


Compared to C/C, the for loop in ECMAScript cannot create a local context.
for (var k in {a: 1, b: 2}) {
alert(k);
}
alert(k); // Although the loop has ended, the variable k is still in the current scope
At any time, a variable can only be declared by using the var keyword.
The assignment statement above:
a = 10;
This simply creates a new property on the global object (but it is not a variable). "Not a variable" does not mean that it cannot be changed, but that it does not conform to the variable concept in the ECMAScript specification, so it is "not a variable" (the reason why it can become a property of the global object is entirely because there is a global in JavaScript Object, this operation is not to declare a variable but to add an attribute a
to the global object.
Let’s look at a simple example to illustrate the problem
if (!("a" in window)) {
var a = 1;
}
alert(a);
First of all, all global variables are attributes of window. The statement var a = 1; is equivalent to window.a = 1;
You can use the following method to check whether a global variable is declared
"Variable name" in window
Second, all variable declarations are at the top of the scope, look at a similar example:
alert("a" in window);
var a;
At this time, even though the declaration is after the alert, the alert still pops up as true. This is because the JavaScript engine will first scan all variable declarations, and then move these variable declarations to the top. The final code effect is like this:
var a;
alert("a" in window);
Third, you need to understand that the meaning of this question is that the variable declaration is advanced, but the variable assignment is not, because this line of code includes variable declaration and variable assignment.
You can split the statement into code like this:
var a; //Declaration
a = 1; //Initialization assignment
So to sum up, when variable declaration and assignment are used together, the JavaScript engine will automatically divide it into two parts in order to advance the variable declaration. The reason why the assignment step is not advanced is because it may affect the execution of the code. expected result.
The code in the question is equivalent to:
var a;
if (!("a" in window)) {
a = 1;
}
alert(a);
According to the analysis of the above example, when declaring a variable, you must add var before the declared local variable. If you declare a global variable, you do not need to add var (it is best to limit the number of global variables and try to use local variables)
The following describes several features of using var
Using the var statement to declare a variable multiple times is not only legal, but also does not cause any errors.
If a reused statement has an initial value, it acts simply as an assignment statement.
If a declaration is reused without an initial value, it will have no effect on the original variable.
Variables declared without var exist as global variables; variables declared with var are local variables, especially within functions. Moreover, after testing, declaration with var is faster than without var. Set up as many local variables as possible in the function, so that it is safe and fast, and the variable operations are more reasonable. Logic errors will not be caused by random manipulation of global variables in the function.
When declaring an object, it is best to use the object's self-face method, which is much faster than the new method.
The variable name is chosen by yourself. In order to take care of semantics and specifications, the variable name may be slightly longer, but please note that the length of the variable name will also affect the execution speed of the code. Declarations with long variable names do not execute as quickly as short ones.
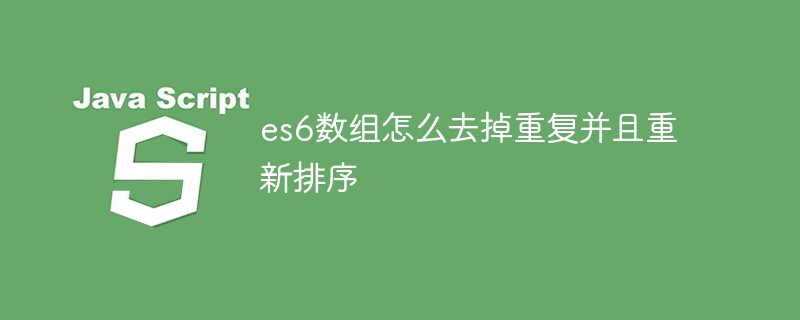
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
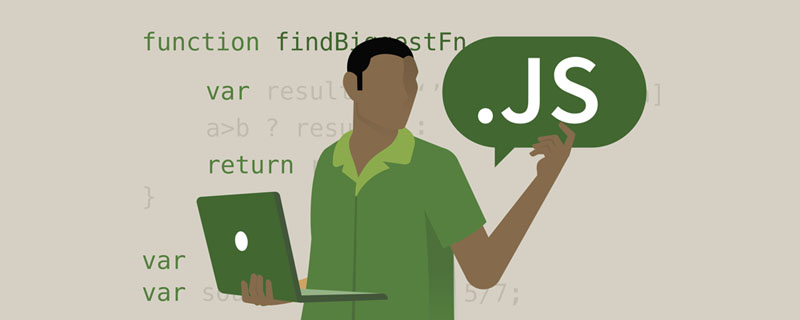
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
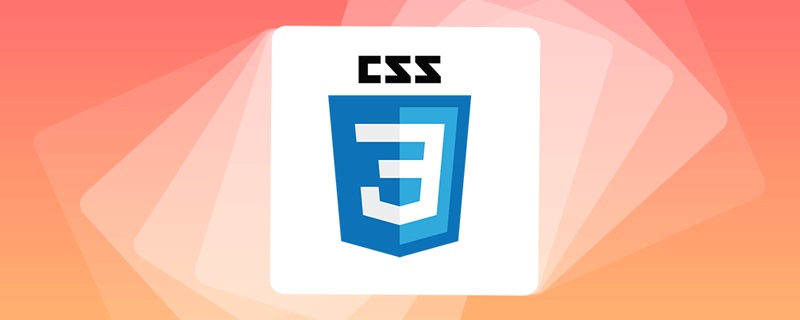
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
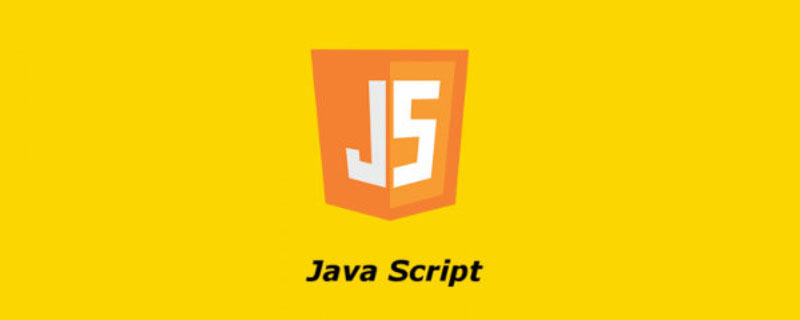
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
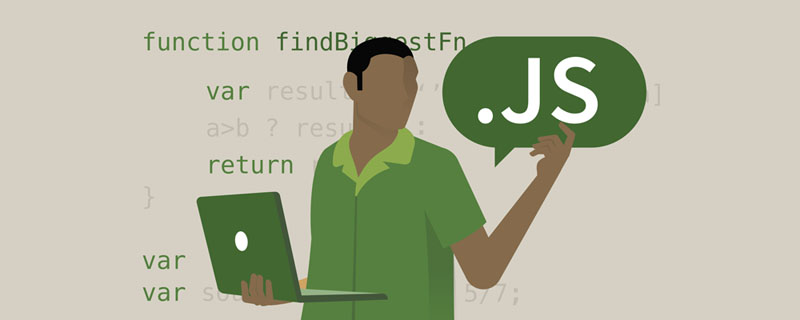
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
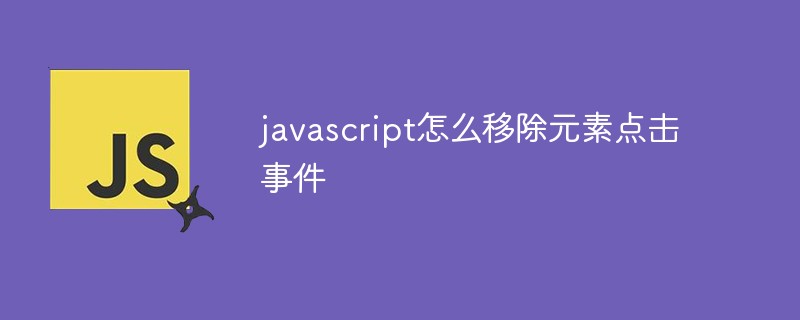
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
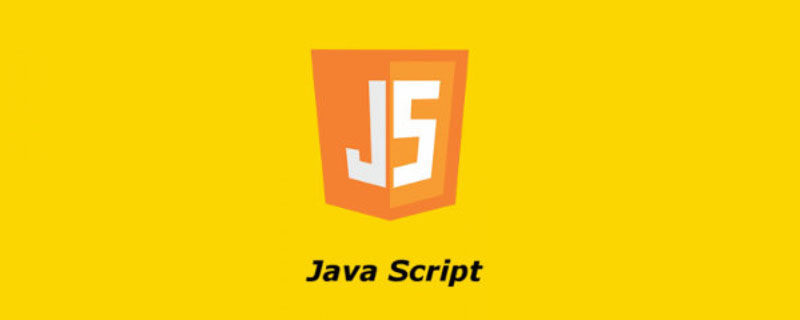
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
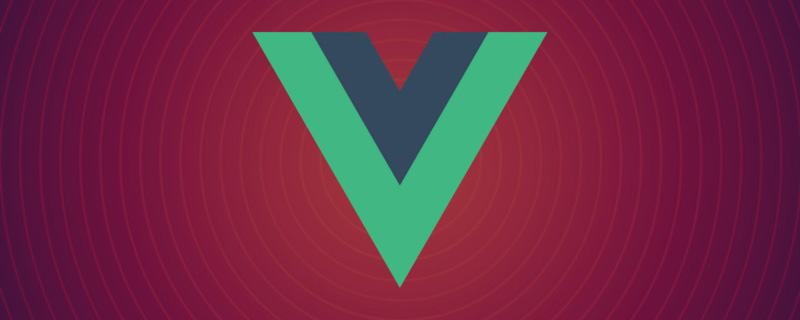
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
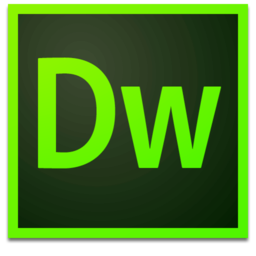
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
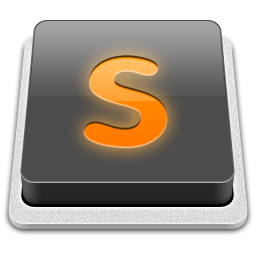
SublimeText3 Mac version
God-level code editing software (SublimeText3)
