Although JavaScript does not provide the inheritance keyword, we can still come up with some good ways to implement it.
1. Prototype chain inheritance:
var Base = function()
{
This.level = 1;
This.name = "base";
This.toString = function(){
return "base";
};
};
Base.CONSTANT = "constant";
var Sub = function()
{
};
Sub.prototype = new Base();
Sub.prototype.name = "sub";
Advantages: Judging from the instanceof keyword, an instance is both an instance of the parent class and an instance of the subclass. It seems to be the purest inheritance.
Disadvantages: The attributes and methods of the subclass that are different from the parent class must be executed separately after statements such as Sub.prototype = new Base(); and cannot be packaged in the Sub constructor. For example: Sub.prototype.name = "sub"; multiple inheritance cannot be implemented.
2. Construction inheritance:
var Base = function()
{
This.level = 1;
This.name = "base";
This.toString = function(){
return "base";
};
};
Base.CONSTANT = "constant";
var Sub = function()
{
Base.call(this);
This.name = "sub";
};
Advantages: Multiple inheritance can be achieved, and property settings unique to subclasses can be placed inside the constructor.
Disadvantage: Use instanceof to find that the object is not an instance of the parent class.
3. Instance inheritance:
var Base = function()
{
This.level = 1;
This.name = "base";
This.toString = function(){
return "base";
};
};
Base.CONSTANT = "constant";
var Sub = function()
{
var instance = new Base();
Instance.name = "sub";
Return instance;
};
Advantages: It is an object of the parent class, and the same effect can be obtained by using new to construct the object or not using new to construct the object.
Disadvantages: The generated object is essentially an instance of the parent class, not an object of the subclass; multiple inheritance is not supported.
4. Copy inheritance:
{
This.level = 1;
This.name = "base";
This.toString = function(){
return "base";
};
};
Base.CONSTANT = "constant";
var Sub = function()
{
var base = new Base();
for(var i in base)
Sub.prototype[i] = base[i];
Sub.prototype["name"] = "sub";
};
Advantages: Supports multiple inheritance.
Disadvantages: low efficiency; unable to obtain non-enumerable methods of the parent class.
These forms have their own characteristics. As far as the code I provide is concerned, it satisfies the following table:
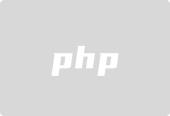
2012-1-10: Supplement, if we don’t need class inheritance, but only object inheritance, for browsers that support ECMAScript 5, we can also use the Object.create method:
var Base = function()
{
This.level = 1;
This.name = "base";
This.toString = function(){
return "base";
};
};
Base.CONSTANT = "constant";
var sub = Object.create(new Base());
sub.name = "sub";