双色球
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>双色球</title>
<style>
.winning-box{
height: 80px;
display: none;
grid-template-columns: repeat(7,50px);
gap:20px;
margin-top: 20px;
place-content: center;
place-items: center;
}
.winning-box .redNum{
width: 50px;
height: 50px;
border-radius: 50%;
display: grid;
place-items: center;
background-color: #DC1E0F;
padding: auto;
box-shadow: 3px 3px 2px gray;
}
.winning-box .blueNum{
width: 50px;
height: 50px;
border-radius: 50%;
display: grid;
place-items: center;
background-color: #2C8EFF;
padding: auto;
box-shadow: 3px 3px 2px gray;
}
.winning-box .redNum:hover,
.winning-box .blueNum:hover{
cursor: pointer;
width: 60px;
height: 60px;
transition: 0.5s;
}
.form{
display: flex;
gap: 20px;
place-content: center;
}
.form>li{
list-style: none;
}
span{
display: flex;
margin-top: 20px;
place-content: center ;
}
</style>
</head>
<body>
<div>
<div class="winning-box">
</div>
</div>
<span class="s">请输入您要选的号码</span>
<ul class="form">
<li><input type="number" name="" id="" class="number" max="33" min="0"></li>
<li><input type="number" name="" id="" class="number" max="33" min="0"></li>
<li><input type="number" name="" id="" class="number" max="33" min="0"></li>
<li><input type="number" name="" id="" class="number" max="33" min="0"></li>
<li><input type="number" name="" id="" class="number" max="33" min="0"></li>
<li><input type="number" name="" id="" class="number" max="33" min="0"></li>
<li><input type="number" name="" id="" class="number" max="16" min="0"></li>
<button onclick="getDate()">Submit</button>
</ul>
<script>
// 红球数组
let red = [];
// 蓝球数组
let blue = [];
// 中奖数组
let Award_Winning = [];
// 生成1-33的红球数组
for(let a=1;a<=33;a++){
red.push(a);
}
// console.log(red);
// 生成1-16的蓝球数组
for(let a = 1;a<=16;a++){
blue.push(a);
}
// console.log(blue);
// 随机取红球
for (let a = 0;a < 6;a++){
let index = Math.floor(Math.random()*red.length);
Award_Winning.push(red[index]);
red.splice(index,1);
}
Award_Winning.sort((a,b)=> a-b);
let index = Math.floor(Math.random()*blue.length);
Award_Winning.push(blue[index]);
console.log(Award_Winning);
const winning = document.querySelector('.winning-box');
Award_Winning.forEach((item,index)=>{
if(index<=5){
let div = document.createElement('div');
div.className='redNum';
div.textContent=Award_Winning[index];
winning.append(div);
}else {
let div = document.createElement('div');
div.className='blueNum';
div.textContent=Award_Winning[index];
winning.append(div);
}
});
const ul =document.querySelector('.form');
const box = document.querySelector('.winning-box');
function getDate(){
// 用户数组
let arr = [];
const li =document.querySelectorAll('.number');
li.forEach(a=>arr.push(parseInt(a.value)));
// console.log(arr)
// 对比中将数组输出是否中奖
let flag=0;
for (let i = 0;i<7;i++){
for(let j=0;j<7;j++){
if(arr[i]===Award_Winning[j]){
flag++;
break;
// console.log(flag);
}
}
}
if(flag===7){
let span=document.createElement('span');
span.className='conguratulate';
span.textContent='恭喜中奖';
ul.insertAdjacentElement('afterend',span);
box.style.display='grid';
}
else if(flag===0){
let span=document.createElement('span');
span.className='conguratulate';
span.textContent=`很遗憾没有中奖!
本期中奖号码如上`;
ul.insertAdjacentElement('afterend',span);
box.style.display='grid';
}
}
</script>
</body>
</html>
效果:
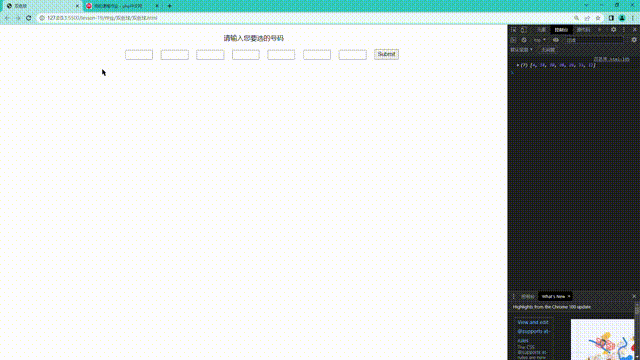
购物车
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>购物车</title>
<style>
.box {
width: 22em;
height: 2em;
}
.list>li {
height: 1.6em;
background-color: #efefef;
display: grid;
grid-template-columns: repeat(5, 3em);
gap: 1em;
place-items: center right;
border-bottom: 1px solid #ccc;
}
.list>li:first-of-type {
background-color: lightseagreen;
color: white;
}
.list>li:hover:not(:first-of-type) {
cursor: pointer;
background-color: lightcyan;
}
.list>li input[type='number'] {
width: 3em;
border: none;
outline: none;
text-align: center;
font-size: 1em;
background-color: transparent;
}
.list>li:last-of-type span.total-num,
.list>li:last-of-type span.total-amount {
grid-column: span 2;
place-self: center right;
color: lightseagreen;
}
.account {
float: right;
background-color: lightseagreen;
color: white;
border: none;
outline: none;
width: 4.5em;
height: 1.8em;
}
.account:hover {
background-color: coral;
cursor: pointer;
}
</style>
</head>
<body>
<div class="box">
<div class="selectAll">
<!-- checkAll(): 当全选按钮的状态发生变化化,触发该事件 change -->
<input type="checkbox" class="check-all" name="check-all" onchange="checkAll()" checked />
<label for="check-all">全选</label>
</div>
<ul class="list">
<li><span>选择</span><span>品名</span><span>数量</span><span>单价</span><span>金额</span></li>
<li>
<input type="checkbox" onchange="checkItems()" checked />
<span class="content">手机</span>
<input type="number" value="1" min="1" class="num" />
<span class="price">100</span>
<span class="amount">0</span>
</li>
<li>
<input type="checkbox" onchange="checkItems()" checked />
<span class="content">电脑</span>
<input type="number" value="2" min="1" class="num" />
<span class="price">200</span><span class="amount">0</span>
</li>
<li>
<input type="checkbox" onchange="checkItems()" checked />
<span class="content">相机</span>
<input type="number" value="3" min="1" class="num" />
<span class="price">300</span>
<span class="amount">0</span>
</li>
<li>
<span>总计:</span>
<span class="total-num">0</span>
<span class="total-amount">0</span>
</li>
</ul>
<button class="account">结算</button>
</div>
<script>
function checkAll() {
//全选按钮状态
let status = event.target.checked;
//子商品状态
document
.querySelectorAll(".list li input[type='checkbox']")
.forEach((item) => (item.checked = status));
autoCalculate();
}
function checkItems() {
//全部商品
let items = document.querySelectorAll(
".list li input[type='checkbox']"
);
//判断状态
let status = [...items].every((item) => item.checked === true);
document.querySelector(".check-all").checked = status;
autoCalculate();
}
const nums = document.querySelectorAll(".num");
//商品是否被选中
function goodStatus(numArr) {
let items = document.querySelectorAll(
".list li input[type='checkbox']"
);
return numArr.map((num, index) => {
if (items[index].checked === false) {
return (num = 0);
} else {
return num;
}
});
}
//计算总数量
function getTotalNum(numArr) {
numArr = goodStatus(numArr);
return numArr.reduce((acc, cur) => acc + cur);
}
//计算每个商品金额
function getAmount(numArr, priceArr) {
return numArr.map((num, index) => num * priceArr[index]);
}
//总金额
function getTotalAmount(amountArr) {
amountArr = goodStatus(amountArr);
return amountArr.reduce((acc, cur) => acc + cur);
}
//自动计算
function autoCalculate() {
//数量数组
const numArr = [...nums].map((num) => parseInt(num.value));
//单价数组
const prices = document.querySelectorAll(".price");
const pricesArr = [...prices].map((price) =>
parseInt(price.textContent)
);
//金额数组
const amountArr = getAmount(numArr, pricesArr);
document.querySelector(".total-num").textContent = getTotalNum(numArr);
document.querySelector(".total-amount").textContent =
getTotalAmount(amountArr);
//为每个商品填充金额
document
.querySelectorAll(".amount")
.forEach((amount, index) => (amount.textContent = amountArr[index]));
}
//自动加载
window.onload = autoCalculate();
//数量变化自动计算
[...nums].forEach((num) => (num.onchange = autoCalculate));
</script>
</body>
</html>
效果
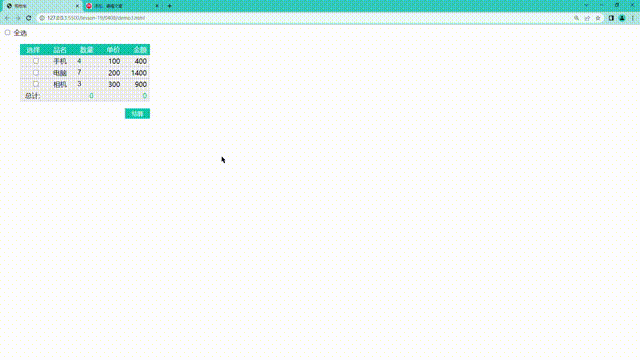