent = RandomAgent(env); for (int episode = 0; episode < 1000; episode++) { auto observation = env->reset(); int score = 0; while (true) { auto action = agent.act(observation); observation, score, done, info = env->step(action); if (done) { break; } } std::cout << "Episode " << episode << ": " << score << std::endl; } }
Practical case: Generative Adversarial Network (GAN)
GAN is a type of AI technology that can generate new data, such as images or text. By integrating GANs into C++ graphics applications, developers can create the following functionality:
- Image generation
- Texture synthesis
- Image editing
// 使用 PyTorch C++ API 创建一个 GAN // ... (省略 PyTorch 头文件) int main() { // 定义网络结构 Generator generator; Discriminator discriminator; // 定义损失函数 BCELoss bce_loss; MSELoss mse_loss; // 定义优化器 Adam generator_optimizer(generator->parameters()); Adam discriminator_optimizer(discriminator->parameters()); // 训练循环 for (int epoch = 0; epoch < 100; epoch++) { // ... (省略训练代码) } // 生成图像 auto noise = torch::randn({1, 100}, torch::kFloat32); auto image = generator->forward(noise); // ... (省略保存图像的代码) }
The above is the detailed content of Guide to Integrating Artificial Intelligence Technology into C++ Graphics Programming. For more information, please follow other related articles on the PHP Chinese website!
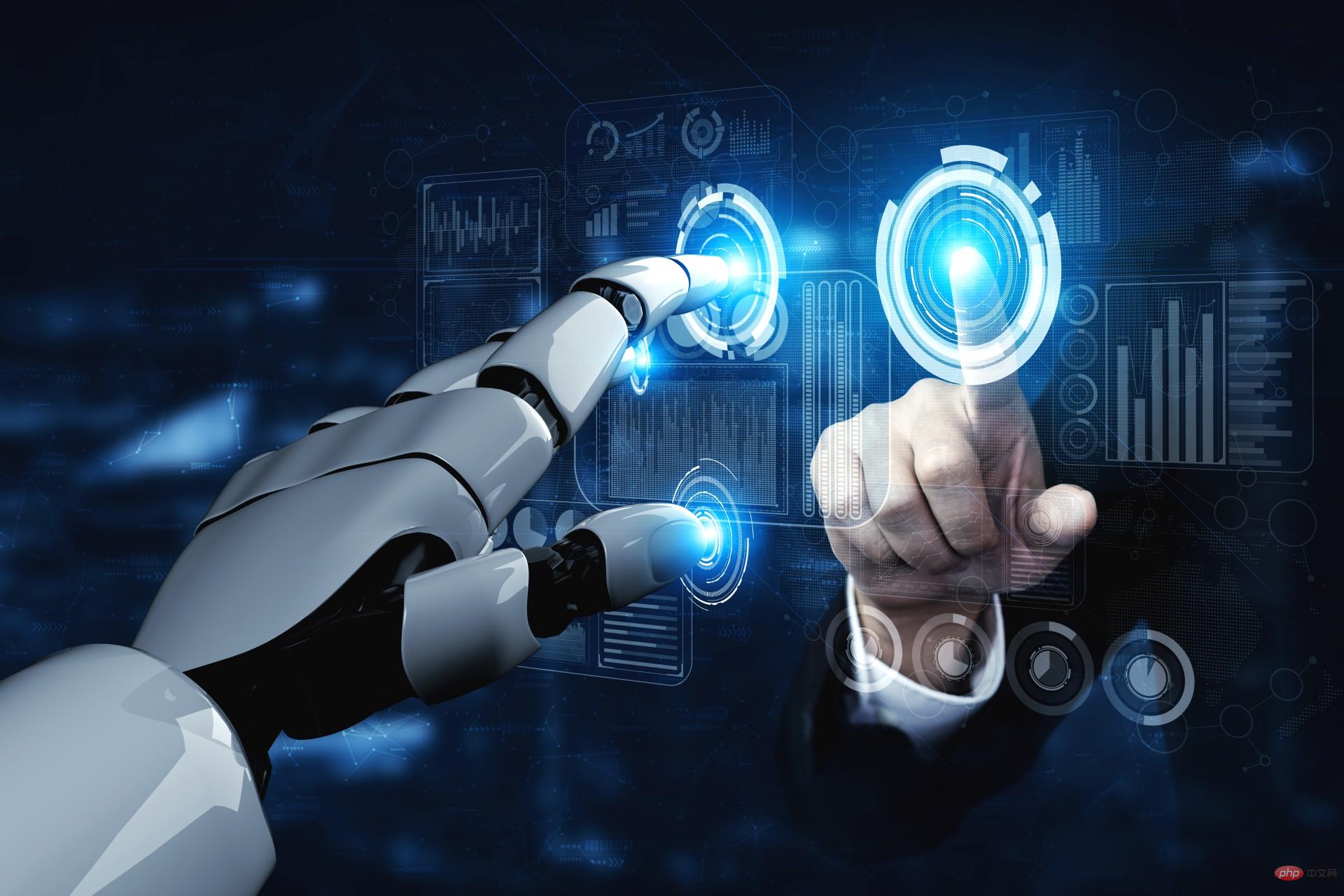
机器学习是一个不断发展的学科,一直在创造新的想法和技术。本文罗列了2023年机器学习的十大概念和技术。 本文罗列了2023年机器学习的十大概念和技术。2023年机器学习的十大概念和技术是一个教计算机从数据中学习的过程,无需明确的编程。机器学习是一个不断发展的学科,一直在创造新的想法和技术。为了保持领先,数据科学家应该关注其中一些网站,以跟上最新的发展。这将有助于了解机器学习中的技术如何在实践中使用,并为自己的业务或工作领域中的可能应用提供想法。2023年机器学习的十大概念和技术:1. 深度神经网
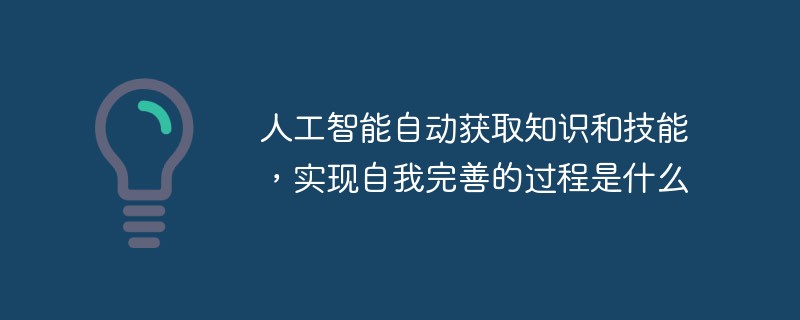
实现自我完善的过程是“机器学习”。机器学习是人工智能核心,是使计算机具有智能的根本途径;它使计算机能模拟人的学习行为,自动地通过学习来获取知识和技能,不断改善性能,实现自我完善。机器学习主要研究三方面问题:1、学习机理,人类获取知识、技能和抽象概念的天赋能力;2、学习方法,对生物学习机理进行简化的基础上,用计算的方法进行再现;3、学习系统,能够在一定程度上实现机器学习的系统。

本文将详细介绍用来提高机器学习效果的最常见的超参数优化方法。 译者 | 朱先忠审校 | 孙淑娟简介通常,在尝试改进机器学习模型时,人们首先想到的解决方案是添加更多的训练数据。额外的数据通常是有帮助(在某些情况下除外)的,但生成高质量的数据可能非常昂贵。通过使用现有数据获得最佳模型性能,超参数优化可以节省我们的时间和资源。顾名思义,超参数优化是为机器学习模型确定最佳超参数组合以满足优化函数(即,给定研究中的数据集,最大化模型的性能)的过程。换句话说,每个模型都会提供多个有关选项的调整“按钮
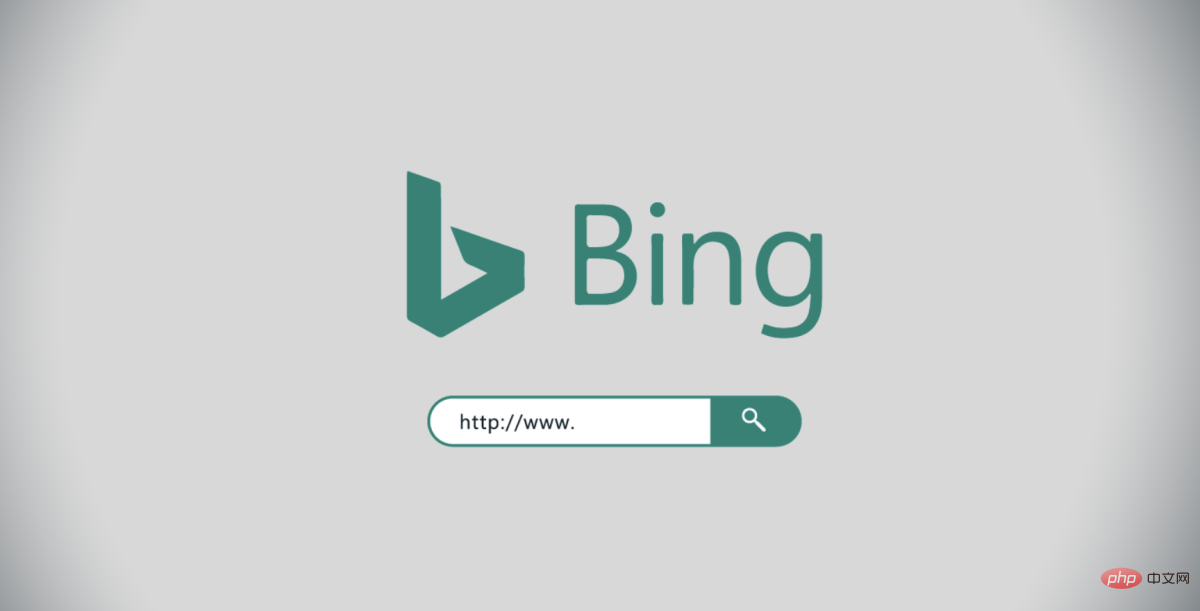
截至3月20日的数据显示,自微软2月7日推出其人工智能版本以来,必应搜索引擎的页面访问量增加了15.8%,而Alphabet旗下的谷歌搜索引擎则下降了近1%。 3月23日消息,外媒报道称,分析公司Similarweb的数据显示,在整合了OpenAI的技术后,微软旗下的必应在页面访问量方面实现了更多的增长。截至3月20日的数据显示,自微软2月7日推出其人工智能版本以来,必应搜索引擎的页面访问量增加了15.8%,而Alphabet旗下的谷歌搜索引擎则下降了近1%。这些数据是微软在与谷歌争夺生
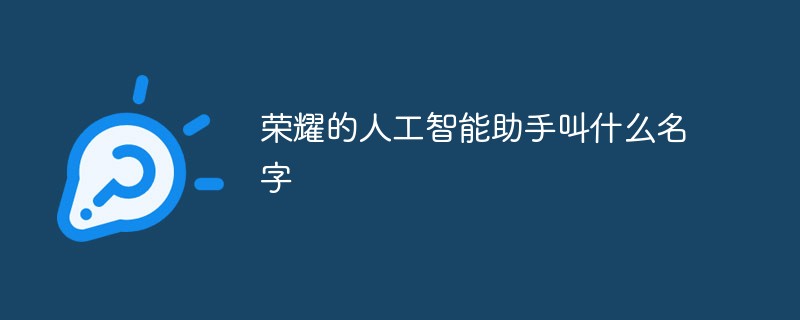
荣耀的人工智能助手叫“YOYO”,也即悠悠;YOYO除了能够实现语音操控等基本功能之外,还拥有智慧视觉、智慧识屏、情景智能、智慧搜索等功能,可以在系统设置页面中的智慧助手里进行相关的设置。
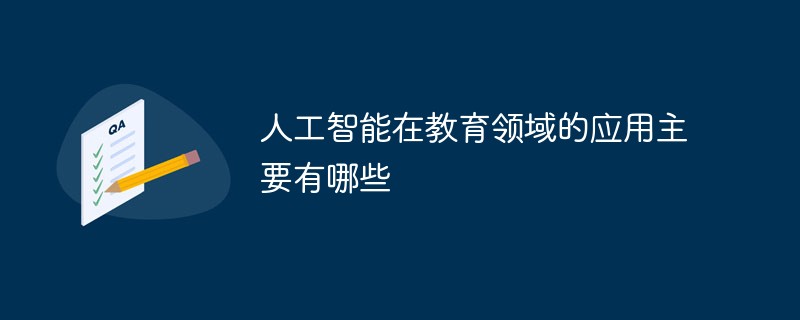
人工智能在教育领域的应用主要有个性化学习、虚拟导师、教育机器人和场景式教育。人工智能在教育领域的应用目前还处于早期探索阶段,但是潜力却是巨大的。
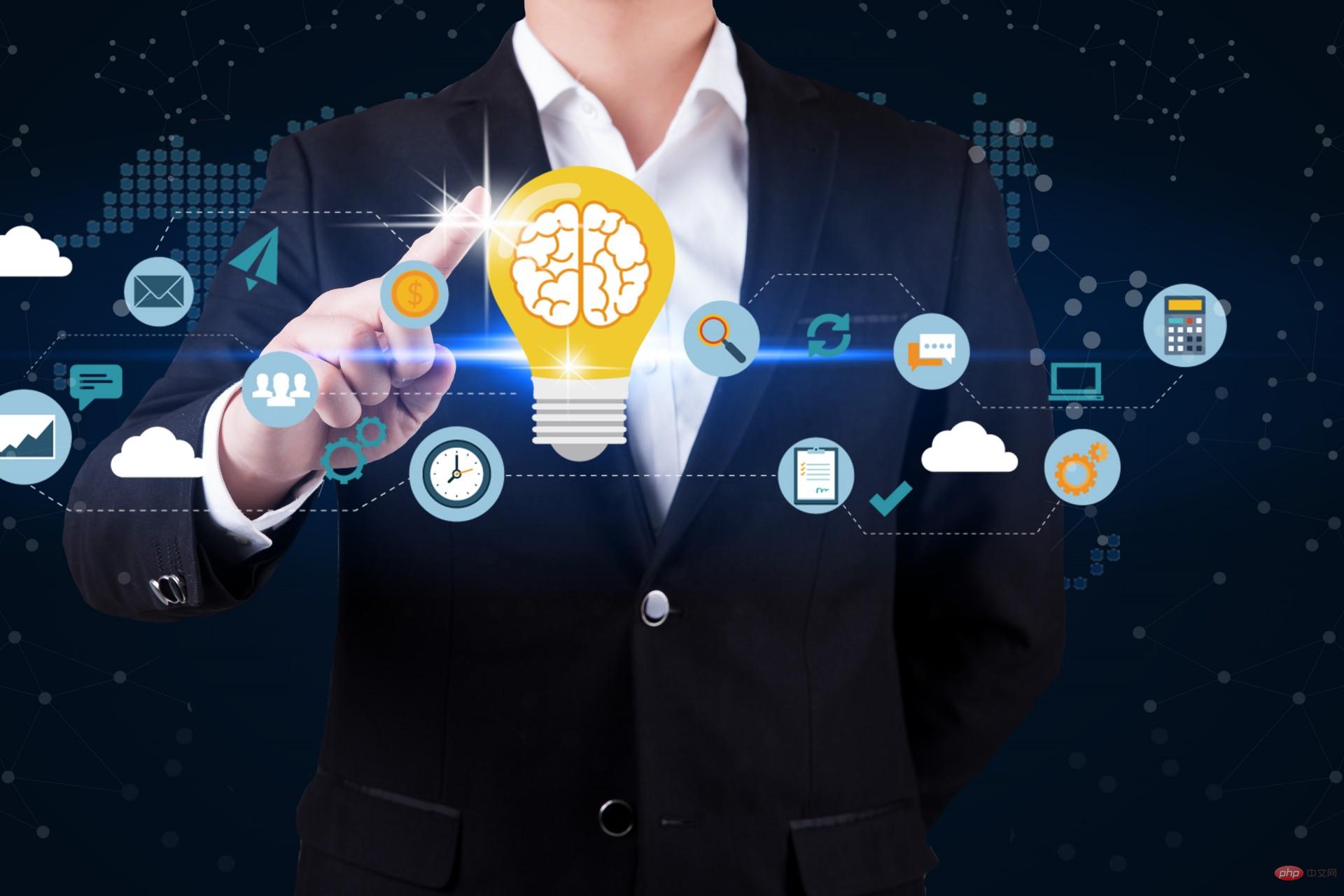
阅读论文可以说是我们的日常工作之一,论文的数量太多,我们如何快速阅读归纳呢?自从ChatGPT出现以后,有很多阅读论文的服务可以使用。其实使用ChatGPT API非常简单,我们只用30行python代码就可以在本地搭建一个自己的应用。 阅读论文可以说是我们的日常工作之一,论文的数量太多,我们如何快速阅读归纳呢?自从ChatGPT出现以后,有很多阅读论文的服务可以使用。其实使用ChatGPT API非常简单,我们只用30行python代码就可以在本地搭建一个自己的应用。使用 Python 和 C
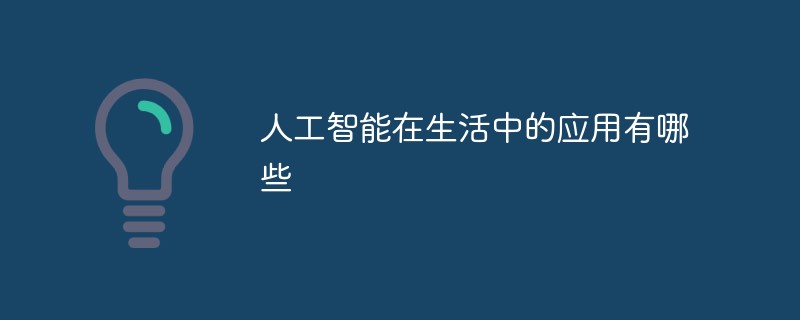
人工智能在生活中的应用有:1、虚拟个人助理,使用者可通过声控、文字输入的方式,来完成一些日常生活的小事;2、语音评测,利用云计算技术,将自动口语评测服务放在云端,并开放API接口供客户远程使用;3、无人汽车,主要依靠车内的以计算机系统为主的智能驾驶仪来实现无人驾驶的目标;4、天气预测,通过手机GPRS系统,定位到用户所处的位置,在利用算法,对覆盖全国的雷达图进行数据分析并预测。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
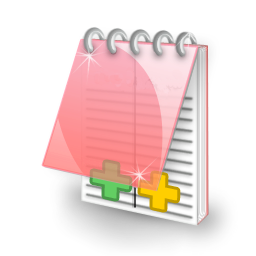
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!
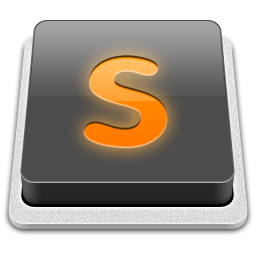
SublimeText3 Mac version
God-level code editing software (SublimeText3)
