Study notes on call and apply in Javascript_javascript skills
Let’s first look at the explanation of call in MDN
The call() method calls a function or method using a specified this value and several specified parameter values.
Note: The function of this method is similar to the apply() method. The only difference is that the call() method accepts a list of several parameters, while the apply() method accepts an array containing multiple parameters.
Grammar
fun.call(thisArg[, arg1[, arg2[, ...]]])
Parameters
thisArg
The this value specified when the fun function is running. It should be noted that the specified this value is not necessarily the real this value when the function is executed. If the function is in non-strict mode, the this value specified as null and undefined will automatically point to the global object (in the browser, it is window object), and this whose value is a primitive value (number, string, Boolean value) will point to the automatic wrapping object of the primitive value.
arg1, arg2, ...
The specified parameter list.
The examples on MDN were not easy to understand at first, so I posted them here. If you are interested, you can check them out for yourself call-Javascript
The thisArg here is interpreted as the this value specified when fun is running. That is to say, after using call, this in fun points to thisArg? Look at the code
var p="456"; function f1(){ this.p="123"; } function f2() { console.log(this.p); } f2(); //456 f2.call(f1()); //123 f2.apply(f1()); //123
The first output is the global variable of the call. Later, due to the use of call and apply, this in f2 points to f1, so the output becomes 123. In fact, f1 borrows the method of f2 and outputs its own p
At this time, delete this.p in f1(), and three 456s will be output, which confirms that when this is null or undefined, it actually points to the global variable
As for pointing to the original value, it points to its packaging object. Since the packaging objects I understand are temporary, and the test only outputs the type of the original value instead of object, how can I prove it here? If anyone knows, I hope you can Discuss it with me, thank you!
Since call can realize one object borrowing another object, can’t it also realize inheritance? Look at the code
function f1(){ this.father="father" } function f2() { f1.call(this); this.child="child"; } var test=new f2(); console.log(test.father); //father
There is no father in test, because of the
in f2()f1.call(this);
This here points to f2, which means that f2 borrows the method of f1, which actually realizes inheritance
Let’s talk about the parameters here. The parameters here are passed to fun. Let’s look at the code
function f1(){ this.p="123"; } function f2(x) { console.log(this.p); console.log(x); } f2.call(f1(),456); //123 //456
The first output is 123 because of the p in f1, and the subsequent 456 is the parameter passed to f2. It is easy to understand
The main thing is to pay attention to the difference between the parameters in call and apply
call is passed in one by one, and apply is passed in an array
function f1(){ this.p="测试call"; } function f2(x,y,z) { console.log(this.p); console.log(x); console.log(y); console.log(z); } function f3(){ this.p="测试apply"; } f2.call(f1(),4,5,6); f2.call(f1(),[4,5,6]); f2.apply(f3(),[4,5,6]); f2.apply(f3(),4,5,6);
You can see the results here
The first test call is output correctly
Since the second test call is passed in an array, it first outputs an array and then two undefined
The third section of test apply outputs correctly
The fourth paragraph directly reports an error due to incorrect parameter format
The difference here should be obvious
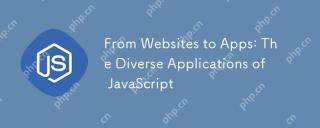
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
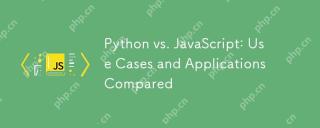
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
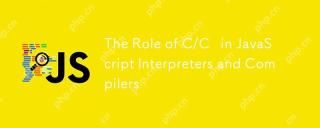
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
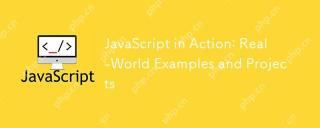
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
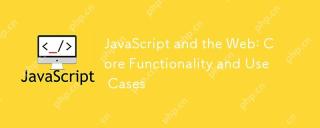
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
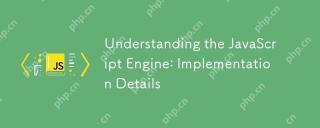
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
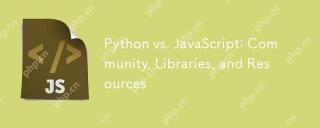
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
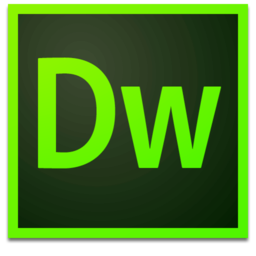
Dreamweaver Mac version
Visual web development tools
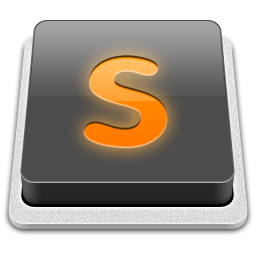
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
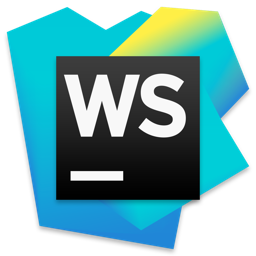
WebStorm Mac version
Useful JavaScript development tools