JS部分
const insertComment = function (ele,isBtn = false) {
if (event.key === 'Enter' || isBtn) {
// 1. 非空判断
if (ele.value.length === 0) {
// 提示用户
alert('留言不能为空');
// 重置焦点
ele.focus();
// 直接返回
return false;
}
const ul = document.querySelector('.container_body');
ele.value = `<div class="personal_body"><div class="comment_body">${ele.value}</div>`
ele.value += `<div class="submit_del"><button onclick="del(this.parentNode)">删除评论</button></div>`;
ul.insertAdjacentHTML(`afterend`, `${ele.value}<div class="zdhf">自动回复内容</div><br><hr>`);
// 3. 清空输入框
ele.value = null;
}
};
submit_btn.onclick = (e)=>{
const text = document.getElementById("pl")
insertComment(text,true);
}
// 删除
const del = function (ele) {
console.log(ele)
return confirm('是否删除?') ? (ele.parentNode.outerHTML = null) : false;
};
CSS部分
*{
margin: 0;
padding: 0;
}
.container{
display: grid;
place-content: center;
}
.main{
display: grid;
}
.comment,.submit,.comment_body{
background-color: lightskyblue;
padding: 5px;
}
.comment{
width: 400px;
height: 200px;
border-radius: 30px 30px 0 0;
display: grid;
}
.comment textarea{
width: 380px;
height:190px;
border: none;
border-radius: 10px;
display: grid;
place-self: center center;
}
.submit{
width: 400px;
border-radius: 0 0 30px 30px;
display: grid;
}
.submit button{
background-color: pink;
border: none;
border-radius: 30px;
padding: 10px;
display: grid;
place-self: center center;
}
input{
border: none;
border-radius: 10px;
}
/*接收评论区部分*/
.container_body{
width: 400px;
margin-top: 30px;
display: grid;
place-content: center;
}
.personal_body{
display: grid;
place-self: center center;
}
.comment_body{
width: 400px;
min-height: 50px;
border-radius: 30px;
display: grid;
place-self: center center;
text-align: center;
}
.submit_del{
width: 400px;
border-radius: 0 0 30px 30px;
display: grid;
place-self: center center;
text-align: center;
}
.submit_del button{
border: none;
background-color: pink;
border-radius: 30px;
padding: 10px;
}
.zdhf{
width: 400px;
min-height: 50px;
background-color: lightgreen;
border-radius: 30px;
margin-top: 5px;
display: grid;
place-self: center center;
text-align: center;
}
HTML部分
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>实战评论功能</title>
</head>
<link rel="stylesheet" href="work.css">
<body>
<section class="container">
<h2>评论区</h2>
<div class="main">
<div class="comment">
<textarea name="pl" id="pl" cols="30" rows="10" placeholder="请输入你的评论内容" onkeydown="insertComment(this)"></textarea>
</div>
<div class="submit">
<button type="submit" id="submit_btn">提交评论/或按回车提交</button>
</div>
</div>
</section>
<section class="container_body">
</section>
</body>
<script src="work.js"></script>
</html>
效果图
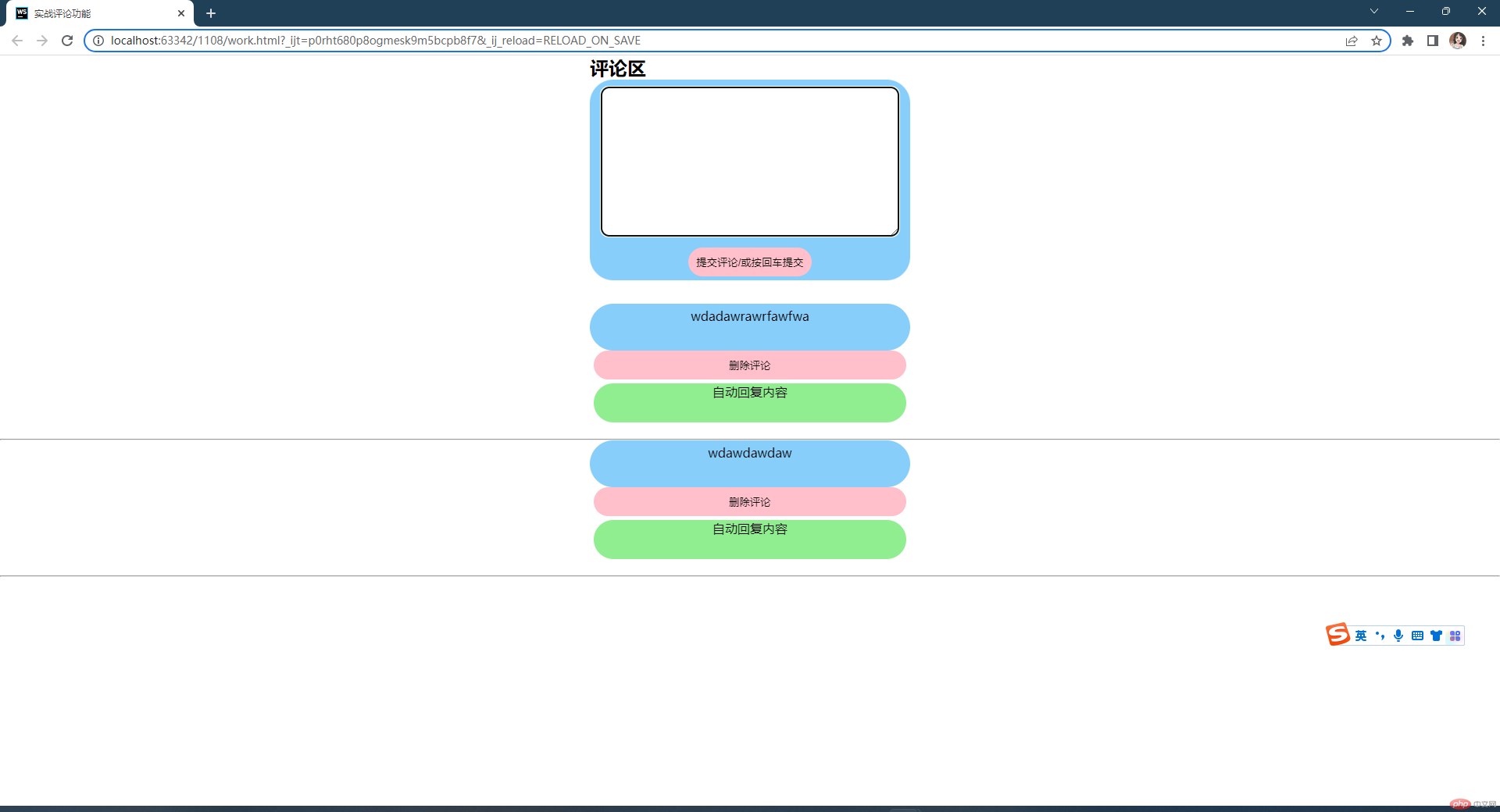