固定导航栏和可编辑表格
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
body, ul, li {
margin: 0;
padding: 0;
}
ul {
list-style: none;
}
a {
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
.container {
width: 600px;
margin: 20px auto;
background-color: #fbfbfb;
}
nav > div {
height: 90px;
display: flex;
justify-content: space-between;
align-items: center;
border: 1px solid #ccc;
padding: 0 20px;
}
nav > ul {
height: 30px;
width: 580px;
background-color: #fbfbfb;
border-bottom: 1px solid #888888;
display: flex;
justify-content: space-between;
align-items: center;
padding: 5px 10px;
}
main {
height: 100vh;
border: 1px solid #ccc;
}
main > table {
border: 1px solid #ccc;
margin: 15px auto;
background-color: #fff;
width: 95%;
}
main > table thead th:first-of-type {
width: 10%;
}
main > table thead th:first-of-type ~ * {
width: 45%;
}
main > table tbody input {
width: 95%;
margin: 0 1px;
}
main > table tr {
border: 1px solid #ccc;
background-color: aliceblue;
}
footer {
height: 60px;
line-height: 60px;
border: 1px solid #ccc;
text-align: center;
}
</style>
</head>
<body>
<header class="container">
<nav>
<div id="nav-top">
<div>Logo</div>
<div></div>
<div></div>
</div>
<ul id="nav-menu">
<li><a href="" title="">导航1</a></li>
<li><a href="" title="">导航2</a></li>
<li><a href="" title="">导航3</a></li>
<li><a href="" title="">导航4</a></li>
<li><a href="" title="">导航5</a></li>
</ul>
</nav>
</header>
<main class="container ">
<table>
<thead>
<tr>
<th>ID</th>
<th>name</th>
<th>value</th>
</tr>
</thead>
<tbody>
<tr>
<td>1</td>
<td>text name</td>
<td>text value</td>
</tr>
<tr>
<td>2</td>
<td>text name2</td>
<td>text value2</td>
</tr>
</tbody>
</table>
</main>
<footer class="container">
@copy 2021
</footer>
<script>
// 固定导航
var navTop = document.getElementById('nav-top'),
navMenu = document.getElementById('nav-menu');
window.onscroll = function () {
if (document.documentElement.scrollTop > navTop.offsetHeight + navTop.offsetTop) {
navMenu.style.cssText = 'position:fixed;top:0';
} else {
navMenu.style.cssText = 'position:static';
}
}
// 可编辑表格
// var tds = document.getElementsByTagName('table')[0].getElementsByTagName('td');
// [...tds].forEach(function (td){
// // ID字段不可编辑
// if (td !== [...td.parentNode.childNodes].filter(el => el.nodeType === 1).shift()) {
// td.onclick = function () {
// // 如果input框,直接获取焦点
// if ([...this.childNodes].some(el => el.nodeName === 'INPUT')) {
// [...this.childNodes].filter(el => el.nodeName === 'INPUT').shift().focus();
// } else {
// var eInput = document.createElement('input');
// eInput.type = 'text';
// eInput.value = this.textContent;
// this.innerHTML = '';
// this.appendChild(eInput);
// eInput.focus();
// // 失去焦点时
// eInput.onblur = function () {
// this.parentNode.innerHTML = this.value;
// }
// }
// }
// }
// });
var tBody = document.getElementsByTagName('table')[0].getElementsByTagName('tbody')[0];
tBody.addEventListener('click', function (ev) {
var event = ev || window.event;
event.stopPropagation ? event.stopPropagation() : (event.cancelBubble = true);
// ID字段不可编辑
if ([...event.target.parentNode.childNodes].filter(el => el.nodeType === 1).shift() !== event.target) {
// 如果input框,直接获取焦点
if ([...event.target.childNodes].some(el => el.nodeName === 'INPUT')) {
[...event.target.childNodes].filter(el => el.nodeName === 'INPUT').shift().focus();
} else {
var eInput = document.createElement('input');
eInput.type = 'text';
eInput.value = event.target.textContent;
event.target.innerHTML = '';
event.target.appendChild(eInput);
eInput.focus();
// 失去焦点时
eInput.onblur = function () {
this.parentNode.innerHTML = this.value;
}
}
}
});
</script>
</body>
</html>
- 页面滚动时,导航栏固定在顶部
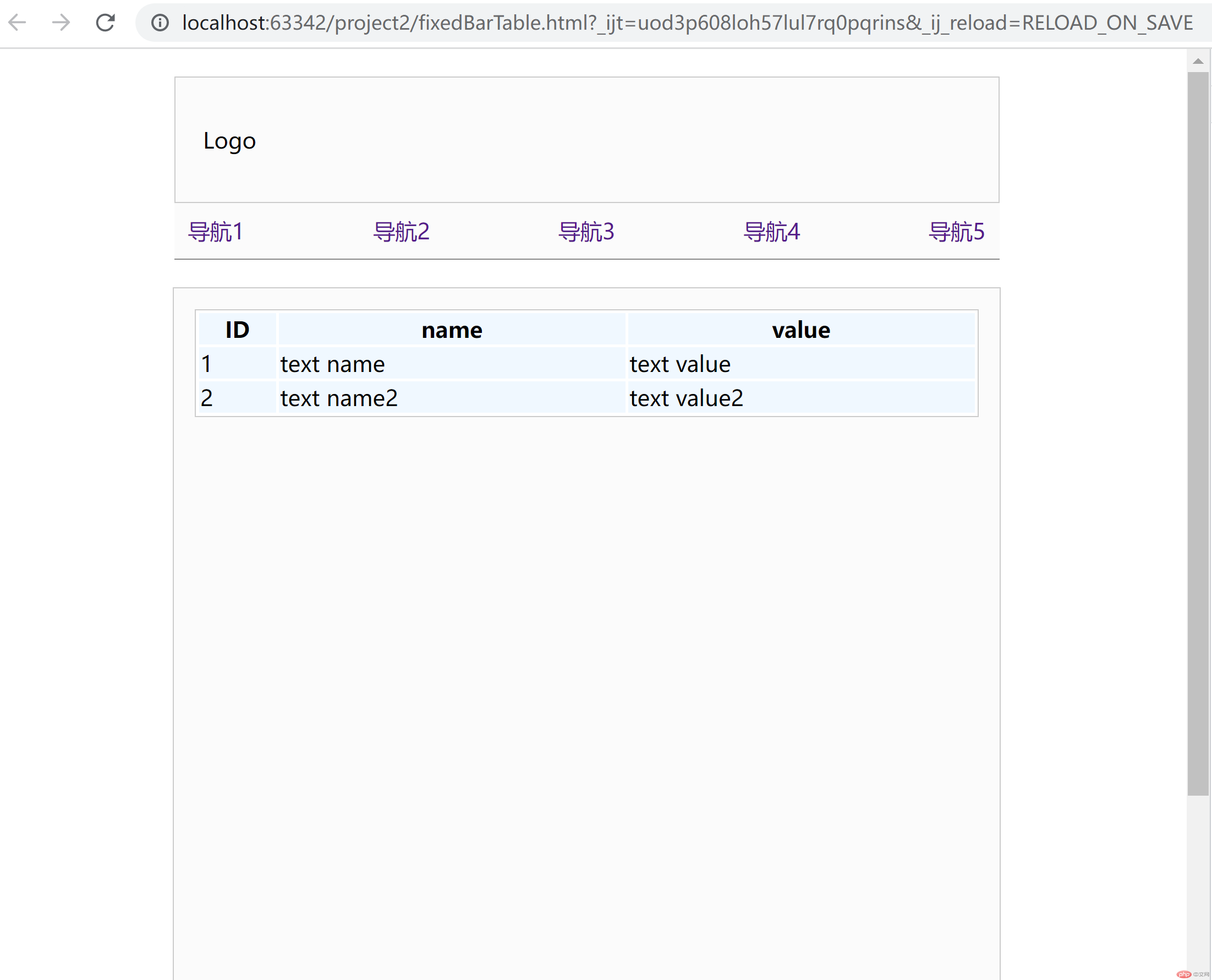
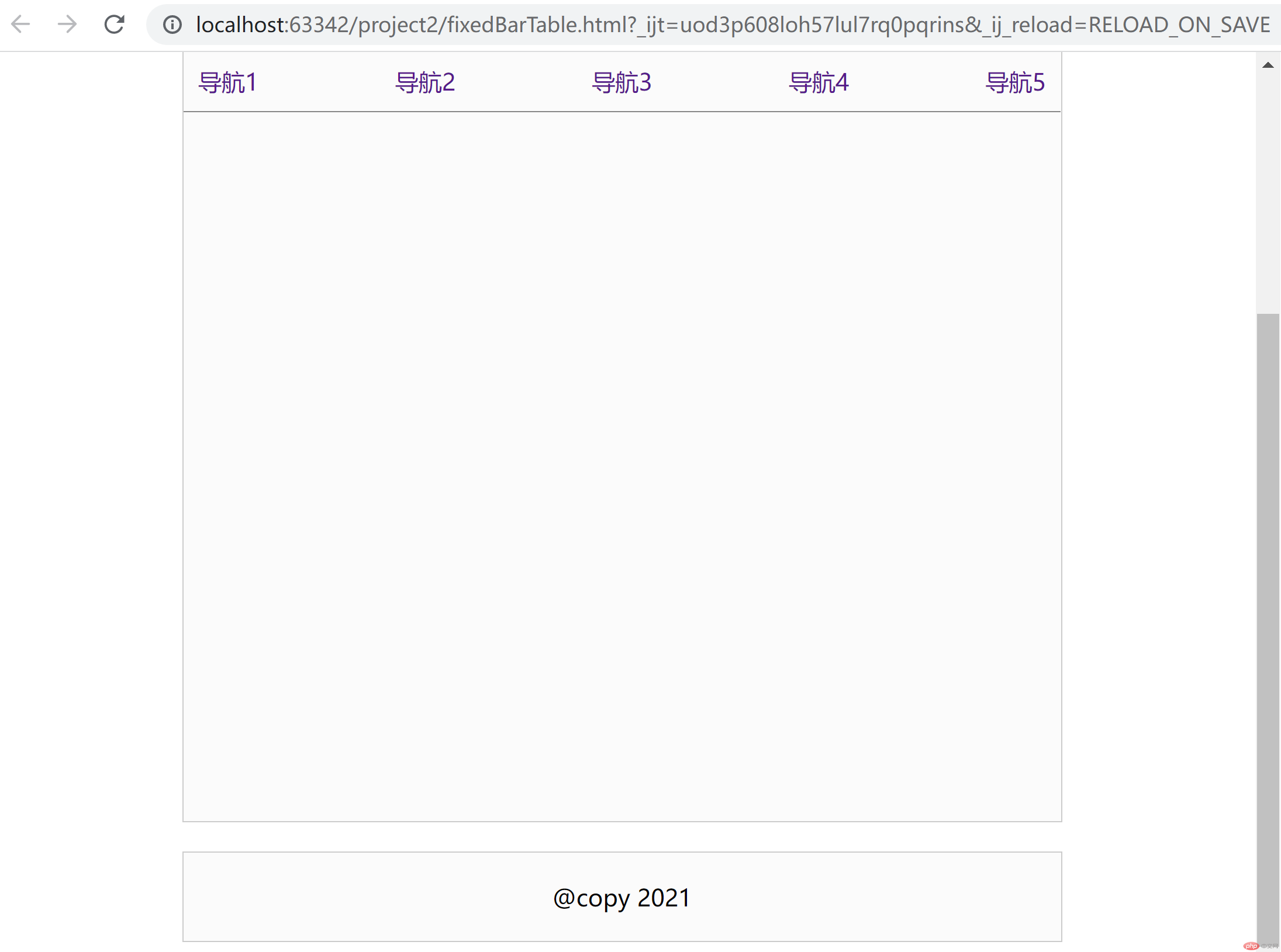
- 编辑第一张图中表格最后一个字段为 hello world! 失去焦点生效
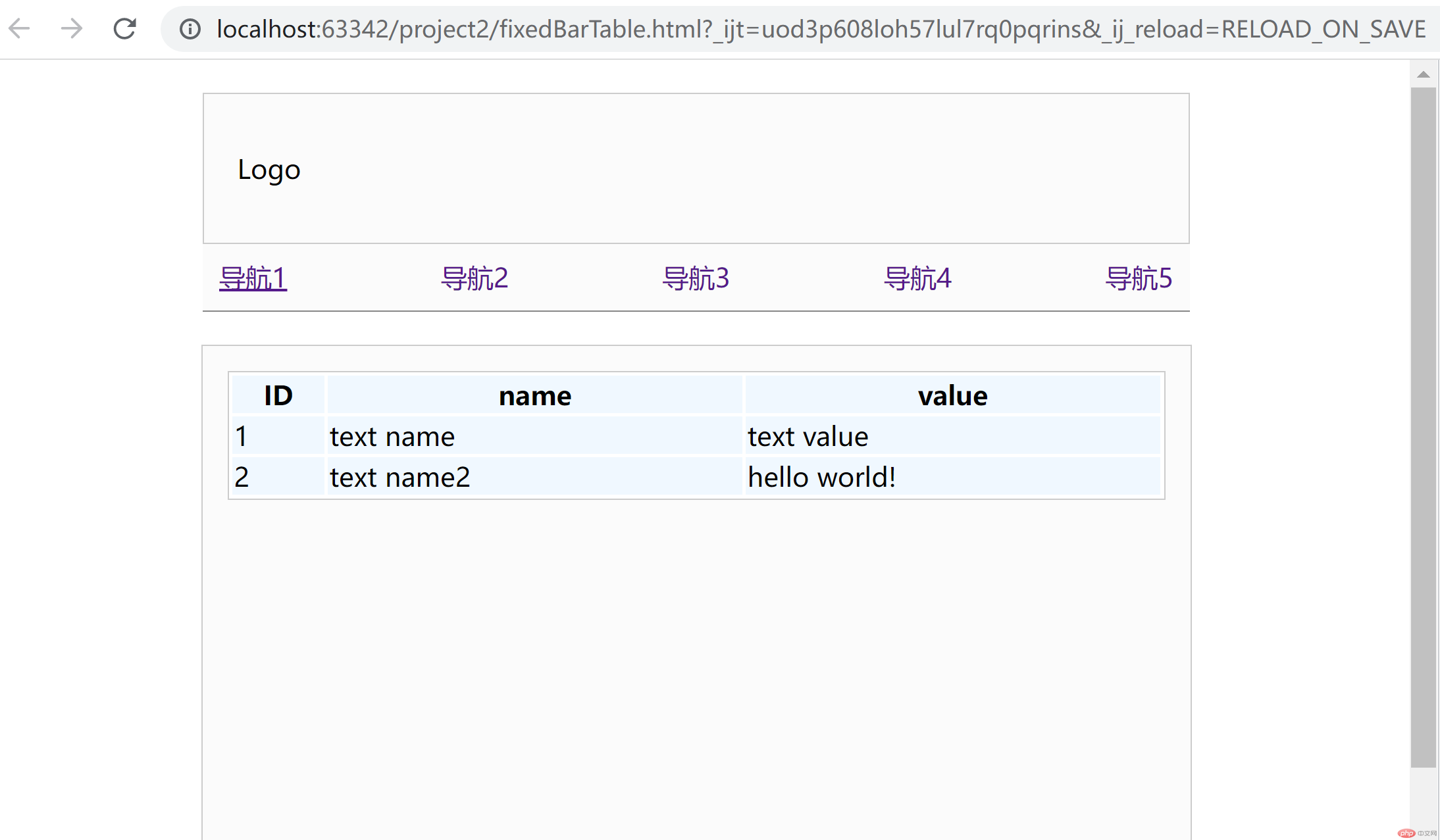