Using mongoskin to operate mongoDB instances in Node.js_node.js
1. Nonsense
Since January 2013, I have been exposed to mongodb for development and developed travel tag services, Weibo tag retrieval systems, map services, and web APP services... The scenario of using MongoDB has been transferred from .NET and JAVA environments to the node.js platform. . The more I find that the combination of Node.js and mongodb feels very good. It feels like mongodb and node.js are a natural match. Indeed, the client of mongodb is the parsing engine of JS. Therefore, choosing mongodb and node.js for product prototypes is also a very nice choice. On the Internet, I met netizens asking about which driver is best for mongodb development. I have always used the native driver before, but there are many things to pay attention to when writing code, such as the closing operation of the connection, etc... Therefore, in node.js In the development environment, I recommend using mongoskin.
2. Several concepts that need to be discussed
(1) Database: Same as relational database.
(2) Set: Table in a relational database.
(3) Document: Analogous to the records of a relational database, it is actually a JSON object.
(4) Database design: It is recommended to consider NoSQL design and abandon the design ideas of relational data; in fact, NoSQL database design is broad and profound and needs to be continuously practiced in projects.
(5) User system: Each database has its own administrator, who can:
use dbname; db.addUser('root_1' , 'test');
(7) It is recommended to change the external port
(8) Start the service (this is under win, slightly modified under Linux):
mongod --dbpath "XXMongoDBdatadb" --logpath "XXMongoDBlogmongo.log" --logappend -auth --port 7868
3. Build mongodb development infrastructure
(0) npm install mongoskin Install mongoskin
Node.js installation, package and other mechanisms are not introduced here.
(1) Create configuration file config.json
{
"dbname":"TEST",
"port": "7868",
"host": "127.0.0.1",
"username": "test",
"password": "test"
}
(2) Create util related class mongo.js: export a DB object
var mongoskin = require('mongoskin'),
config = require('./../config.json');
/*
* @des: Export database connection module
* */
module.exports = (function(){
var host = config.host,
port = config.port,
dbName = config.dbname,
userName = config.username,
Password = config.password,
str = 'mongodb://' userName ':' password '@' host ':' port '/' dbName;
var option = {
native_parser: true
};
return mongoskin.db(str, option);
})();
(3) Build the basic class of CRUD: In order to reduce repeated CURD code, you only need to pass in the relevant JSON object
var db = require('./mongo.js'),
Status = require('./status'),
mongoskin = require('mongoskin');
var CRUD = function(collection){
This.collection = collection;
db.bind(this.collection);
};
CRUD.prototype = {
/*
* @des: Create a record
* @model: Inserted record, model in JSON format
* @callback: callback, returns successfully inserted records or failure information
*
* */
Create: function(model, callback){
db[this.collection].save(model, function(err, item){
if(err) {
return callback(status.fail);
}
Item.status = status.success.status;
Item.message = status.success.message;
return callback(item);
});
},
/*
* @des: Read a record
* @query: Query conditions, JSON literal for Mongo query
* @callback: callback, returns records that meet the requirements or failure information
*
* */
Read: function(query, callback){
db[this.collection].find(query).toArray(function(err, items){
if(err){
return callback(status.fail);
}
var obj = {
status: status.success.status,
message: status.success.message,
items: items
};
return callback(obj);
});
},
/*
* @des: Update a record
* @query: Query condition, JSON literal of Mongo query, here is _id
* @updateModel: Model in JSON format that needs to be updated
* @callback: Return success or failure information
*
* */
Update: function(query, updateModel, callback){
var set = {set: updateModel};
db[this.collection].update(query, set, function(err){
if(err){
return callback(status.fail);
}else{
return callback(status.success);
}
});
},
/*
* @des: Delete a record
* @query: Query conditions, JSON literal for Mongo query
* @callback: Return failure or success information
*
* */
deleteData: function(query, callback){
db[this.collection].remove(query, function(err){
if(err){
return callback(status.fail);
}
return callback(status.success);
});
}
};
module.exports = CRUD;
(4) Build status.json, because some status is needed to indicate success and failure. It can be expanded to include verification code errors, SMS verification errors, username errors, etc.
module.exports = {
/*
*Success status
*
* */
Success: {
status: 1,
message: 'OK'
},
/*
* Failed status
*
* */
fail: {
status: 0,
message: 'FAIL'
},
/*
* The passwords entered twice are inconsistent
* */
RepeatPassword: {
status: 0,
message: 'The passwords entered twice are inconsistent'
}
};
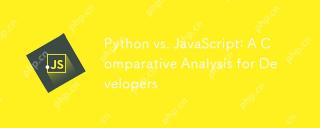
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
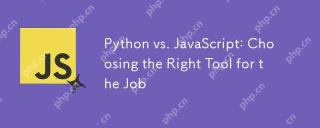
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
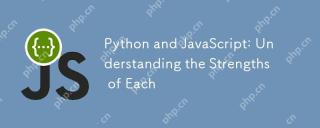
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
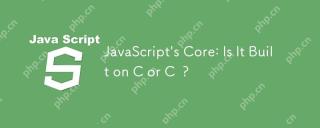
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
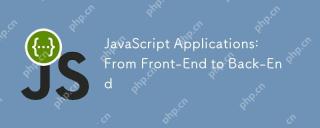
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
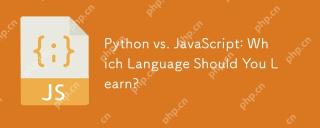
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
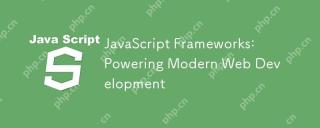
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
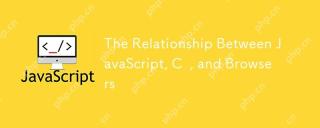
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
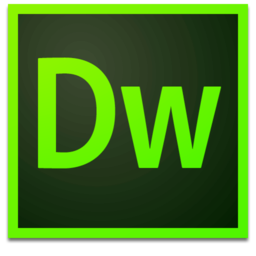
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
