3 ways to implement synchronization operations in node.js_node.js
As we all know, asynchronous is a unique feature and advantage, but at the same time, the need for synchronization in the program (for example, the execution order of the control program is: func1 -> func2 ->func3) is also very common. This article records some of my thoughts on this issue.
Function that needs to be executed:
var func1 = function(req,res,callback){
setTimeout(function(){
console.log('in func1');
callback(req,res,1);
},13000);
}
var func2 = function(req,res,callback){
setTimeout(function(){
console.log('in func2');
callback(req,res,2);
},5000);
}
var func3 = function(req,res,callback){
setTimeout(function(){
console.log('in func3');
callback(req,res,3);
},1000);
}
It can be seen that the setTimeout function is used in func1, func2 and func3, and the execution time is 13 seconds, 5 seconds and 1 second respectively. Due to the asynchronous nature of nodejs, if you use the ordinary function calling method:
var req = null;
var res = null;
var callback = function(){};
func1(req,res,callback);
func2(req,res,callback);
func3(req,res,callback);
Output content:
in func3
in func2
in func1
The reason is that because nodejs is asynchronous, func2 will not wait for func1 to finish executing, but will execute it immediately (the same is true for func3). Since func3 has the shortest running time, it ends first, followed by func2 and func1 last. But this is obviously not the result we want. what to do?
Solution 1: callback
//Deep nesting
var req = null;
var res = null;
func1(req,res,function(){
func2(req,res,function(){
func3(req,res,function(){
Process.exit(0);
})
});
});
Although this method can be solved quickly, the problems exposed are also obvious. First, the code maintenance is not good, and second, the deep nesting of the code looks very uncomfortable. This approach is not advisable.
Solution 2: Recursive call
function executeFunc(funcs,count,sum,req,res){
if(count == sum){
Return ;
}
else{
funcs[count](req,req,function(){
Count ;
executeFunc(funcs,count,sum,req,res);
});
}
}
//Synchronous call
var req = null;
var res = null;
var funcs = [func1,func2,func3];
var len = funcs.length;
executeFunc(funcs,0,len,req,res);
First combine multiple functions into an array. You can then use the characteristics of recursive functions to make the program execute in a certain order.
Solution 3: Call the class library
With the development of nodejs, there are more and more corresponding class libraries. Step and async are good ones.
1. The call of Step is relatively refreshing:
Step(
function thefunc1(){
func1(this);
},
function thefunc2(finishFlag){
console.log(finishFlag);
func2(this);
},
function thefunc3(finishFlag){
console.log(finishFlag);
}
);
2.async’s series method, in this case, its calling method:
var req = null;
var res = null;
var callback = function(){};
async.series(
[
Function(callback){
func1(req,res,callback);
},
Function(callback){
func2(req,res,callback);
},
Function(callback){
func3(req,res,callback);
}
]
);
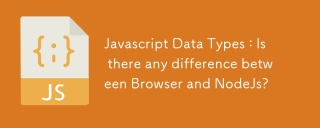
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
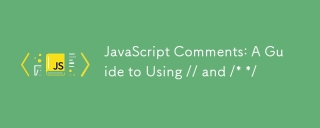
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
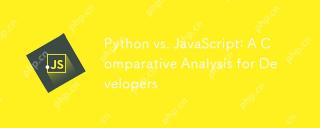
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
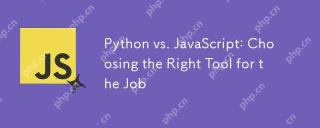
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
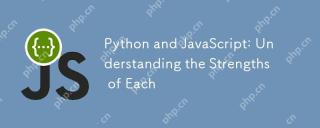
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
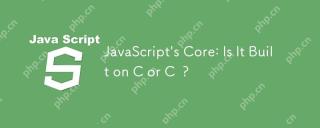
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
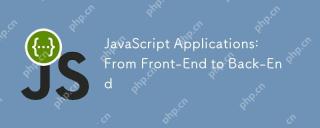
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
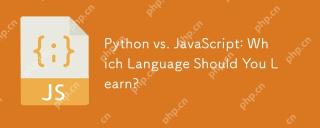
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
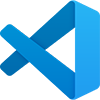
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
