What is an object?
From the definition of JavaScript, an object is a collection of unordered properties, and its properties can contain basic values, objects or functions. That is to say, an object is a set of attributes in no particular order. Each attribute is mapped to a value, which is a set of key-value pairs. The value can be data or an object.
The simplest object
A pair of curly brackets {} in JavaScript can define an object. This writing method is actually the same as calling the constructor of Object
var obj={};
var obj2= new Object();
The object constructed in this way only contains a pointer to the prototype of the Object. You can use some valueOf, hasQwnProperty and other methods, which have little practical effect. Custom objects always have some custom properties and methods.
var obj={};
=0; a:0,
fn:function( ){
alert(this);
You can add properties and methods to it through "." after defining the object, or you can use the literal assignment method to add properties and methods to it when defining the object. The object created in this way has the same methods as Attributes can directly use object references, similar to static variables and static functions of classes. There is an obvious flaw in creating objects in this way - it is very laborious to define a large number of objects, and it is necessary to write almost repetitive code over and over again.
Abstract it
Since it is repeated code, it can be abstracted, use functions to do these repeated tasks, and call a method specifically created when creating an object. For different attribute values, only You just need to pass in different parameters.
Copy code
The code is as follows:
obj={};
In this way, when creating a large number of objects, you can do some repetitive work by calling this method. This method is not perfect, because in many cases it is necessary to determine the type of the object. The objects created by the above code are the most The original Object object instance only extends some properties and methods.
Style
It’s time for function to appear again. In JavaScript, function is an object. When creating an object, you can discard the above createObj method and directly use function as an object. How to achieve reuse? This lies in the special nature of function as an object. Sex.
1. Function can accept parameters and can create objects of the same type and different values based on the parameters
2. When function is used as a constructor (called through the new operator), it will return an object. Some basic knowledge of constructors is mentioned in jQuery of the poor and lower-middle-class peasant version. Simply copy it
The return value of the constructor is divided into two situations. When the function has no return statement or returns a basic type (bool, int, string, undefined, null), it returns an anonymous object created by new, which is It is a function instance; if the function body returns a reference type object (Array, Function, Object, etc.), the object will overwrite the anonymous object created by new as the return value.
3. So how to use function to solve the type identification problem? Each function instance object will have a constructor attribute (not "has", but can correspond). This attribute can indicate who constructs it, or it can Use the instanceof operator to determine whether the object is an instance of XXX.
Don’t just talk without practicing, code
function Person(name){
this.name =name;
this.fn=function(){
alert(this.name);
}
}
var person1=new Person('Byron');
console.log(person1.constructor==Person);//true
console.log(person1 instanceof Person); //true
This would be perfect, no! Although the constructor can be object-specific, the methods must be repeated in every instance of the object!
function Person(name){
this.name =name;
this.fn=function(){
alert(this.name);
}
}
var person1=new Person('Byron');
var person2=new Person('Frank');
console.log(person1.fn==person2.fn);//false
Look, although the fn of the two instances are exactly the same, they are not the same thing. If a function object has a thousand methods, then each of its instances must contain copies of these methods, which is very frustrating. The memory is speechless.
No more nonsense
Is there a nearly perfect way to construct an object that does not require repeated work, is stylish, and does not require the repetition of common methods of objects? ? In fact, we can find that using function is already close to the requirements. There is only one difference - a container shared by all instances of function objects is needed. In this container, instances need to share attributes and methods. It just so happens that this container is ready - —prototype, students who don’t know prototype can take a look at JavaScript prototype
function Person(name){
this.name=name;
}
Person.prototype.share=[];
Person. prototype. printName=function(){
; ;
console.log(person1.printName==person2.printName);//true
In this way, each Person instance has its own attribute name, as well as the attribute share and method printName shared by all instances. The basic problems are solved. For general object processing, you can always create this stylish and loving way. Object mode.
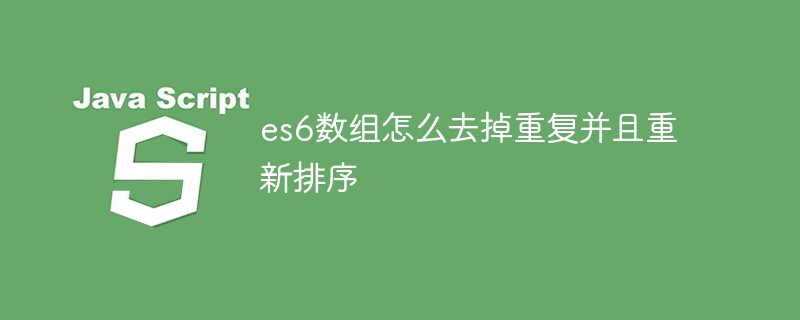
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
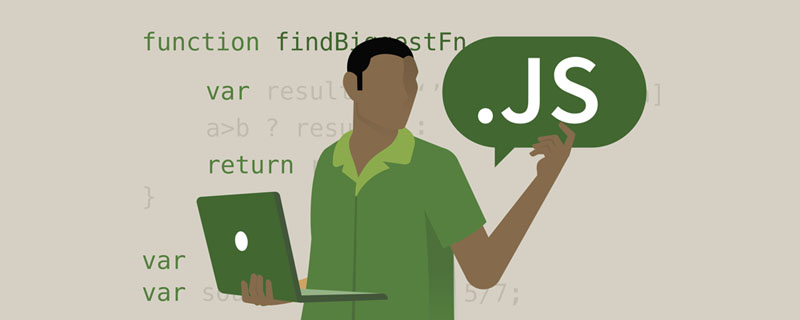
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
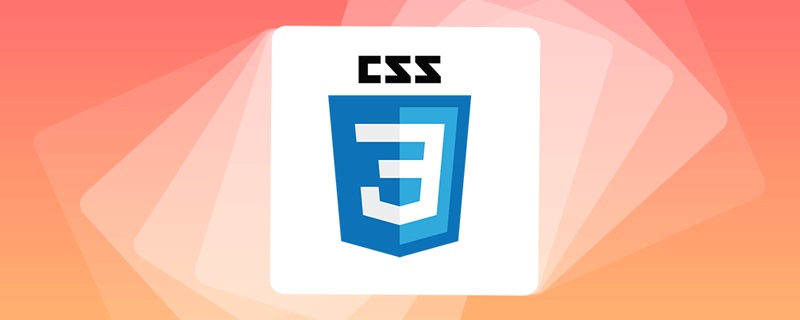
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
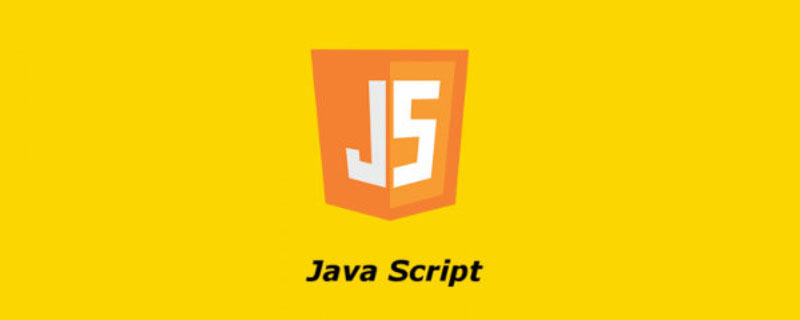
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
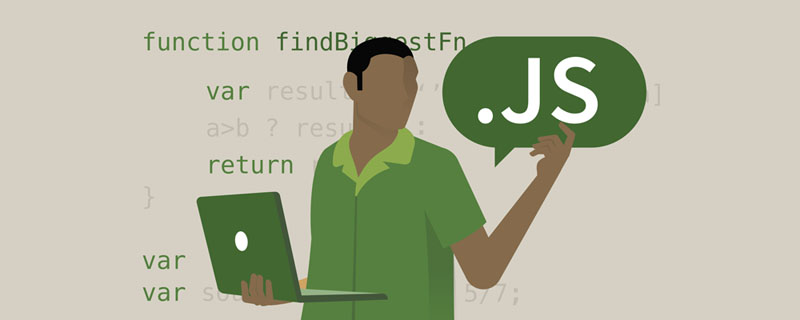
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
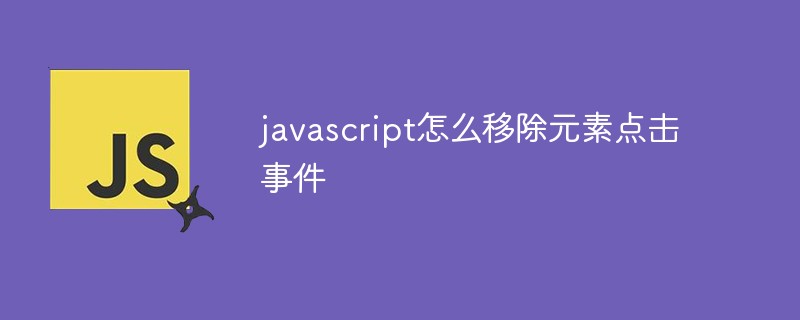
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
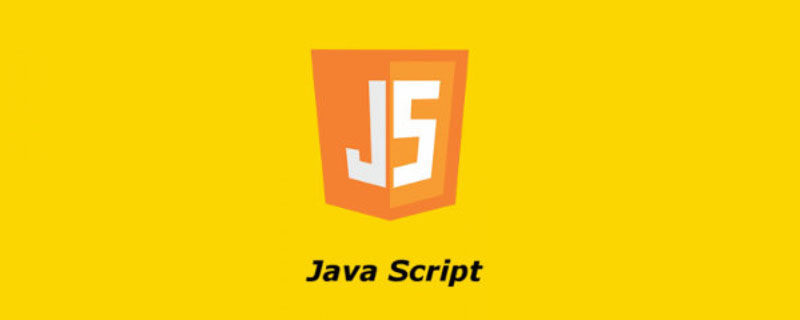
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
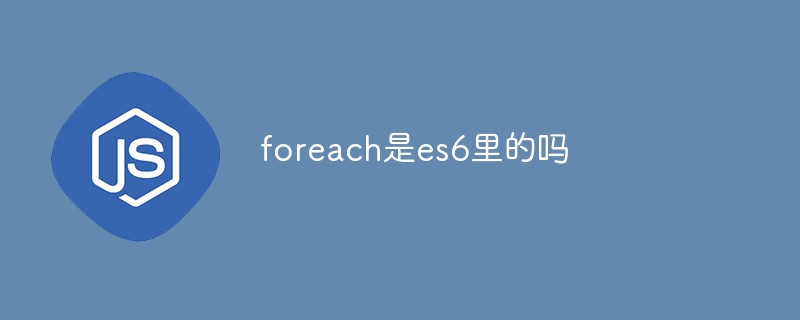
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
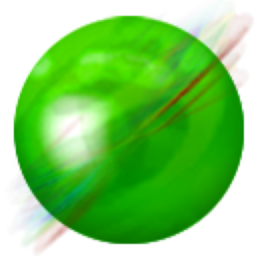
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
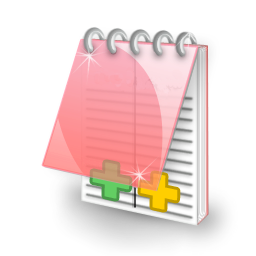
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
