How to use exception handling in PHP?
Exception handling in PHP allows handling unexpected errors and improves code stability. To throw an exception, use the throw keyword, and to catch an exception, use the try...catch structure. Best practices include throwing only critical errors, providing friendly error messages, and using logging. Practical case: The function that calculates the quotient handles the case where the divisor is zero by throwing DivisionByZeroException.
Exception Handling in PHP
Exception handling is an important feature in PHP that allows you to handle An unexpected error occurred. By using exceptions, you can provide friendly and meaningful error messages to users and prevent your application from crashing.
How to throw an exception
To throw an exception, use PHP's built-in throw
keyword. It accepts an object that implements the Throwable
interface as a parameter. The following is an example of throwing an InvalidArgumentException
exception:
<?php throw new InvalidArgumentException("无效的参数"); ?>
How to catch an exception
To catch an exception, use try... catch
structure. The try
block contains code that may throw exceptions, while the catch
block is used to catch and handle exceptions:
<?php try { // 可能抛出异常的代码 } catch (InvalidArgumentException $e) { // 捕获 InvalidArgumentException 异常并进行处理 }
You can do this in a try
To catch multiple exceptions in a block, use multiple catch
blocks:
<?php try { // 可能抛出异常的代码 } catch (InvalidArgumentException $e) { // 捕获 InvalidArgumentException 异常并进行处理 } catch (OutOfRangeException $e) { // 捕获 OutOfRangeException 异常并进行处理 }
Best Practices
When using exception handling, please follow the following best practices Best practice:
- Only throw serious and unrecoverable errors. Small errors or warnings should not be converted to exceptions.
- Provide meaningful and user-friendly error messages. Help users understand errors and take appropriate action.
- Use logging to record exceptions. This will help you debug problems and identify patterns.
Practical Case
Suppose you have a function that calculates the quotient of two numbers. If the dividend is 0, the function should throw a DivisionByZeroException
exception. The following is the implementation of the function:
<?php function divide($numerator, $denominator) { if ($denominator == 0) { throw new DivisionByZeroException("除数不能为 0"); } return $numerator / $denominator; } ?>
When using this function, you can use the try...catch
structure to catch and handle exceptions:
<?php try { $result = divide(10, 2); } catch (DivisionByZeroException $e) { echo "除数不能为 0"; } ?>
The above is the detailed content of How to use exception handling in PHP?. For more information, please follow other related articles on the PHP Chinese website!
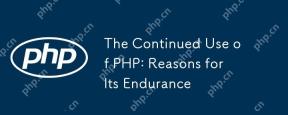
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
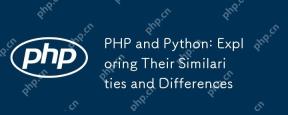
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
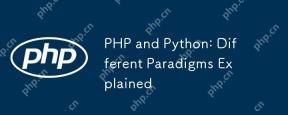
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
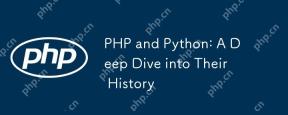
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
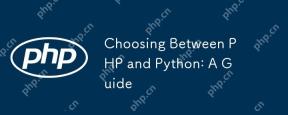
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
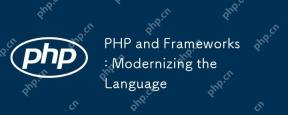
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
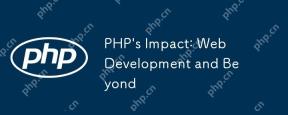
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
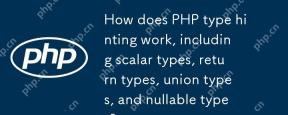
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
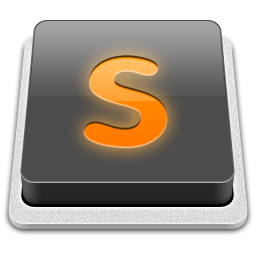
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor