Requirements
Drag sorting, as you can imagine from the name, is to press and hold a row of data and drag it to the desired sorting position, and save the new sorting queue.
Thoughts
First create an anchor point for the list row, bind the mousedown and mouseup events, when the mouse moves to the position you want to insert, move the object row to the target row, and then sort all the rows it passes.
The idea is very simple, but there are still several issues to pay attention to
1. The position to which you move can be regarded as the position to be inserted into the target row.
2. When moving out of the top and bottom, it is judged as the first and last.
3. Processing of moving up and moving down
Solution
About the event
The mouse press and release events in Javascript are onmousedown and onmouseup. In JQuery, they are mousedown and mouseup. Therefore, mousedown and mouseup are used here
First of all, you need to know how many lines there are in the interface and how high each line is, because you need to judge the movement distance of the mouse
var tbodyHeight=setting.frame.outerHeight(); //setting.frame, parent object
var lineNum=$("." setting.dgLine) .length; //setting.dgLine, classname of each line
var lineHeight=Math.ceil(tbodyHeight/lineNum);
All you need to do is get lineNum (the number of lines). In addition to calculating the line height, another purpose is to use index() to move the line to the target position through the sequence index value
When the mousedown event is triggered, it is necessary to start calculating the distance of the mouse movement, which is used to determine where the line should be moved
dgid=$(this).attr(setting.id ); //The ID of the mobile row, setting.id, is the name used to mark the ID in each row
thisIndex=$("#" setting.linePre dgid).index(); //The index of the row, setting .linePre, each line ID is off
thisLineTop=$("#" setting.linePre dgid).offset().top; //The top value of this line
topDistance=thisIndex*lineHeight; //This line The distance from the top of the first line
downDistance=(lineNum-thisIndex-1)*lineHeight; //The distance between this line and the bottom of the last line
dgid is mainly used to distinguish the identifier of each line. The general list is output by the program loop. Without such an ID, it is impossible to tell which line is which. Therefore, in HTML, You need to define a person to store the ID. The program uses attr to get this value, ensuring that each row has its own unique value.
thisLineTop is mainly used to calculate the height based on the mouse movement position, and then determine which line it has moved to based on the line height and index value. Another function is to determine whether the moving anchor point is pressed. If there is a value, it means yes, and the subsequent mouseup is established. If there is no value, it means the anchor point is not pressed, and mouseup does not perform any operation. Why do this? Because no matter where you click the mouse on the page, the mouseup event will be triggered. If there is no judgment, it will continue to execute, which will cause some problems.
topDistance and downDistance are used to determine whether the mouse has moved out of the list. If it has been removed, that is, the distance the mouse moves is greater than topDistance or downDistance, it can be judged that it needs to be moved to the first or last row.
The mousedown event mainly does these few things. Of course, for the sake of effect, you can also add some things
$("#" setting.linePre dgid).css ('background',setting.lineHighlight); //Highlight mobile lines
var left=e.pageX 20;
var top=e.pageY;
dg_tips(left,top); //Create A prompt layer
$('body').css('cursor','move'); //Change the mouse gestures of the page
$("body").disableSelection(); //Disable pressing Select the page element when the mouse is moved behind the mouse
setting.frame.mousemove(function(e){ //Let the prompt layer follow the mouse movement
$("#dgf").css({"left":e. pageX setting.tipsOffsetLeft 'px',"top":e.pageY 'px'});
});
The purpose of these is just to make the operation more effective. For example, highlighting a row is to let the user know which row they are operating on. The prompt layer works the same way.
Regarding disabling selection, .disableSelection(); this is a method included in jQuery_UI. If you are using jQuery_UI, you can use it directly. If not, you can do this
$('body').each(function() { select':'none',
'-webkit-user-select':'none',
'user-select':'none'
}).each(function() {
this.onselectstart = function() { return false; };
});
});
});
});
Considering versatility, .disableSelection();
will not be used in the following code.
Okay, here is the mouseup event. The mouseup event here is bound to the body, because if the mouseup is only bound to the anchor point, when the mouse moves out of the anchor point and then releases the mouse, you will find that the mouseup event will not be executed because it will think It is a mouseup of other objects. Therefore, the safest way is to use $('body').mouseup. Basically there will be no problems.
var moveDistance=e.pageY-thisLineTop;
Do different processing according to different directions
Copy code
focusIndex=0;
}else{
focusIndex=thisIndex-Math.ceil( moveDistance/lineHeight);
}
$("." setting.dgLine).eq(focusIndex).before($("#" setting.linePre dgid));//Insert the line into the target position
}
}
}else{
if(thisIndex!=lineNum-1){
if(moveDistance>lineHeight/2 lineHeight){
if(moveDistance>downDistance){
focusIndex=lineNum-1;
}else{
focusIndex=thisIndex Math.ceil(moveDistance/lineHeight)-1;
}
$("." setting.dgLine).eq( focusIndex).after($("#" setting.linePre dgid));
}
}
}
The reason why it is judged whether the movement distance exceeds 1/2 of the row height is because if it only moves a small point, it can be regarded as not moving. When calculating the target index value, Math.ceil is used, and the carry is the most. When the moving distance is greater than 0, the carry is -1, because it is downward.
Moving up and moving down use different insertion methods, before and after. You can try to think about why before and after are used.
Copy code
Basically this is the situation, the main problem is in handling movement and determining where to insert. Everything else is very simple.
The complete package program is given below, including the update data part
/*
*
* DragList.js
* @author fuweiyi
*
*/
(function($){
$.fn.DragList=function(setting){
var _setting = {
frame : $(this),
dgLine : 'DLL',
dgButton : 'DLB',
id : 'action-id',
linePre : 'list_',
lineHighlight : '#ffffcc',
tipsOpacity : 80,
tipsOffsetLeft : 20,
tipsOffsetTop : 0,
JSONUrl : '',
JSONData : {},
maskLoaddingIcon : '',
maskBackgroundColor : '#999',
maskOpacity : 30,
maskColor : '#000',
maskLoadIcon:'',
};
var setting = $.extend(_setting,setting);
var dgid='',thisIndex,thisLineTop=0,topDistance,downDistance;
var tbodyHeight=setting.frame.outerHeight();
var lineNum=$("."+setting.dgLine).length;
var lineHeight=Math.ceil(tbodyHeight/lineNum);
$("."+setting.dgButton).mousedown(function(e){
dgid=$(this).attr(setting.id);
thisIndex=$("#"+setting.linePre+dgid).index();
var left=e.pageX+20;
var top=e.pageY;
thisLineTop=$("#"+setting.linePre+dgid).offset().top;
topDistance=thisIndex*lineHeight;
downDistance=(lineNum-thisIndex-1)*lineHeight;
$("#"+setting.linePre+dgid).css('background',setting.lineHighlight);
dg_tips(left,top);
$('body').css('cursor','move');
unselect();
setting.frame.mousemove(function(e){
$("#dgf").css({"left":e.pageX+setting.tipsOffsetLeft+'px',"top":e.pageY+'px'});
});
});
$('body').mouseup(function(e){
if(thisLineTop>0){
var moveDistance=e.pageY-thisLineTop;
if(moveDistance if(thisIndex!=0){
moveDistance=Math.abs(moveDistance);
if(moveDistance>lineHeight/2){
if(moveDistance>topDistance){
focusIndex=0;
}else{
focusIndex=thisIndex-Math.ceil(moveDistance/lineHeight);
}
$("."+setting.dgLine).eq(focusIndex).before($("#"+setting.linePre+dgid));
dg_update(thisIndex,focusIndex);
}
}
}else{
if(thisIndex!=lineNum-1){
if(moveDistance>lineHeight/2+lineHeight){
if(moveDistance>downDistance){
focusIndex=lineNum-1;
}else{
focusIndex=thisIndex+Math.ceil(moveDistance/lineHeight)-1;
}
$("."+setting.dgLine).eq(focusIndex).after($("#"+setting.linePre+dgid));
dg_update(thisIndex,focusIndex);
}
}
}
$("#dgf").remove();
$("#"+setting.linePre+dgid).css('background','');
dgid='';
thisLineTop=0;
$('body').css('cursor','default');
onselect();
}
});
function dg_update(thisIndex,focusIndex){
dg_mask();
var start=thisIndex
for(var i=start;i ids+=i==start?$("."+setting.dgLine).eq(i).attr(setting.id):','+$("."+setting.dgLine).eq(i).attr(setting.id);
vals+=i==start?i:','+i;
}
$.getJSON(setting.JSONUrl,{'do':'changeorders','ids':ids,'vals':vals},function(d){
$("#dg_mask").remove();
});
}
function dg_mask(){
var W=setting.frame.outerWidth();
var H=setting.frame.outerHeight();
var top=setting.frame.offset().top;
var left=setting.frame.offset().left;
var mask=" 正在使劲的保存...
$('body').append(mask);
$("#dg_mask").css({"background":"#999","position":'absolute','width':W 'px','height':H 'px','line-height':H 'px','top':top 'px','left':left 'px','filter':'alpha(opacity=' setting.maskOpacity ')','moz-opacity':setting.maskOpacity/100,'opacity':setting.maskOpacity/100,'text-align':'center','color':'#000'});
}
function dg_tips(left,top){
var floatdiv="
$('body').append(floatdiv);
}
function unselect(){
$('body').each(function() {
$(this).attr('unselectable', 'on').css({
'-moz-user-select':'none',
'-webkit-user-select':'none',
'user-select':'none'
}).each(function() {
this.onselectstart = function() { return false; };
});
});
}
function onselect(){
$('body').each(function() {
$(this).attr('unselectable', '').css({
'-moz-user-select':'',
'-webkit-user-select':'',
'user-select':''
});
});
}
}
})(jQuery);
使用
拖动 | 名称 |
![]() |
这里是一行 |
参数主要是dgLine,dgButton,id,linePre和JSONUrl,通过看HTML代码,应该不难理解。
关于拖动排序就是这么多了,当然还可以把效果做得更漂亮些,提示更清楚点,有助于用户体验
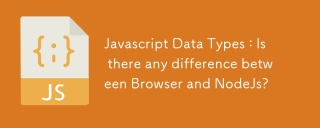
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
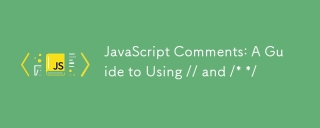
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
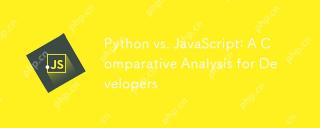
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
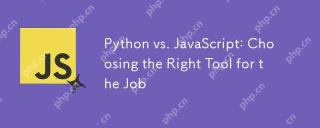
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
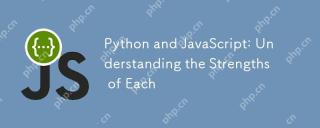
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
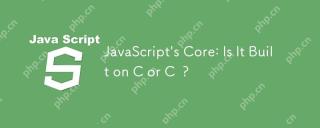
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
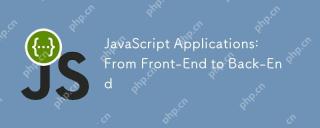
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
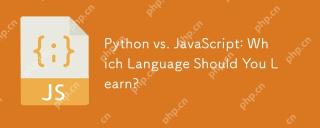
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
