1、对象字面量 2、访问接口3、接口属性化
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>访问器属性</title>
</head>
<body>
<script>
// 1、对象字面量
// 2、访问接口
// 3、接口属性化 -
// 对象声明的两种方式
// 1、一次性添加
let item = {
date : {
name :'电脑',
price :5000,
}
}
console.log(item);
console.log(item.date);
console.log(item.date.name);
console.log(item.date.price);
console.log('============');
// 为属性 data price 设置访问接口
// 接口就是 方法 函数
// 数据 只有两种访问方式:读取、写入
let item1 = {
date1 : {
name1 :'电脑',
price1 :5000,
},
// 读取操作
getPrice () {
return item1.date1.price1;
},
// 写操作,一定要传参
setPrice(value){
this.date1.price1 = value;
},
}
// 注意方法后面要跟括弧、
// 给大家拓展个小技巧,括号我是这么记得:
// 首先是中括号[]:这玩意儿绝大多数情况下都是用来包裹数组的。
// 然后是大括号{}:这玩意儿绝大多数是用来包裹代码段(块)和对象
// 的,比如自己写的函数的代码逻辑部分就得在大括号里。
// 最后是小括号():这玩意儿绝大多数是用来跟函数打交道的。比如说调用
// 函数后面得跟它,自己写的函数,函数的参数得传在小
// 括号里。还有一部分情况是需要让编程语言将一段代码
// 看做一个整体,需要用小括号包裹起来。
console.log(item1.getPrice());
console.log('------------');
item1.date1.price1 = 30;
console.log(item1.getPrice());
console.log('------------');
console.log(item1.date1.price1);
console.log('------------');
// 用 price1的访问接口
item1.setPrice(90);
console.log(item1.getPrice());
console.log('============');
// 2、逐个追加
// 先创建一个空对象(理论上的)
let item2 = { };
item2.name2 = '钢琴';
item2.price2 =9999;
item2.date ={};
item2.date.width = 100;
item2.date.color = 200;
console.log(item2);
console.log(item2.name2);
console.log(item2.date);
console.log('-------------------');
// 读取操作
item2.getPrice = function (){
return item2.date.width;
};
// 写操作,一定要传参
item2.setPrice = function(value){
this.date.width = value ;
};
console.log(item2.getPrice());
console.log('-------------------');
item2.setPrice(600);
console.log(item2.getPrice());
console.log('============');
// 采用接口数据验证
let item3 = { };
item3.name3 = '钢琴';
item3.price3 =9999;
item3.date ={};
item3.date.width = 550;
item3.date.color = 200;
console.log(item3);
console.log(item3.name3);
console.log(item3.date);
console.log('-------------------');
// 读取操作
item3.getPrice = function (){
return item3.date.width;
};
// 写操作,一定要传参
item3.setPrice = function(value){
if(value < 4000) return false;
this.date.width = value ;
};
console.log(item3.getPrice());
console.log('-------------------');
item3.setPrice(5500);
console.log(item3.getPrice());
console.log('-------------------');
// 价格小于4000失效
item3.setPrice(2000);
console.log(item3.getPrice());
// 价格大于4000生效
item3.setPrice(5300);
console.log(item3.getPrice());
// 简化版 模板字面量
// 注意反引号、
console.log(`${item3.date.width} : ${item3.date.color} `);
console.log('--********--');
// 再次简化 : 接口属性化
let item4 = {
date4 :{
name4:'苹果',
price4:30,
},
// price 属性的访问接口 属性为 访问器属性。看着
get price4() {
return this.date4.price4;
},
set price4(value) {
this.date4.price4 = value;
},
// 经过以上操作,访问接口全部属性化了
// 这是访问器属性,实际上是方法,只不过伪装成了属性。套用一个属性的马甲
};
// 访问器属性:属性二字,表明了该方法的使用方式
console.log(item4.price4);
console.log(`${item4.price4} `);
</script>
</body>
</html>
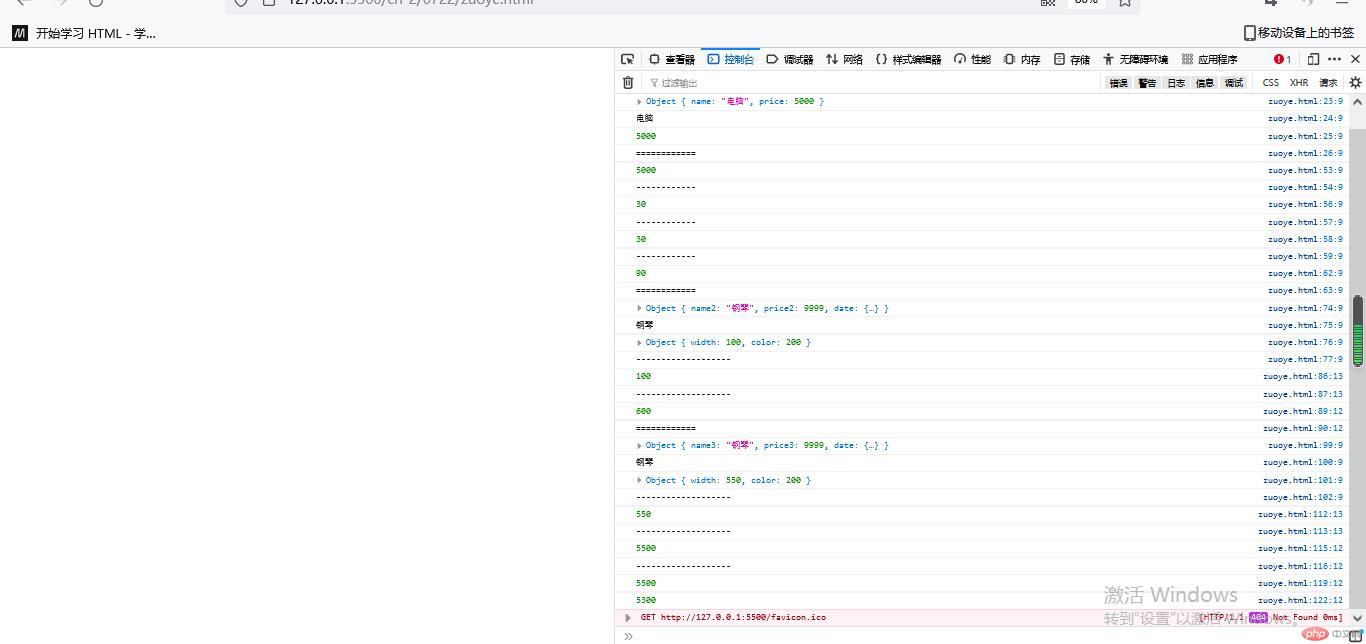
构造函数、对象访问
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>构造函数、面向对象特征</title>
</head>
<body>
<script>
// 函数:创建一个对象
let User1 =function (name ,sex) {
// 步骤 三步
// 1步骤一:创建空对象 保存结果
let obj = {};
// 2、为这个新对象,添加属性和方法
obj.name = name;
obj.sex = sex ;
// 3、返回这个新对象
return obj ;
};
let user1 = User1('小明','男');
console.log(user1);
let user2= User1('小红','女');
console.log(user2);
// 构造函数:专用来创建对象的函数 以下
// 可以用来简化,直接this 引用 这个新对象
let User2 =function (name ,sex) {
this.name = name;
this.sex = sex ;
};
let user3 = User2('小黑','男')
console.log(user3);
// 此时 this的 指向有问题 没有指向对象,而是指向全局了 Window this指向有问题需要修正
// 以上
// 构造函数:专用来创建对象的函数 以下
// 可以用来简化,直接this 引用 这个新对象
let User3 =function (name ,sex) {
this.name = name;
this.sex = sex ;
};
// new 可以修正 构造函数内部 this的指向。指向新的对象,而非Window
// 因此构造函数必须 是用new 调用
let user4 = new User2('小黑','男')
console.log(user4);
console.log('============');
// 此时 this的 没有有问题 修正后
// 以上
// 构造函数: 创建对象(实例) ,通常对象和实例是同义
// 构造函数 必须使用new调用
let User5 = function (name , sex ){
// 属性
this.name =name;
this.sex = sex;
// 方法
this.getInfo =function() {
// 模板字面量,注意反引号,注意大括号
return `${this.name}:${this.sex}`;
};
};
let user5 = new User5 ('小白','女');
let user6= new User5 ('小小','女');
console.log(user5);
console.log('----------');
console.log(user5,user6);
// 此时两个对象user5和 user6属性不同,是正确的,但是方法一样,出现代码冗余,需要简化
// 多余的方法,实际上应该是被所有实例所共享,只需要保留一个方法即可
// 不需要为每个对象保留一遍方法的代码
// 其中prototype
console.log('----------');
// 查看对象的构造器,查本溯源
console.log(user5.constructor);
// 查看构造函数
console.log(User5);
// 对象的构造器等于 构造函数、、
console.log(user5.constructor == User5);
console.log('----------');
// 自动打开
console.dir(User5);
// 所有函数都有一个prototype
// 这个原型属性对普通函数,没有用、、
// 但是对于构造函数,有用、
// 构造函数原型属性的成员,,可以被该该构造函数所有实例共享
console.log('----------');
// 自己指向了自己
console.dir(User5.constructor);
console.log('----------');
console.log(User5.constructor);
console.log('-===========--');
let User6 = function (name , sex ){
// 属性
// 自有属性
this.name =name;
this.sex = sex;
// 方法 放在这儿会被每个对象生成一个副本,因此放到公共位置
// this.getInfo =function() {
// // 模板字面量,注意反引号,注意大括号
// return `${this.name}:${this.sex}`;
// };
};
// 将对象的共享成员,挂载到构造器的原型属性prototype上
// 共享属性
User6.prototype.getInfo = function (){
// 模板字面量
return `${this.name}:${this.sex}`;
};
let user7 = new User6 ('小朱','女');
let user8= new User6 ('小刘','女');
// 不会展示原型属性
console.log(user7,user8);
// 查看原型属性
console.log(User6.prototype);
console.log('-===========--');
// 共享成员:加到原型属性
// 1、自有成员 直接在方法 this.xxx
// 2、共享成员,构造器的原型属性 fun.prototype
// 3、私有成员 let
// 4、静态成员 static 当前构造函数使用static
// 5、继承
let User7 = function (name , sex ){
// 私有成员
let email = '1123@qq.com';
// 属性
// 自有属性
this.name =name;
this.sex = sex;
};
// 静态成员: 只能用构造函数 自身防伪,不能用实例访问
// 只属于 构造函数。不属于 实例对象, 只能有类访问
// 函数也是对象,是对象就可以为他添加属性
User7.nation = '中国';
console.log(User7.nation);
console.log('-----------');
let y = new User7('a','b');
// 访问不到 nation
console.log(y.nation);
console.log('-===========--');
// 继承
// jS没有类的概念 基于原型来实现继承
// 父级为构造函数
let Parent = function() {};
// 方法公共的
Parent.prototype.sum = function(){
return this.a +this.b;
};
// 子类构造器,构造函数、、
let Child = function (a,b) {
this.a =a;
this.b =b ;
}
// 子类继承父类的原型方法
Child.prototype = Parent.prototype;
let child = new Child (1,20);
console.log(child.sum());
</script>
</body>
</html>
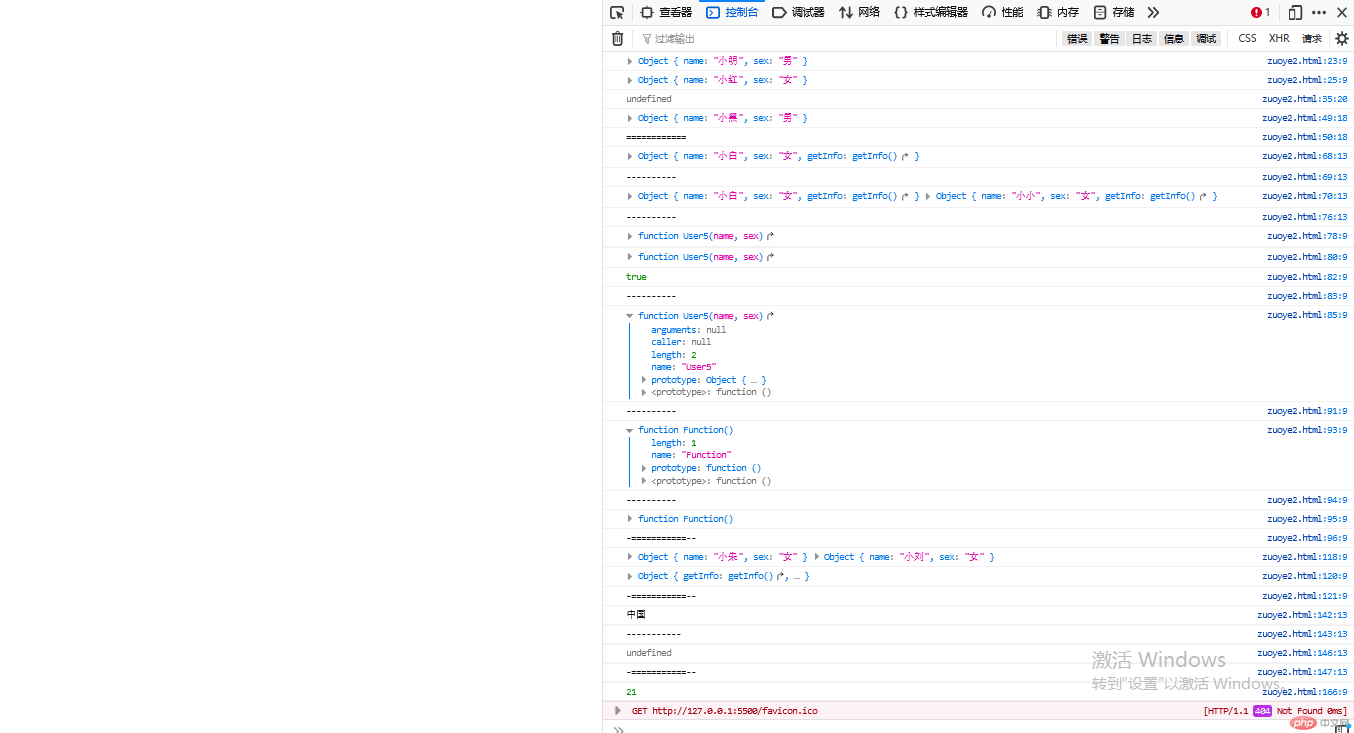
class类简化构造器
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>class类简化构造函数创建对象的过程</title>
</head>
<body>
<script>
// 类?
// 其实js没有类 ,js中的类就是函数
// 声明类 ,空类
class Class1 {};
console.dir(Class1);
console.log('----------');
console.dir(function(){});
console.log('----------');
console.log(typeof Class1);
console.log('----------');
// es6 class
// class 声明类
class Demo1 {
// 构造方法,实例初始化
// 注意构造方法后面 不要有分号
constructor(name ,sex) {
// 自有属性
this.name= name;
this.sex = sex;
// 注意构造方法后面 不要有分号
}
// 共享、原型成员 声明在原型里面
// 注意构造方法后面 不要有分号
getInfo() {
// 模板字面量
return `${this.name}:${this.sex} `;
}
// 静态成员
static status = 'enable';
};
const obj = new Demo1 (`小黑`,'男');
console.log(obj.getInfo());
console.log('----------');
// 访问不到 静态成员要以构造函数访问
console.log(obj.status);
console.log('----------');
// 访问到了
console.log(Demo1.status);
console.log('----------');
// 继承 extends Demo2 也是类
class Demo2 extends Demo1 {
// 子类 构造器 写的方法一
// 注意构造方法后面 不要有分号
// constructor(name ,sex) {
// 自有属性
// this.name= name;
// this.sex = sex;
// 注意构造方法后面 不要有分号
// }
// 子类 构造器 写的方法二
// super()会自动 调用父类的构造器初始化该实例
constructor(name ,sex,email) {
super(name,sex);
// 子类自有属性 子类属性初始化
this.email =email;
}
// 子类对父类方法初始化和扩展
getInfo() {
// 模板字面量 写法一
// 反引号中不能有中文
return `${this.name}:${this.sex},${this.email} `;
// 简写
return `${super.getInfo()}+${this.email} `;
}
}
const obj2 = new Demo2('小曹','男','244@qq.com');
console.log(obj2.getInfo());
console.log('----------');
// 在类中可以使用访问器属性
class Demo3 {
age = 30;
get age(){
return this.age;
}
set age(value){
this.age =value;
}
}
// 访问器属性实现
const obj4 = new Demo3 ();
console.log(obj4.age);
console.log('----------');
obj4.age = 80;
console.log(obj4.age);
</script>
</body>
</html>
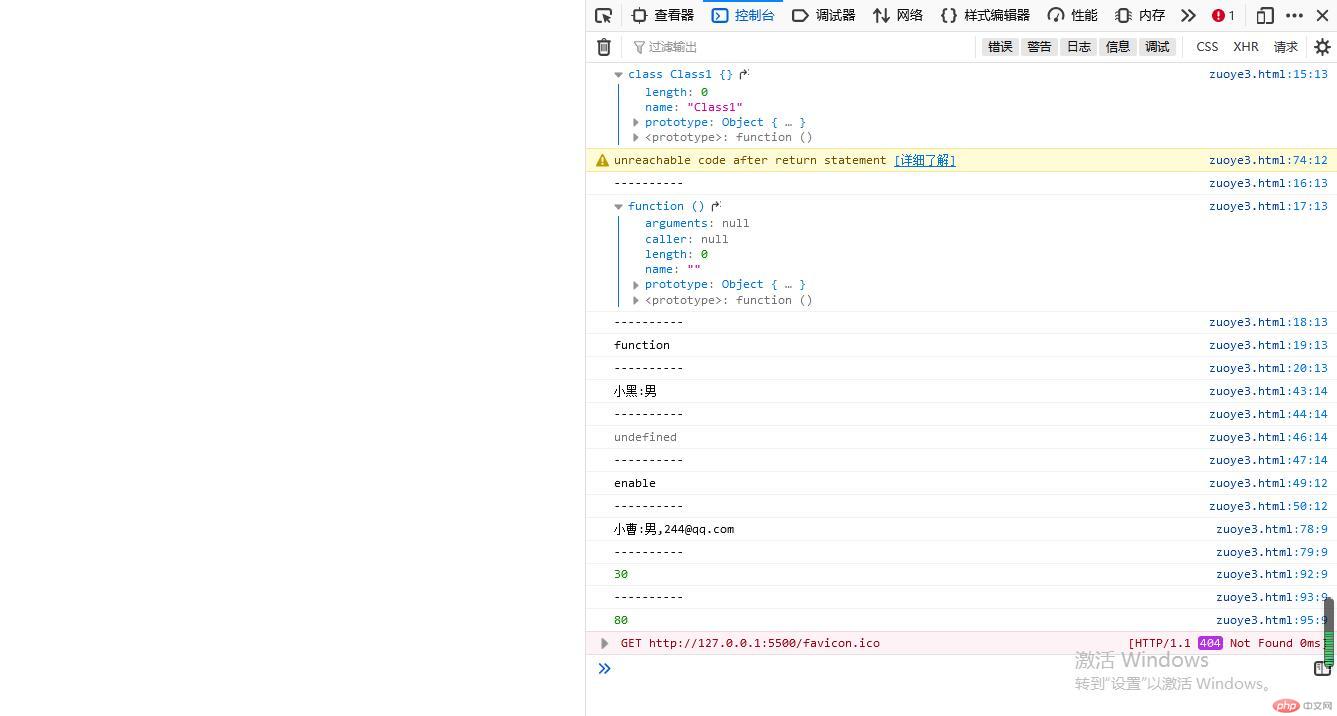
解构赋值
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>解构赋值</title>
</head>
<body>
<script>
const user = ['小红','女'];
let username = user[0];
let username1 = user[1]
console.log(username ,username1);
console.log('------------');
// 1、数字解构
// 更新
[username ,username1] = ['小芳','女'];
console.log(username ,username1);
// 初始化
let [username2 ,username3] = ['小购','女'];
console.log(username2 ,username3);
console.log('------------');
// 参数不足:默认值上
// 模板中有三个变量,但是值只有两个 不足,只展示两个
[username ,username1,age] = ['小解','女'];
console.log(username ,username1);
// 参数过多
[a,b,c,...arr] = [1,2,3,4,5,6,7];
console.log(a,b,c);
console.log(...arr);
// 二次交换
let x =10;
let y =20;
console.log([x,y]);
console.log('------------');
// let z = x;
// let x = y ;
// let y = z;
[y,x] = [x,y];
console.log([x,y]);
console.log('------------');
// 对象解构
// 目标是吧对象中的属性值保存到对应的,与属性同名的变量中
let {id , lesson ,price} = {id :1, lesson :'语文',price:90};
console.log(id, lesson,price );
// 更新 必须转为表达式 小括号 把整个表达式封装起来
({id , lesson ,price} = {id :2, lesson :'数学',price:60});
console.log(id, lesson,price );
console.log('------------');
// 如果左边模板中的变量存在命名冲突,需要起一个别名
let {id:item , num ,score} = {id :3, num :'英语',score:50};
console.log(item,num,score);
console.log('------------');
// 应用场景
function getUser(user123){
// user123 是一个对象
console.log(user123.id11, user123.name11, user123.email11);
}
// 方法一
let user123 = { id11:55, name11:'小好',email11:'1234@qq.com'}
// 方法一 调用
getUser(user123);
console.log('------------');
let user456 = {id1234:11, name1234:11,email1234:11};
function getUser2({id1234, name1234,email1234}){
// user456 是一个对象
console.log(id1234, name1234,email1234);
}
// 方法一 调用
getUser2(user456);
</script>
</body>
</html>
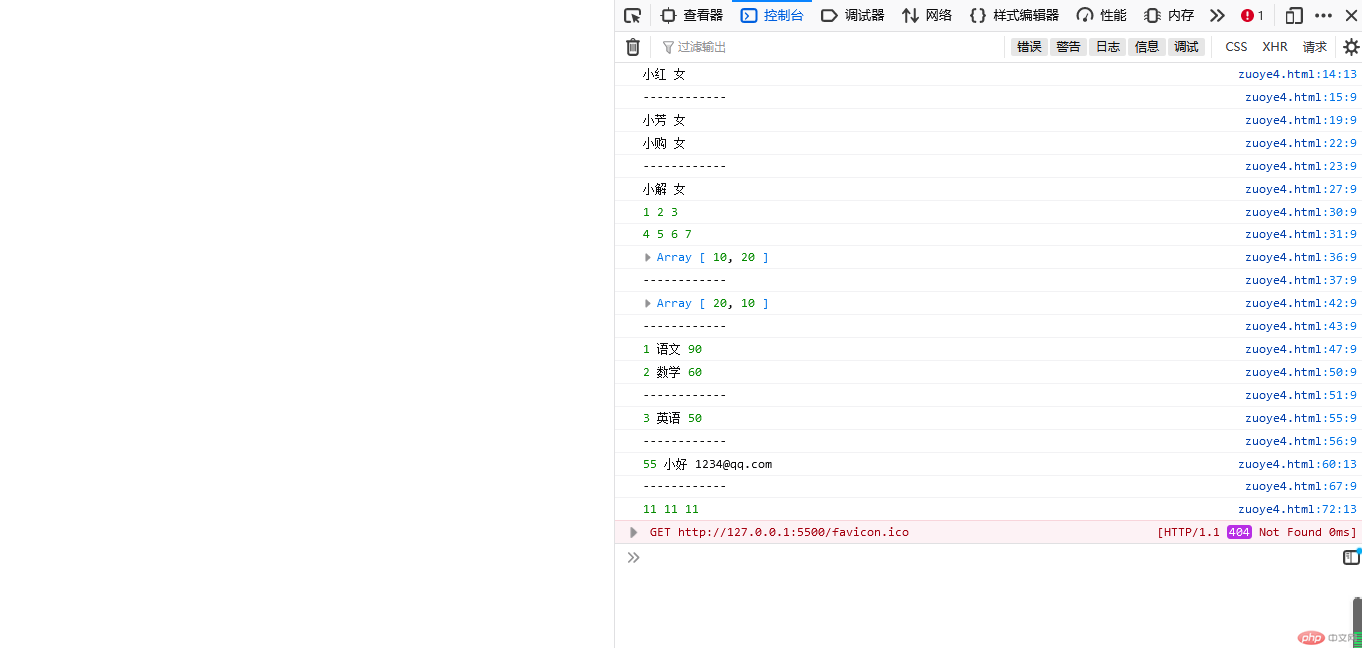