How to customize Grunt tasks
Sometimes we need to write some of our own grunt tasks, here is a specific example
1. Preparation
1. Create a new directory g1
2. Create a new package.json and put it in g1
3. Create a new Gruntfile.js and put it in g1
package.json
{
"name": "g1",
"version": "0.1.0",
"author": "@snandy",
"homepage": "http://www.g1.com",
"devDependencies" : {
"grunt": "~0.4.0"
}
}
4. cd into g1, npm install to install the grunt package
The entire directory structure is as follows
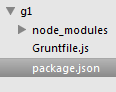
Gruntfile.js is temporarily empty.
2. Create the simplest task
grunt.registerTask(taskName, [description,] taskFunction)
taskName task name, use grunt in the command line taskName
description description of the task
taskFunction implementation of the task
Fill in the code in Gruntfile.js
module.exports = function(grunt) {
grunt.registerTask('mytask', 'A simplest task demonstration, print different outputs according to parameters.', function(arg1, arg2) {
.log.writeln('Task' this.name ", no parameters passed");
} else if (arguments.length === 1) {
grunt.log.writeln('Task' this.name ", one parameter is " arg1);
} else {
grunt.log.writeln('task' this.name ", there are two parameters are " arg1 ", " arg2);
}
});
};
Registered a task "mytask" to implement the simplest way to achieve different print outputs based on different passed parameters. To see the running results, we need to enter the command line.
Go to the g1 directory and enter grunt mytask
Enter grunt mytask:snandy
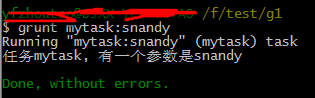
Add a colon after the task name to pass parameters
Enter grunt mytask:snandy:backus
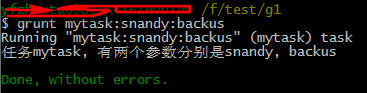
You can pass multiple parameters separated by colon
3. Create multiple tasks at one time
grunt.registerMultiTask(taskName, [description,] taskFunction)
You can see that the parameters are the same but the method names are different. But the usage method is different. You need to initialize the config first. Gruntfile.js is as follows
module.exports = function(grunt) {
grunt.initConfig({
log: {
t1: [1, 2, 3],
t2: 'hello world',
t3: false
}
});
grunt.registerMultiTask('log', 'Log stuff.', function() {
grunt.log.writeln(this.target ': ' this.data);
});
};
Enter the g1 directory and test separately
Enter grunt, and three subtasks t1, t2, t3 will be executed in sequence
Enter grunt log:t1, grunt log:t2, grunt log:t3 respectively
4. Communication between tasks
You can call another task within a task, as follows
Copy code The code is as follows:
module.exports = function(grunt) {
grunt.registerTask('mytask', 'The simplest task demonstration, printing different outputs according to parameters.', function(arg1, arg2) {
if (arguments.length === 0) {
grunt.log.writeln('task' this.name ", no parameters passed");
} else if (arguments.length === 1) {
grunt.log.writeln('Task' this.name ", one parameter is " arg1); .name ", there are two parameters: " arg1 ", " arg2);
}
});
grunt.registerTask('default', 'Default task', function( ) {
Enter the command line and type grunt
To call multiple tasks, just pass them to the run method separated by commas, or in array form
Copy code
The code is as follows: