


Golang error handling strategy: handle exceptions and failures gracefully
Strategy for handling exceptions and failures in Go language: Use fmt.Errorf to create a custom error object. Use errors.New to create short error messages. Provide detailed error tracing by creating error chains. Use Panic and Recovery to handle catastrophic errors. Create custom type errors to provide specific error information. Provides practical cases for handling file opening errors, demonstrating how to handle exceptions and failures in the Go language gracefully.
Go language error handling strategy: handle exceptions and failures gracefully
Introduction
In large and complex Go programs, error handling is crucial. The Go language provides a feature-rich error handling mechanism that enables developers to handle exceptions and failures gracefully. This article will explore error handling strategies in the Go language and illustrate them through practical cases.
fmt.Errorf
Use fmt.Errorf
to create an error object:
func main() { err := fmt.Errorf("open file: %v", os.ErrNotExist) }
errors.New
Create an error object with a short error message:
func main() { err := errors.New("open file failed") }
error chaining
Chain error objects together for more detailed errors Trace:
func main() { err := os.ErrNotExist err = fmt.Errorf("open file: %w", err) }
Exception handling (Panic and Recover)
Panic is a mechanism in the Go language to handle catastrophic errors. Recover can be used to recover from a Panic.
func main() { defer func() { if err := recover(); err != nil { // 处理 Panic } }() // 触发 Panic panic("file does not exist") }
Custom type error
Create a custom type error to provide more specific error information:
type FileNotExistError struct { path string } func (e FileNotExistError) Error() string { return fmt.Sprintf("file not found: %s", e.path) } func main() { err := FileNotExistError{path: "/home/user/file.txt"} }
Practical case: processing File open error
func openFile(path string) error { f, err := os.Open(path) if err != nil { switch { case os.IsNotExist(err): return fmt.Errorf("open file: file not found: %v", err) case errors.Is(err, io.EOF): return fmt.Errorf("open file: reached EOF unexpectedly: %v", err) default: return fmt.Errorf("open file: unknown error: %v", err) } } return nil } func main() { path := "/home/user/file.txt" err := openFile(path) if err != nil { log.Fatalf("open file error: %v", err) } }
Conclusion
The Go language provides a series of error handling functions that enable developers to handle exceptions and failures gracefully. You can create robust and easy-to-maintain Go programs by using fmt.Errorf
, errors.New
, error chaining, exception handling, and custom type errors.
The above is the detailed content of Golang error handling strategy: handle exceptions and failures gracefully. For more information, please follow other related articles on the PHP Chinese website!
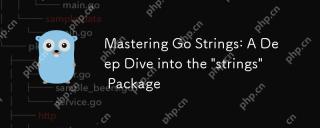
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
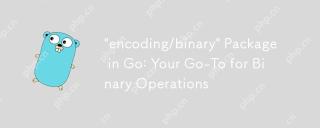
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
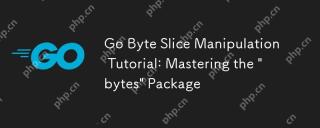
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
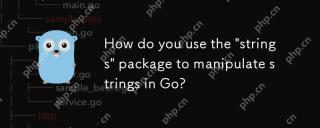
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
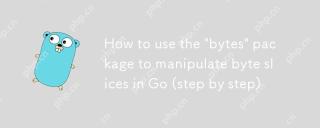
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
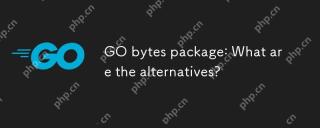
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
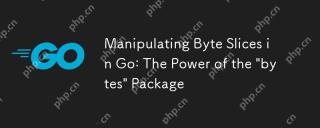
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
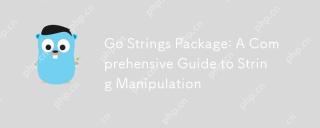
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
