Closure is a very important feature of ECMAScript, but it is difficult to describe it with a suitable definition. Although closures are difficult to describe clearly, they are easy to create, or accidentally create. However, the existence of closures actually has certain potential problems. In order to avoid "accidentally" creating closures and to better take advantage of their advantages, it is necessary to understand the mechanics of closures.
Definition of closure
Regarding closure, there are too many definitions, especially some definitions are very abstract, like this one:
A "closure" is an expression (typically a function) that can have free variables together with an environment that binds those variables.
Roughly speaking, closure is an expression that has some free variables and binds these variables execution environment. This definition is too literal and difficult to understand.
There is another definition:
All functions are closures. This definition confuses me a lot. In other words, since Javascript does not have block-level scope, closures generally refer to functions (I can’t think of any other ways to form closures other than functions).
I don’t want to discuss too much about the relationship between functions and closures here. Let’s give a definition that I think is easier to understand.
First of all, the existence of closures is based on the scope chain. Due to the scope chain mechanism, all functions (even global functions) can reference variables in the context execution environment (ie, free variables).
Secondly, there must be free variables inside the closure. By the way, there are two types of variables 1. Local variables (bound variables) 2. Non-local variables (free variables)
Finally, they still exist after their context ends. That is, the inner function has a longer life cycle than its outer function.
About the analysis of closure definition
Regarding the two points of closure definition, I have been considering whether they must be satisfied at the same time.
First of all, if there are no free variables inside the closure, that is to say, it does not access external variables, then the meaning of the closure is lost. (Unless the behavior is changed by other closures) Therefore, I think free variables are a requirement.
Secondly, if there are free variables inside the function, but when its context is destroyed, it will also be destroyed. It can be imagined that although the internal function accesses its external function variable, it is also recycled after the external function is executed. In this case, the discussion of closures is meaningless.
Let’s look at two examples:
var objectA = (function() {
var localA = "localA";
innerFn();
// 单纯的内部函数调用
function innerFn() {
localA = "innerChange";
}
return {
getLocalA : function() {
return "empty";
}
};
})();
objectA.getLocalA();
objectA.getLocalA = function() {
return localA;
};
//console.log(objectA.getLocalA()); //error: localA is not defined
var objectB = (function() {
var localB = "localB";
return {
getLocalB : function() {
return "empty";
},
updateGetLocalB : function() {
this.getLocalB = function() {
return localB;
};
},
updateLocalB : function() {
localB = "changeLocalB";
}
};
})();
console.log(objectB.getLocalB()); // empty
// 通过其他闭包改变
objectB.updateGetLocalB();
console.log(objectB.getLocalB()); // localB
objectB.updateLocalB();
console.log(objectB.getLocalB()); // changeLocalB
闭包的优点和缺点
闭包的优点是闭包内部可以访问到定义它们的外部函数的参数和变量(除了this和arguments)。
闭包主要的问题便是它会保存包含它的函数的作用域,因此比一般函数占用更多的内存空间,因此不宜过度使用闭包。
闭包的应用
闭包最基本的应用场景便是通过保护内部变量从而实现私有,比如模块模式。
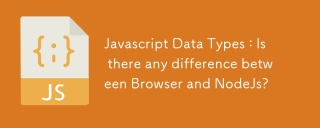
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
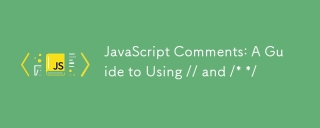
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
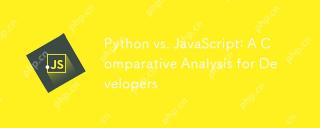
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
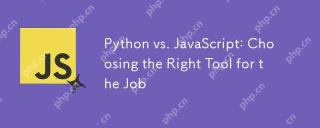
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
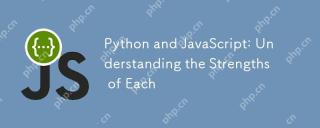
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
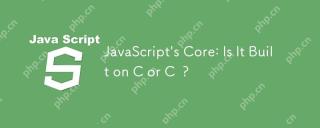
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
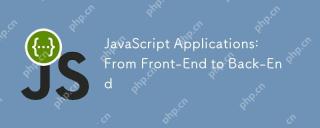
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
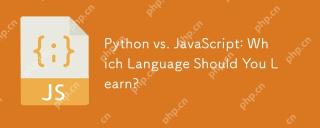
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
