Through the Go standard library database/sql package, you can connect to remote databases such as MySQL, PostgreSQL or SQLite: Create a connection string containing database connection information. Use the sql.Open() function to open a database connection. Perform database operations such as SQL queries and insert operations. Use defer to close the database connection to release resources.
How to connect to a remote database using Golang
Golang is a powerful programming language that can easily connect to a remote database. This tutorial will introduce how to use the Go standard library database/sql
package to connect to remote databases such as MySQL, PostgreSQL and SQLite.
Requirements
- Golang 1.16 or higher
- Remote database (such as MySQL, PostgreSQL or SQLite)
Connection String
First we need to create a connection string that contains the information needed to connect to the database. Here's how to create connection strings for different databases:
MySQL:
"user:password@tcp(host:port)/dbname"
PostgreSQL:
"user=username password=password host=address port=port dbname=database"
SQLite:
"path/to/sqlite.db"
Among them, user
, password
, host
, port
and dbname
is database specific.
Connect to the database
Use the database/sql
package to connect to the database:
import ( "database/sql" _ "github.com/go-sql-driver/mysql" // Import MySQL driver _ "github.com/lib/pq" // Import PostgreSQL driver _ "github.com/mattn/go-sqlite3" // Import SQLite driver ) func main() { // Create a connection string connStr := "user:password@tcp(host:port)/dbname" // Open the database connection db, err := sql.Open("mysql", connStr) if err != nil { panic(err) } defer db.Close() // Close the connection when the function returns }
Practical case
The following is a database using MySQL Simple example of:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" // Import MySQL driver ) func main() { // Connect to the database db, err := sql.Open("mysql", "root:@/test") if err != nil { panic(err) } defer db.Close() // Close the connection when the function returns // Create a table query := `CREATE TABLE IF NOT EXISTS users ( id INT PRIMARY KEY AUTO_INCREMENT, username VARCHAR(255) NOT NULL, password VARCHAR(255) NOT NULL );` _, err = db.Exec(query) if err != nil { panic(err) } // Insert a record into the table query = `INSERT INTO users (username, password) VALUES (?, ?)` stmt, err := db.Prepare(query) if err != nil { panic(err) } _, err = stmt.Exec("admin", "password") if err != nil { panic(err) } // Retrieve the record from the table query = `SELECT * FROM users WHERE id = ?` var id int var username string var password string err = db.QueryRow(query, 1).Scan(&id, &username, &password) if err != nil { panic(err) } fmt.Println("ID:", id, "Username:", username, "Password:", password) }
The above is the detailed content of How to connect to remote database using Golang?. For more information, please follow other related articles on the PHP Chinese website!
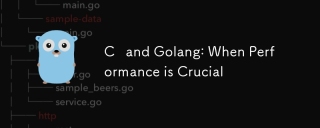
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
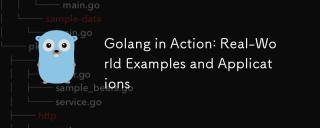
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
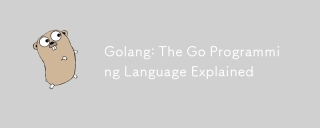
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
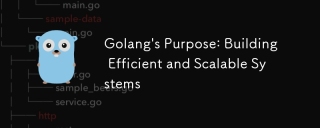
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
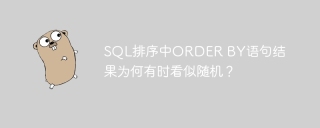
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
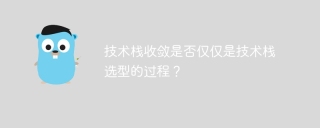
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
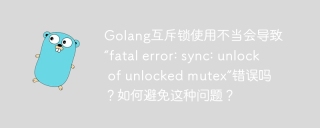
Golang ...
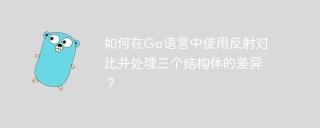
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
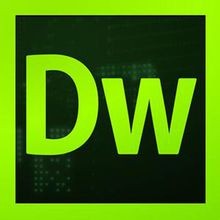
Dreamweaver CS6
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),