今天所学心得、笔记
1、jQuery实现留言本案例(todolist)
示例截图
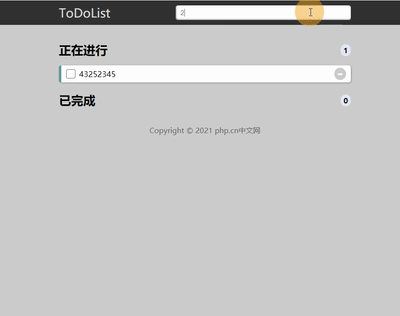
示例代码
<body>
<header>
<div class="header">
<label for="add">ToDoList</label>
<input type="text" id="add" placeholder="添加计划项目......" autofocus>
</div>
</header>
<main>
<div class="todo">
<div class="title">
<span class="titleText">正在进行</span>
<span class="titleTip">0</span>
</div>
<ul class="todoList">
<!-- <li>-->
<!-- <div class="left">-->
<!-- <input type="checkbox" name="">-->
<!-- <span>111111111111111</span>-->
<!-- </div>-->
<!-- <a href="#" class="right">-</a>-->
<!-- </li>-->
</ul>
</div>
<div class="done">
<div class="title">
<span class="titleText">已完成</span>
<span class="titleTip">0</span>
</div>
<ul class="doneList">
<!-- <li>-->
<!-- <div class="left">-->
<!-- <input type="checkbox" name="">-->
<!-- <span>111111111111111</span>-->
<!-- </div>-->
<!-- <a href="#" class="right">-</a>-->
<!-- </li>-->
</ul>
</div>
</main>
<footer>
<span>Copyright © 2021 php.cn中文网</span>
</footer>
<script src="jquery-3.5.1.js"></script>
<script>
// 启动时,读取缓存数据,并渲染到页面
loadToPage();
// 获取input框输入的数据
$("input#add").on("keydown", function (ev) {
let addText = $(this).val();
if(addText !== '') {
// console.log(ev.keyCode);
if(ev.keyCode == 13) {
// console.log(addText);
//获取本地存储的数据
let localData = getData();
localData.push({title: addText, done: false});
//存储入本地缓存
saveData(localData);
loadToPage();
$(this).val('');
}
}
});
//渲染数据,至页面
function loadToPage() {
// 总数,计数器
let todoCount = 0;
let doneCount = 0;
//渲染前,先清空页面数据
$(".todoList, .doneList").empty();
// 获取本地缓存数据
let localData = getData();
// console.log(localData);
// // 循环数据,渲染数据,至页面(原生写法)
// localData.forEach((item, index) => {
// // console.log(item);
// $(".todoList").prepend(`<li><div class='left'><input type='checkbox'><span>${item}</span></div><a href='#' class='right' id="${index}">-</a></li>`);
// });
// 循环数据,渲染数据,至页面(jQuery写法, 要注意:数据与index在括号中的位置,与原生相反)
$.each(localData, (index, item) => {
if(!item.done) {
$(".todoList").prepend(`<li><div class='left'><input type='checkbox' data-id="${index}"><span>${item.title}</span></div><a href='#' class='right' id="${index}">-</a></li>`);
todoCount++;
}else {
$(".doneList").prepend(`<li><div class='left'><input type='checkbox' data-id="${index}" checked><span>${item.title}</span></div><a href='#' class='right' id="${index}">-</a></li>`);
doneCount++;
}
})
$(".todo .titleTip").text(todoCount);
$(".done .titleTip").text(doneCount);
}
//存储列表数据,入本地缓存
function saveData(data) {
// 将列表数据,由对象格式转换成,字符串格式,存储到本地
localStorage.setItem('todoList', JSON.stringify(data));
}
//读取本地存储列表数据
function getData() {
let data = localStorage.getItem('todoList');
if(data) {
// 本地存储里面的数据,由字符串格式转换成,对象格式
return JSON.parse(data);
}else {
return [];
}
}
// 删除列表中的数据
$(".todoList, .doneList").on("click", "a", function () {
// 获取a标签id
let id = $(this).val("id").get(0).id;
// console.log(id);
// 获取本地缓存数据
let localData = getData();
// 删除数据
localData.splice(id, 1);
//存储数据,并接着重新渲染数据
saveData(localData);
// 重新渲染页面
loadToPage();
});
//进行中,已完成,分组设置(修改done的值),在loadToPage()函数中进行分组显示
$(".todoList, .doneList").on('click', 'input', function () {
// 获取本地缓存数据
let localData = getData();
// console.log(localData);
// 获取选中li的id
let id = $(this).data("id");
// console.log(id);
// 修改对应数据的,done值
localData[id].done = $(this).prop("checked");
// console.log(localData);
//存储数据,并接着重新渲染数据
saveData(localData);
// 重新渲染页面
loadToPage();
});
</script>
</body>
2、$.ajax实现,$.get,$.post,$.ajax实现jsonp跨域请求
示例截图
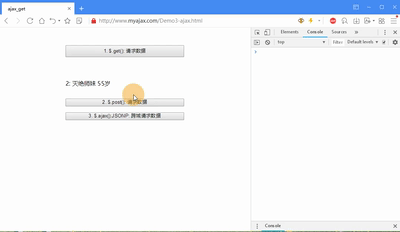
示例代码
<body>
<div>
<button class="get">1. $.get(): 请求数据</button>
<button class="post">2. $.post(): 请求数据</button>
<button class="jsonp">3. $.ajax():JSONP: 跨域请求数据</button>
</div>
<script src="jquery-3.5.1.js"></script>
<script>
// 1. $.get(请求url,查询参数,成功回调)
$('.get').click(function (ev) {
$.get('users.php?id=2', function (data) {
$(ev.target).after("<div></div>").next().html(data);
})
})
// 2. post()
$(".post").click(function (ev) {
$.post("users.php", { id: 3 }, function (data) {
console.log(data);
$(ev.target).after("<div></div>").next().html(data);
});
});
// 3. $.ajax实现jsonp跨域请求
$(".jsonp").click(function (ev) {
$.ajax({
type: "get",
url: "http://www.mycors.com/test.php?id=2&jsonp=?",
dataType: "jsonp",
// 告诉跨域访问的服务器需要返回的函数名称
// jsonpCallback: "show",
success: function (data) {
console.log(data);
$("button:last-of-type").after("<div>").next().html(`${data.name} [${data.email}}]`);
},
});
});
</script>
</body>