


Golang network programming: building powerful server and client applications
Go Introduction to Network Programming Network Programming Basics: Understand the client-server model, TCP/IP protocol stack, and common network programming libraries. Build a web server: Use the net/http package to create an HTTP server that handles client requests and generates responses. Build a network client: Use the net/http package to create an HTTP client that sends requests and handles server responses. Practical example: Build a simple HTTP server and a client that sends requests and prints responses.
Go network programming: building powerful server and client applications
In today's digital world, network programming has become essential for developing modern software applications. Few skills. The Go language, known for its concurrency, built-in network support, and powerful standard library, is ideal for network programming. This article will guide you in using Go to build efficient server and client applications, with practical examples.
Network Programming Basics
Before you start building web applications, it is important to understand the basic concepts of network programming. Client-server model, TCP/IP protocol stack and common network programming libraries are essential knowledge.
Building a network server
The Go language provides the net/http package to simplify server development. You can build a simple HTTP server by following these steps:
package main import ( "fmt" "net/http" ) func main() { // 监听端口 8080 http.ListenAndServe(":8080", nil) }
Using this server, you can handle HTTP requests from clients. By adding code in the HTTP handler, the response can be generated dynamically.
Building the network client
Building the network client requires the use of the net package in the Go language. The following is how to build an HTTP client using the net/http package:
package main import ( "fmt" "net/http" ) func main() { // 创建HTTP客户端 client := &http.Client{} // 向服务器发送HTTP请求 resp, err := client.Get("http://localhost:8080") // 检查错误并处理响应 if err != nil { fmt.Println(err) return } // 读取服务器响应 body, err := ioutil.ReadAll(resp.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
Practical Case
Now let's build a practical case that contains a simple HTTP server and A client that sends a request to the server and prints the response.
Server
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Hello from the server!") }) http.ListenAndServe(":8080", nil) }
Client
package main import ( "fmt" "net/http" "io/ioutil" ) func main() { // 向服务器发送HTTP GET请求 resp, err := http.Get("http://localhost:8080") // 检查错误并处理响应 if err != nil { fmt.Println(err) return } // 读取服务器响应 body, err := ioutil.ReadAll(resp.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body)) }
Running Example
- Run the server:
go run server.go
- Run the client:
go run client.go
The client will send The server sends the request and prints the response: "Hello from the server!".
Conclusion
By using the Go language, you can easily build powerful web server and client applications. This guide provides a foundation to help you gain a deeper understanding of network programming, and comes with a practical example to further illustrate the concepts covered. With practice, you will be able to build a variety of complex web applications.
The above is the detailed content of Golang network programming: building powerful server and client applications. For more information, please follow other related articles on the PHP Chinese website!
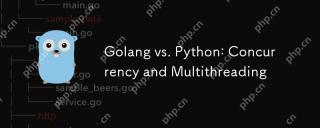
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
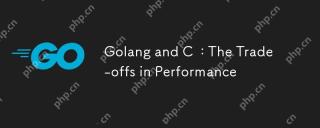
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
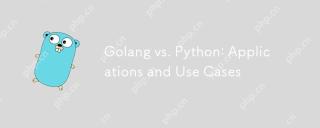
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
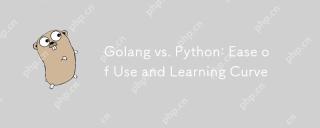
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
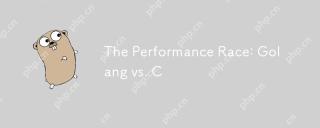
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
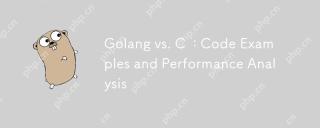
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
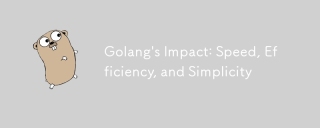
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
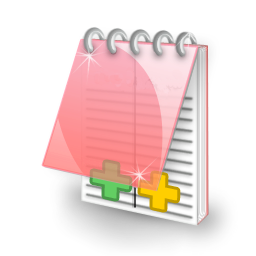
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.