演示效果
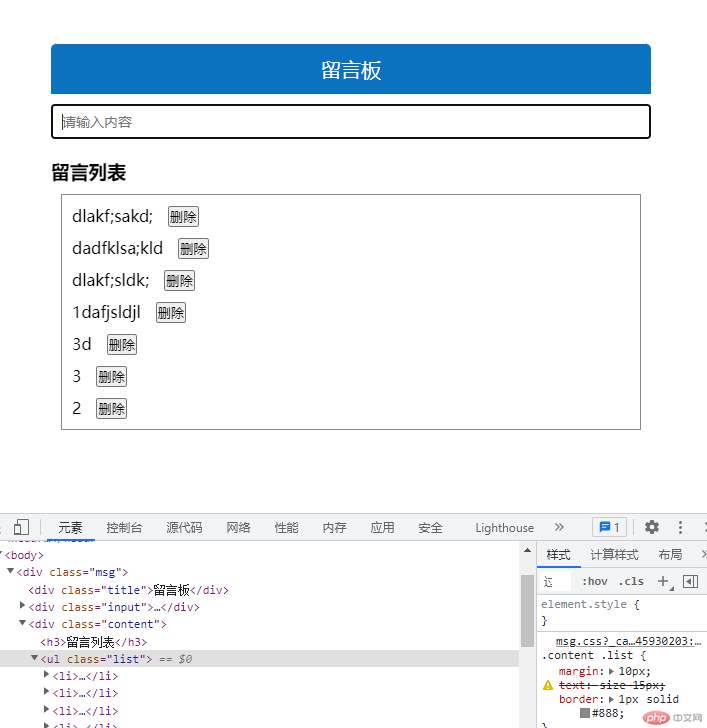
HTML和JS代码
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>留言板</title>
<link rel="stylesheet" href="msg.css" />
</head>
<body>
<div class="msg">
<div class="title">留言板</div>
<div class="input">
<input type="text" onkeydown="addMsg(this)" placeholder="请输入内容" autofocus />
</div>
<div class="content">
<h3>留言列表</h3>
<ul class="list"></ul>
</div>
</div>
<script>
function addMsg(ele) {
// console.log(event);
// console.log(event.key);
if (event.key === "Enter") {
// 1.获取显示留言的容器
const ul = document.querySelector(".list");
// 2.判断用户留言是否为空
if (ele.value.trim().length === 0) {
alert("留言不能为空");
ele.focus();
return false;
}
// 3.添加一条新留言
const li = document.createElement("li");
// console.log(ele.value);
// li.textContent = ele.value;
li.innerHTML = ele.value + "<button onclick = 'del(this.parentNode)'>删除</button>";
// ul.append(li);
// if (ul.firstElementChild !== null) {
// ul.firstElementChild.before(li);
// } else {
// ul.append(li);
// }
// 简洁写法
ul.insertAdjacentElement("afterBegin", li);
// 4.清空上一条留言
ele.value = null;
// 5.焦点重置
ele.focus();
}
}
// 删除
function del(ele) {
console.log(ele);
// 删除1
// ele.remove();
// 删除2
// ele.outerHTML = null;
// 判断
// if (confirm("是否删除?")) {
// ele.remove();
// } else {
// return false;
// }
// 简化判断
return confirm("是否删除?") ? ele.remove() : false;
}
</script>
</body>
</html>
CSS代码
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
a {
text-decoration: none;
color: #555;
}
li {
list-style: none;
}
.msg {
width: 600px;
margin: 50px auto;
background-color: white;
border-radius: 5px;
}
.title {
width: 100%;
height: 50px;
line-height: 50px;
padding: 0 40px;
background-color: #0b72c0;
color: #fff;
font-size: 20px;
text-align: center;
border-top-left-radius: 5px;
border-top-right-radius: 5px;
}
.input input[type="text"] {
width: 100%;
height: 35px;
border: 1px #e5e5e5 solid;
margin: 10px 0 20px 0;
text-indent: 10px;
font-size: 14px;
color: #333;
}
.content .list {
margin: 10px;
text: size 15px;
border: 1px solid #888;
}
.content .list li {
margin: 10px;
}
.content .list li button {
margin-left: 15px;
}