


JavaScript method to determine whether a variable is an object or an array_javascript skills
typeof all returns object
All data types in JavaScript are objects in the strict sense, but in actual use we still have types. If you want to determine whether a variable is an array or an object, you can’t use typeof because it all returns object
var o = { 'name':'lee' };
var a = ['reg','blue'];
document.write( ' o typeof is ' typeof o);
document.write( '
');
document.write( ' a typeof is ' typeof a);
Execution:
o typeof is object
a typeof is object
Therefore, we can only give up this method. There are two ways to determine whether it is an array or an object
First, use typeof plus length attribute
Array has length attribute, object does not, and typeof array and object both return object, so we can judge this way
var o = { 'name':'lee' };
var a = ['reg','blue'];
var getDataType = function(o){
If(typeof o == 'object'){
If( typeof o.length == 'number' ){
return 'Array';
}else{
return 'Object'; }
}else{
return 'param is no object type';
}
};
alert( getDataType(o) ); // Object
alert( getDataType(a) ); // Array
alert( getDataType(1) ); // param is no object type
alert( getDataType(true) ); // param is no object type
alert( getDataType('a') ); // param is no object type
Second, use instanceof
Use instanceof to determine whether a variable is an array, such as:
var a = ['reg','blue'];
alert( a instanceof Array ); // true
alert( o instanceof Array ); // false
var a = ['reg','blue'];
alert( a instanceof Object ); // true
alert( o instanceof Object ); // true
var a = ['reg','blue'];
var getDataType = function(o){
If(o instanceof Array){
return 'Array'
}else if( o instanceof Object ){
return 'Object';
}else{
return 'param is no object type';
}
};
alert( getDataType(o) ); // Object
alert( getDataType(a) ); // Array
alert( getDataType(1) ); // param is no object type
alert( getDataType(true) ); // param is no object type
alert( getDataType('a') ); // param is no object type
If you don’t judge Array first, for example:
var o = { 'name':'lee' };
var a = ['reg','blue'];
var getDataType = function(o){
If(o instanceof Object){
return 'Object'
}else if( o instanceof Array ){
return 'Array';
}else{
return 'param is no object type';
}
};
alert( getDataType(o) ); // Object
alert( getDataType(a) ); // Object
alert( getDataType(1) ); // param is no object type
alert( getDataType(true) ); // param is no object type
alert( getDataType('a') ); // param is no object type
Then the array will also be judged as object.
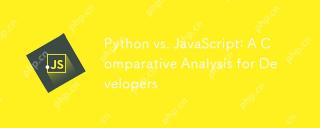
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
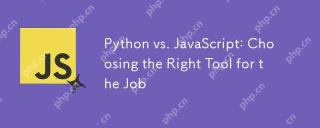
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
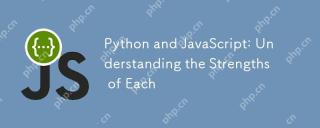
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
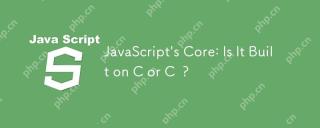
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
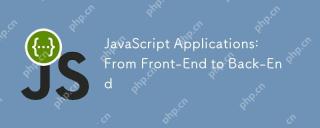
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
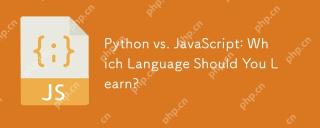
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
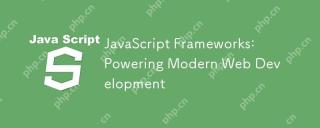
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
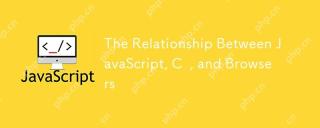
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
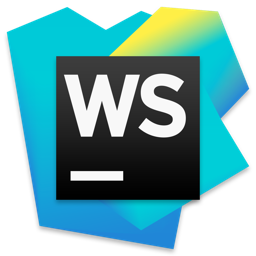
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
