浏览器的js属性
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>浏览器的js属性</title>
</head>
<body>
<!-- 1、预定义属性 id \class、style -->
<button id="1" class="2" style="color: red;">按钮1</button>
<!-- 2、z自定义属性 data-xxx-->
<button id="3" class="4" data-text="自定义">自定义按钮</button>
<!-- 3、预定义事件 on+事件名称 事件属性为JS代码 -->
<button onclick="document.body.style.background='blue'">预定义事件按键</button>
<!-- 写到事件属性的js 仅限对当前目标有效 内联脚本、行内脚本 js作用域 -->
<!-- 如果想点击变色,再次点击还原 -->
<button onclick="set(this)">背景变色</button>
<!-- js 代码可以自动合并 -->
<script>
// 开关
let status = false;
function set (a) {
status = !status;
let bg = status?'yellow' :null;
let text =status ?'还原背景' : '设置背景';
document.body.style.backgroundColor =bg;
a.textContent = text;
}
</script>
<button onclick="set(this)">背景变色</button>
<!-- js 外部引入 ,主语js代码要放到后面,不要放到前面 -->
<script src="../0725/js/zuoye.js"></script>
</body>
</html>
<!-- 外部引入js -->
// 开关
let status = false;
function set (a) {
status = !status;
let bg = status?'yellow' :null;
let text =status ?'还原背景' : '设置背景';
document.body.style.backgroundColor =bg;
a.textContent = text;
}
·
效果
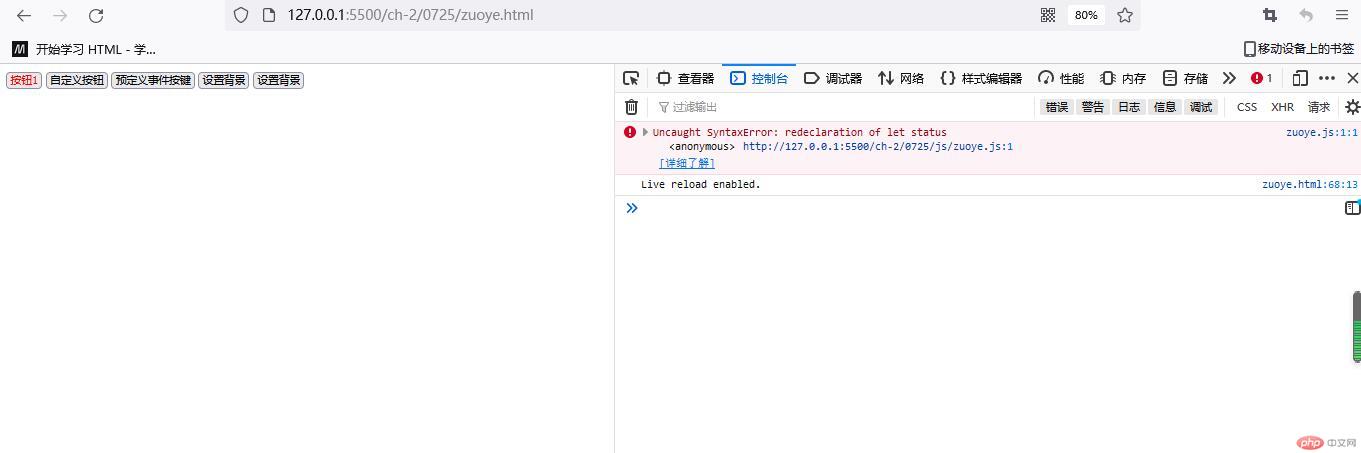
自定义数组
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>类数组</title>
</head>
<body>
<script>
const name = ['小红','小黑','小明'];
console.log(name);
console.log(name[0]);
console.log('------------------');
// 类数组 类似数组
const animals = {
0 : 'dog',
1:'cat',
3:'pig',
};
// 访问方式
// 方法一 0是非法标识符 用【】
console.log(animals[0],animals[1],animals[2]);
// 数组特征
// 1、数组就是一个对象,属性从 0开始递增 有 length属性
// 2、类数组跟 数组类似
</script>
</body>
</html>
效果
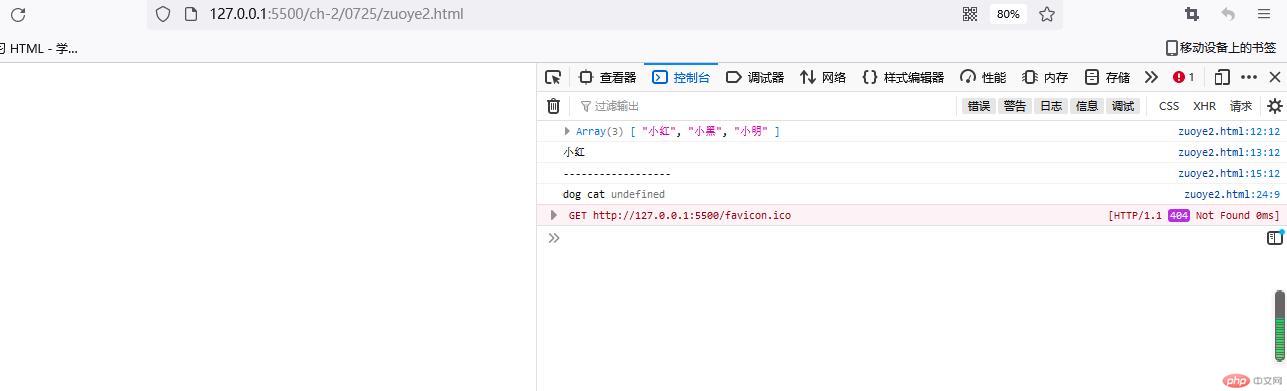
dom元素获取
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>dom元素获取</title>
</head>
<body>
<ul class="list">
<li class="item">item1</li>
<li class="item">item2</li>
<li class="item">item3</li>
<li class="item">item4</li>
<li class="item">item5</li>
<li class="item">item6</li>
</ul>
<script>
// 获取模型/模式两种
// 1、获取一组 querySelectorAll()
// 2、获取一个querySelector()
// 一、一次性获取一组
// 获取ul list 注意 .lits 跟.item之间要有空格
const items = document.querySelectorAll('.list .item');
// douquerySelectorAll()
console.log(items);
// 获取数组接口 注意要keys 复数 节点列表对象
console.log(items.keys());
// 数组采用 for of遍历 获取 键
for (let k of items.keys() )
console.log(k);
// 数组采用 for of遍历 获取 值
for (let v of items.values() )
console.log(v);
// 数组采用 for of遍历 获取 entries 键值对 数组化
for (let kv of items.entries() )
console.log(kv);
console.log('----------------');
// 简化版 forEach(callback) callback 是指回调函数 两个值 第二值 是可选的
// function (当前正在遍历的值,当前值的索引){ } 注意变量跟forEach位置及括弧
items.forEach(function(v,k){
console.log(k,v);
});
console.log('----------------');
items.forEach(function(v){
console.log(v);
// 修改颜色
v.style.backgroundColor = 'red';
});
console.log('----------------');
// 进一步简化 胖箭头
items.forEach(v => console.log(v));
// 修改颜色
items.forEach(v => v.style.backgroundColor = 'blue');
// 二、一次性获取一个 返回的是数组集合的第一个
// document.querySelectorAll()中括弧里面可以使用伪类获取某一个 类似id
// const eles = document.querySelectorAll('.list .item:first-of-type');
// console.log(eles);
const fi = document.querySelectorAll('.list .item:first-of-type');
// 获取第一个
console.log(fi);
// 获取第一个
console.log(fi[0]);
// 给第一个加背景色
fi[0].style.backgroundColor = 'red';
// 优化简版获取
const first = document.querySelector('.list .item');
console.log(first);
// 给第一个 字体换上白色
first.style.color = 'white';
console.log('----------------');
// querySelector/querySelectorAll: 可以在任何元素上调用,不是只用在document
// 获取一组元素 ul 另一 方法
const one = document.querySelector('.list');
console.log(one);
console.log('----------------');
// const ones = list.querySelectorAll('.item');
// const ones = list.querySelectorAll('.item');
// ones.forEach(li => (li.style.backgroundColor = 'violet'));
// 注意词汇 one 已经在上面定义获取为 .list 了
const ones = one.querySelectorAll('.item');
ones.forEach(li => (li.style.backgroundColor = 'violet'));
// 3、快捷方式
console.log(document.querySelector('body'));
console.log(document.body);
</script>
</body>
</html>
效果
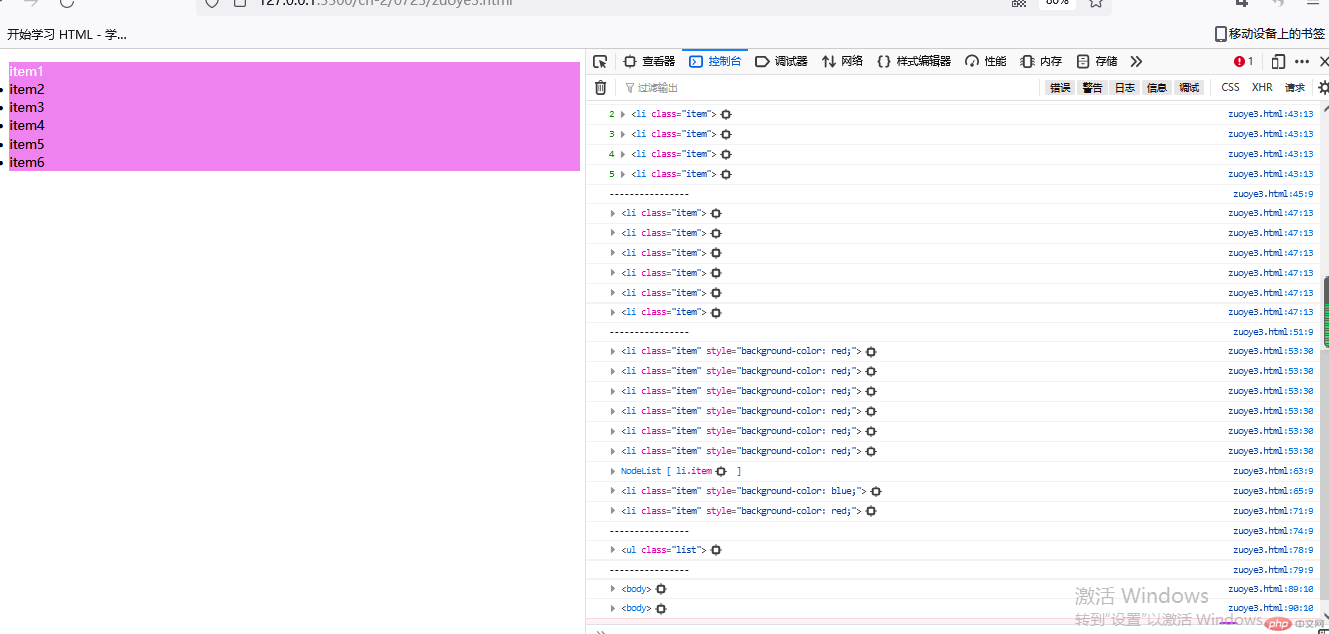
表单元素
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>form表单元素</title>
</head>
<body>
<form aaction="login.php" method="post" id="login">
<!-- fieldset是控件组标签,该标签内容的周围将绘制边框 -->
<fieldset class="login">
<!-- legend 元素为 fieldset 元素定义标题 -->
<legend>用户登录</legend>
<label for="zhanghu">账户:</label>
<input type="zhanghu" name="zhanghu" id="zhanghu" value="18963300001" autofocus>
<br>
<label for="password">密码:</label>
<input type="password" name="password" id="password" value="123456" autofocus>
<br><button>提交</button>
</fieldset>
<script>
// 作业3的方式
const form = document.querySelector('#login');
console.log(form);
console.log(document.querySelector('#zhanghu').value);
console.log('-----------');
// 简洁化 forms. 三种方式
console.log(document.forms[0]);
console.log('-----------');
console.log(document.forms['login']);
console.log('-----------');
console.log(document.forms.login);
console.log('-----------');
// id 相当于name 反过来一样
// 可以跟css 一样 一层一层一点点获取
console.log(document.forms.login .zhanghu.value);
// 前后端分离, 前端发送json到服务器
// json: js对象的序列化,字符串
// 第一步先获取值
let zhanghu = document.forms.login .zhanghu.value;
let password = document.forms.login .password.value;
// 第二 步 开始组装数组
let user = { zhanghu,password};
// 第三步 开始组装js
let json = JSON.stringify(user);
console.log(json);
</script>
</form>
</body>
</html>
效果
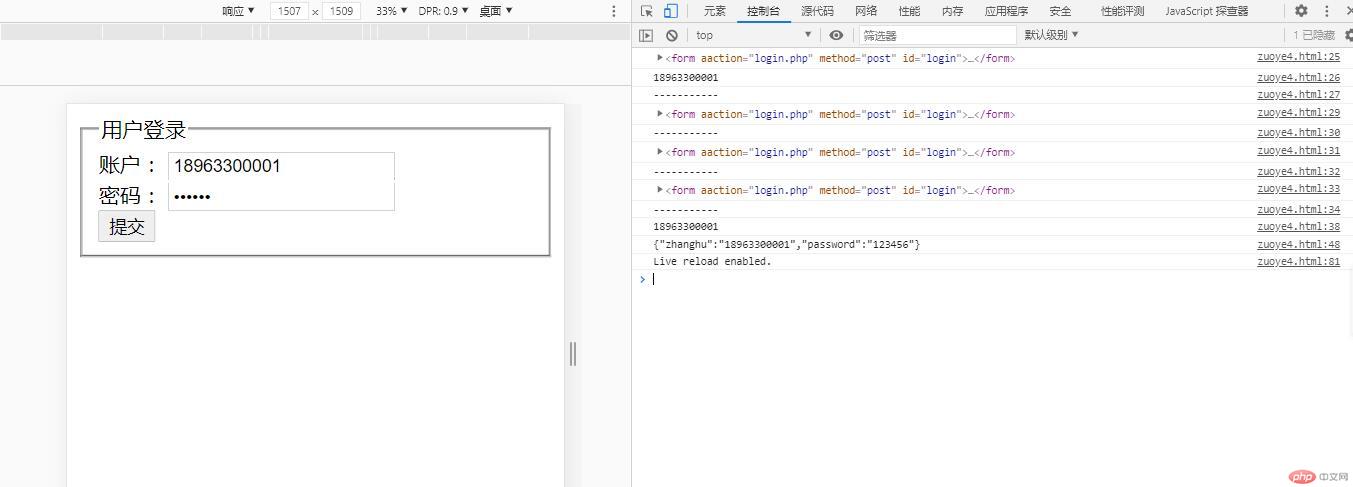
dom树
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>遍历dom树</title>
</head>
<body>
<ul class="list">
<li class="item">item1</li>
<li class="item">item2</li>
<li class="item">item3</li>
<li class="item">item4</li>
<li class="item">item5</li>
<li class="item">item6</li>
</ul>
<script>
// 1、节点类型
// 2、Windows 全局对象
// 2、document 文档对象
// 3、element 元素对象
// 4、textcontent 文本对象
let ul = document.querySelector ('.list');
console.log(ul);
console.log(ul.children);
// ul节点对象,包含回车
console.log(ul.childNodes);
ul.childNodes.forEach(node => {
// 如果节点类型 是1 标识约束节点
if (node.nodeType ==1){
console.log(node);
}
});
// 只返回元素类型的节点,我们需要关注。类数组形式
console.log(ul.children);
// 给所有元素变颜色
// 第一步,类数组变为真数组 不建议
console.log(Array.from(ul.children));
// 简化版 类数组变为真数组 ...rest
console.log(...ul.children);
// 手动放到数组内 并使用foreach遍历
[...ul.children].forEach(li =>(li.style.color = 'blue'));
// 遍历
// 获取第一个
console.log([...ul.children][0]);
// 给第一个加背景色
[...ul.children][0].style.backgroundColor = 'red';
// 优雅简答
// 获取第一个
ul.firstElementChild.style.backgroundColor = 'red';
// 获取下一个
ul.firstElementChild.nextElementSibling.style.backgroundColor = 'red';
// 获取最后一个
[...ul.children][ul.children.length - 1].style.backgroundColor = 'red';
// 获取最后一个再次简化
ul.lastElementChild.style.backgroundColor = 'blue';
// 获取最后一个的前一个
ul.lastElementChild.previousElementSibling.style.backgroundColor = 'blue';
// 获取父节点 父节点一定是元素 或文档节点
ul.lastElementChild.parentElement.style.border = '5px solid red'
</script>
</body>
</html>
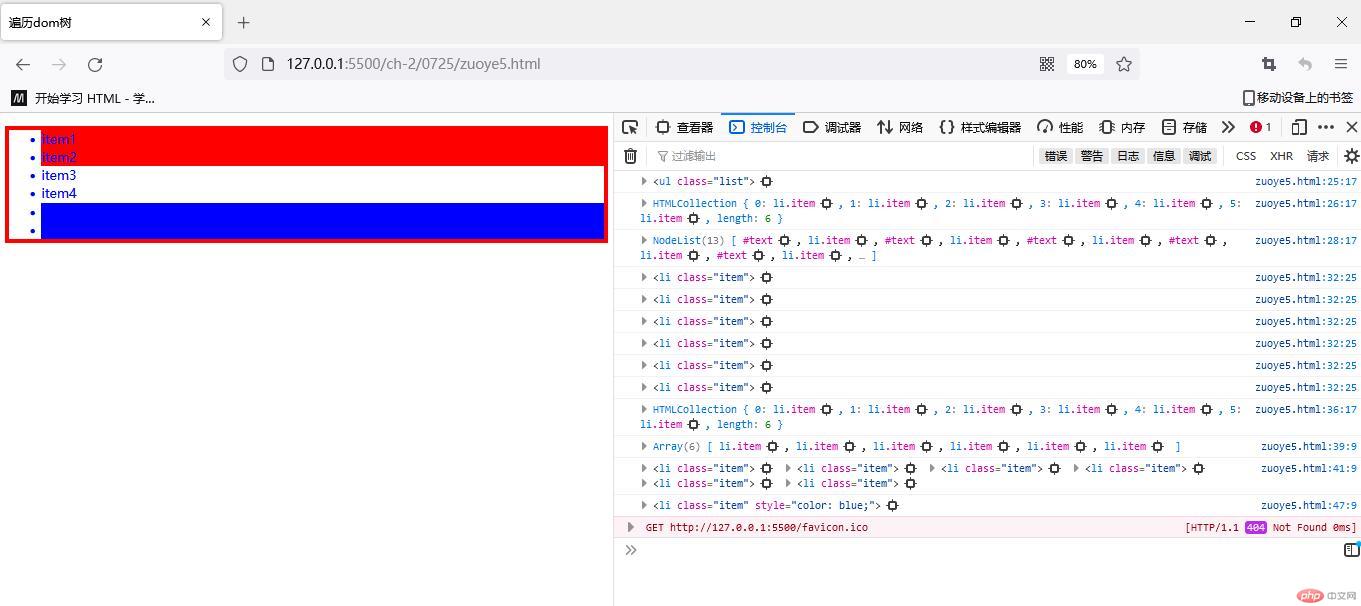